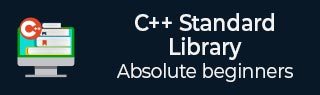
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::sqrt() Function
The C++ Valarray::sqrt() function is used to determine the square root of each element's value in the valarray. The sqrt() function of the cmath’s is called once for each element by this function. It accepts a non-negative number as an input and outputs the square root of the entered value.
In C++, the function sqrt() is only defined for positive integers. This indicates that the sqrt() function cannot be used with negative integers. Negative numbers will provide '-nan' if they are supplied to sqrt in C++ as parameters.
A C++ error will be thrown indicating that "no matching function for call to sqrt()" would be generated if the sqrt() function is used without any parameter.
Syntax
Following is the syntax for C++ Valarray::sqrt() Function −
sqrt(const valarray<Type>& x);
Parameters
x − It contains elements of a type for which the unary function sqrt is defined.
Examples
Example 1
Let's consider the following example, where we are going to use sqrt() function and retrieving output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> tutorial = { 100,125,121,169,143}; valarray<double> valarray1; valarray1 = sqrt(tutorial); cout << "The sqrt()" << " Valarray is : " << endl; for (double& x : valarray1) { cout << x << " "; } cout << endl; return 0; }
Output
When we compile and run the above program, this will produce the following result −
The sqrt() Valarray is : 10 11.1803 11 13 11.9583
Example 2
Consider the following example, where we are going to pass negative number to the sqrt() function and retrieving the output of both original and sqrt() valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarray = {4,25,-0.5,36,81,-1}; cout << "The Orignal Valarray is : "; for (double& ele : myvalarray) cout << ele << " "; valarray<double> sqrtValArray; sqrtValArray = sqrt(myvalarray); cout << "\nThe sqrt Valarray is : "; for (double& ele : sqrtValArray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray is : 4 25 -0.5 36 81 -1 The sqrt() Valarray is : 2 5 -nan 6 9 -nan
Example 3
In the following example, we are going to use the integral type with the sqrt() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { int a = 16; double Result = sqrt(a); cout << "Result = " << Result; return 0; }
Output
On running the above program, it will produce the following result −
Result = 4
Example 4
Following is the example where the compiler will throw an error of using the sqrt() function without any parameter.
#include <iostream> #include <valarray> using namespace std; int main() { int a = 25; double Result = sqrt(); cout << "Result = " << Result; return 0; }
Output
When we compile and run the above program, this will produce the following result −
main.cpp:7:25: error: no matching function for call to 'sqrt()'
To Continue Learning Please Login