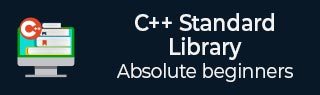
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::sinh() Function
The C++ Valarray::sinh() function calculates the hyperbolic sine of the value of each element in a valarray and returns a valarray that contains the hyperbolic sine of every element.
The hyperbolic sine function, often known as Sinh, is the trigonometric equivalent of the Sin circular function. It is simple to define the hyperbolic sine function as half the difference of two exponential functions at the locations x and -x. Every element in the valarray receives a single call to cmath's sinh() function.
Syntax
Following is the syntax for C++ Valarray::sinh() Function −
valarray<Type> sinh(const valarray<Type>& x);
Parameters
x − It contains elements of a type for which the unary function sinh is defined.
Examples
Example 1
Consider the following example, where we are going to use sinh() function and retrieving output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> valarray0 = {1,0.23,0.5,-0.6}; valarray<double> valarray1; valarray1 = sinh(valarray0); cout << "The New sinh" << " Valarray is : " << endl; for (double& x : valarray1) { cout << x << " "; } cout << endl; return 0; }
Output
When we compile and run the above program, this will produce the following result −
The New sinh Valarray is : 1.1752 0.232033 0.521095 -0.636654
Example 2
In the following example,we are going to use sinh() function and getting output of both original valarray and sinh valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarr = { 0.2,0.4,-1.2,3,4}; cout << "The Orignal Valarray is : "; for (double& ele : myvalarr) cout << ele << " "; valarray<double> sinhvalarray = sinh(myvalarr); cout << "\nThe sinh Valarray is : "; for (double& ele : sinhvalarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray is : 0.2 0.4 -1.2 3 4 The sinh Valarray is : 0.201336 0.410752 -1.50946 10.0179 27.2899
Example 3
Following is the example, where we are going to use the integral type with the sinh() function and retrieve the output.
#include <iostream> #include <cmath> using namespace std; int main() { int a = 4; double Result; Result = sinh(a); cout << "sinh(a) = " << Result << endl; return 0; }
Output
On running the above program, it will produce the following result −
sinh(a) = 27.2899
Example 4
Considering the following example, where we are going to use degree=30 with the sinh() function and checking how it works.
#include <iostream> #include <cmath> using namespace std; int main() { double a = 2.3, result; result = sinh(a); cout << "sinh(a) = " << result << endl; double yDegrees = 30; a = yDegrees * 3.14159/180; result = sinh(a); cout << "sinh(a) applied with degree = " << result << endl; return 0; }
Output
When the above program gets executed, it will produce the following result −
sinh(a) = 4.93696 sinh(a) applied with degree = 0.547853
To Continue Learning Please Login