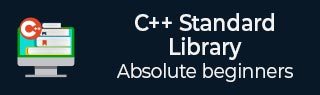
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::exp Function
The class used to represent and work with value arrays is called std::valarray. It provides a variety of generalized subscript operators, slicing, and indirect access in addition to element-wise mathematical operations.
The C++ Valarray::exp() function is used to compute e raised to the power of the value of the element in the valarray. Each element receives a single call to this method, which overloads with cmath's exp() function.
Syntax
Following is the syntax for C++ Valarray::exp Function −
exp (const valarray<T>& x);
Parameters
x − It is containing elements of a type for which the unary function exp is defined.
Examples
Example 1
Let's look into the following example, where we are going to use exp() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> varr = { 4,6,8,10,11 }; valarray<double> varr1; varr1 = exp(varr); cout << "The New exp" << " Valarray is : " << endl; for (double& x : varr1) { cout << x << " "; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The New exp Valarray is : 54.5982 403.429 2980.96 22026.5 59874.1
Example 2
Let's consider the following example, where we are going to use exp() function and retrieving the output with both original valarray and exp valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarray = { 1.2, -0.46, -1.5, 0.45, -1.23 }; cout << "The Orignal Valarray is : "; for (double& ele : myvalarray) cout << ele << " "; valarray<double> expValarray; expValarray = exp(myvalarray); cout << "\nThe exp Valarray is : "; for (double& ele : expValarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray is : 1.2 -0.46 -1.5 0.45 -1.23 The exp Valarray is : 3.32012 0.631284 0.22313 1.56831 0.292293
Example 3
In the following example, we are going to consider the basic example of the usage of the exp() function.
#include <stdio.h> #include <math.h> int main () { double a, result; a = 2.3; result = exp (a); printf ("The exp value of %f is %f.\n", a, result ); return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The exp value of 2.300000 is 9.974182.
To Continue Learning Please Login