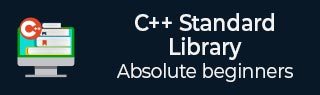
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::cosh Function
The C++ Valarray::cosh() function generates a valarray that contains the hyperbolic cosines of all the elements and is used to compute the hyperbolic cosine of each element's value. The cosh() method in cmath’s is overloaded by this function, which calls it once for each element.
Syntax
Following is the syntax for C++ Valarray::cosh Function −
cosh (const valarray<T>& x);
Parameters
x − It is containing elements of a type for which the unary function cosh is defined.
Examples
Example 1
Let's look into the following example, where we are going to use cosh() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> valarray0 = { 0.15, 0.3, 0.65, 0.89, 0.4 }; valarray<double> valarray1; valarray1 = cosh(valarray0); cout << "The New cosh" << " Valarray is : " << endl; for (double& x : valarray1) { cout << x << " "; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The New cosh Valarray is : 1.01127 1.04534 1.21879 1.42289 1.08107
Example 2
Let's consider the following example, where we are going to use cosh() function and performing a arithmetic operation and retrieving the output.
#include <cmath> #include <iomanip> #include <iostream> #include <valarray> void show(const char* title, const std::valarray<float>& data) { const int w { 8 }; std::cout << std::setw(w) << title << " | "; for (float a : data) std::cout << std::setw(w) << a << " | "; std::cout << '\n'; } int main() { const std::valarray<float> a { .23, .25, .36, .45 }; const auto sinh = std::sinh(a); const auto cosh = std::cosh(a); const auto Multiply = (cosh * sinh); show("sinh(a)", sinh); show("cosh(a)", cosh); show("Result(cosh * sinh)", Multiply ); }
Output
Let us compile and run the above program, this will produce the following result −
sinh(a) | 0.232033 | 0.252612 | 0.367827 | 0.465342 | cosh(a) | 1.02657 | 1.03141 | 1.0655 | 1.10297 | Result(cosh * sinh) | 0.238198 | 0.260548 | 0.39192 | 0.513258 |
Example 3
In the following example, we are going to use cos() function and retrieving the output of both original valarray and cos() valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarr = { 0.2, -0.3, -0.4, 0.5, -0.1 }; cout << "The Orignal Valarray is : "; for (double& ele : myvalarr) cout << ele << " "; valarray<double> coshvalarray = cosh(myvalarr); cout << "\nThe cosh Valarray is : "; for (double& ele : coshvalarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray is : 0.2 -0.3 -0.4 0.5 -0.1 The cosh Valarray is : 1.02007 1.04534 1.08107 1.12763 1.005
To Continue Learning Please Login