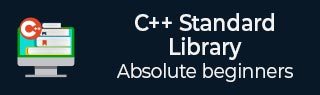
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::cos Function
The C++ Valarray::cos() function calculates the cosine of each element's value in a valarray and returns a valarray that contains the cosines of all the elements. Each element receives a single call to this method, which overloads the cmath’s cos() function.
Syntax
Following is the syntax for C++ Valarray::cos Function −
cos (const valarray<T>& x);
Parameters
x − It is containing elements of a type for which the unary function cos is defined.
Examples
Example 1
Let's look into the following example, where we are going to use cos() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> varr = { 0.1, 0.2, 0.3, 0.4, 0.5 }; valarray<double> varr1; varr1 = cos(varr); cout << "The New cos " << " Valarray is : " << endl; for (double& x : varr1) { cout << x << " "; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The New cos Valarray is : 0.995004 0.980067 0.955336 0.921061 0.877583
Example 2
Let's consider the following example, where we are going to use cos() function and retrieving the output along with multiplication result value of the cos().
#include <cmath> #include <iomanip> #include <iostream> #include <valarray> void show(const char* title, const std::valarray<float>& data) { const int w { 8}; std::cout << std::setw(w) << title << " | "; for (float a : data) std::cout << std::setw(w) << a << " | "; std::cout << '\n'; } int main() { const std::valarray<float> a { .1, .2, .3, .4 }; const auto cos = std::cos(a); const auto Multiply = (cos * cos); show("a", a); show("cos(a)", cos); show("Multiply(cos * cos)", Multiply ); }
Output
Let us compile and run the above program, this will produce the following result −
a | 0.1 | 0.2 | 0.3 | 0.4 | cos(a) | 0.995004 | 0.980067 | 0.955337 | 0.921061 | Multiply(cos * cos) | 0.990033 | 0.960531 | 0.912668 | 0.848353 |
Example 3
In the following example, we are going to use cos() function and retrieving the output of both original valarray and cos() valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarray = { 0.6, -0.5, -0.4, -1 }; cout << "The Orignal Valarray is : "; for (double& ele : myvalarray) cout << ele << " "; valarray<double> cosvalarray = cos(myvalarray); cout << "\nThe cos Valarray is: "; for (double& ele : cosvalarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray is : 0.6 -0.5 -0.4 -1 The cos Valarray is: 0.825336 0.877583 0.921061 0.540302
Example 4
Following is the another example, where we are going to use cos() with integral type and retrieving the output.
#include <iostream> #include <cmath> using namespace std; int main() { int a = 142; double result; result = cos(a); cout << "cos(a) = " << result << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
cos(a) = -0.80901
To Continue Learning Please Login