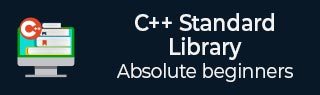
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::atan2 Function
The C++ Valarray::atan2() function calculates the inverse tangent of the (y/x) value of each element in the valarray and returns a valarray containing the inverse tangent of all the items. where y is the proportion of the y-coordinate and x is the proportion of the x-coordinate.
The atan2() function of cmath is overload with in this function, which calls it once for each element. It uses the sings of the arguments to determine the appropriate quadrant.
- If both the y,x are of same valarray size,the function computes the inverse of the each pair of corresponding values from y and x.
- If y is valarray and x is value, the function computes the inverse tangent of each element of y paired with x.
- If x is valarray and y is value, the function computes the inverse tangent of y paired with each element of x.
Syntax
Following is the syntax for C++ Valarray::atan2 Function −
atan2 (const valarray<T>& y, const valarray<T>& x); atan2 (const valarray<T>& y, const T& x); atan2 (const T& y, const valarray<T>& x);
Parameters
- x − It is containing elements of a type for which the unary function abs is defined.
- y − It is a valarray element with the y coordinate(s).
Examples
Example 1
Let's look into the following example, where we are going to use atan2() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { double y[] = { 0.4, 1.2, -1.0 }; double x[] = { 0.5, 1.3, -1.0 }; valarray<double> ycoords(y, 2); valarray<double> xcoords(x, 2); valarray<double> result = atan2(ycoords, xcoords); cout << "Result:"; for (size_t i = 0; i < result.size(); ++i) cout << ' ' << result[i]; cout << '\n'; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
Result: 0.674741 0.745419
Example 2
Considering the another scenario, where we are going to use the atan2() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main (){ valarray<double> y = {4, 10, 9}; valarray<double> x = {12, 22, 14}; valarray<double> result1 = atan2(y, x); cout<<"\natan2(y, x) returns: "; for(int i = 0; i < result1.size(); i++) cout<<result1[i]<<" "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
atan2(y, x) Return: 0.321751 0.426627 0.571337
Example 3
In the following example, we are going to use atan2() function and retrieving the output in radians.
#include <iostream> #include <cmath> #define PI 3.141592654 using namespace std; int main() { double result; int x = -12; float y = 31.6; result = atan2(y, x); cout << "atan2(y/x) = " << result << " radians" << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
atan2(y/x) = 1.93372 radians
Example 4
Following is the example, where we are going to use atan2() function and retrieving the output in degrees.
#include <iostream> #include <cmath> #define PI 3.141592654 using namespace std; int main() { double result; int x = -12; float y = 31.6; result = atan2(y, x); cout << "atan2(y/x) = " << result * (180 / PI) << " degrees"; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
atan2(y/x) = 110.794 degrees
To Continue Learning Please Login