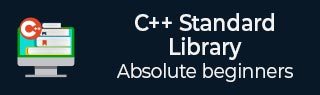
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::asin Function
The C++ Valarray::asin()function generates a valarray holding the values of all the elements and computes the arc sine of each element's value.
The C++ asin() function returns the inverse sine of a radian-based integer. The asin() function of cmath is overloaded, and it is used once for each element.
Syntax
Following is the syntax for C++ Valarray::asin Function −
asin (const valarray<T>& x);
Parameters
x − It is containing elements of a type for which the unary function asin is defined.
Examples
Example 1
Let's look into the following example, where we are going to use the asin() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> varr = { 1.6, -0.5, 0, -1 }; valarray<double> varr1; varr1 = asin(varr); cout << "The asin Valarray " << "Values : " << endl; for (double& x : varr1) { cout << x << " "; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The asin Valarray Values : nan -0.523599 0 -1.5708
Example 2
Following is the another example, where we are going to use the asin() function and retrieving the output with comparison of original and asin Valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<int> myvalarr = { 10, 22, 33, 46, 28 }; cout << "The Orignal Valarray : "; for (int& ele : myvalarr) cout << ele << " "; valarray<int> asinvalarray = asin(myvalarr); cout << "\nThe asin Valarray : "; for (int& ele : asinvalarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray : 10 22 33 46 28 The asin Valarray : -2147483648 -2147483648 -2147483648 -2147483648 -2147483648
Example 3
Considering the another scenario, where we are going to use the asin() function with integral type and retrieving the output in radians, degrees.
#include <iostream> #include <cmath> #define PI 3.141592654 using namespace std; int main() { int x = 1.6; double result; result = asin(x); cout << "asin(x) = " << result << " radians" << endl; cout << "asin(x1) = " << result*180/PI << " degrees"; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
asin(x) = 1.5708 radians asin(x1) = 90 degrees
To Continue Learning Please Login