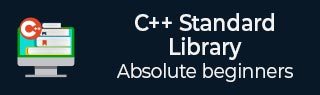
- The C Standard Library
- The C Standard Library
- The C++ Standard Library
- C++ Library - Home
- C++ Library - <fstream>
- C++ Library - <iomanip>
- C++ Library - <ios>
- C++ Library - <iosfwd>
- C++ Library - <iostream>
- C++ Library - <istream>
- C++ Library - <ostream>
- C++ Library - <sstream>
- C++ Library - <streambuf>
- C++ Library - <atomic>
- C++ Library - <complex>
- C++ Library - <exception>
- C++ Library - <functional>
- C++ Library - <limits>
- C++ Library - <locale>
- C++ Library - <memory>
- C++ Library - <new>
- C++ Library - <numeric>
- C++ Library - <regex>
- C++ Library - <stdexcept>
- C++ Library - <string>
- C++ Library - <thread>
- C++ Library - <tuple>
- C++ Library - <typeinfo>
- C++ Library - <utility>
- C++ Library - <valarray>
- The C++ STL Library
- C++ Library - <array>
- C++ Library - <bitset>
- C++ Library - <deque>
- C++ Library - <forward_list>
- C++ Library - <list>
- C++ Library - <map>
- C++ Library - <queue>
- C++ Library - <set>
- C++ Library - <stack>
- C++ Library - <unordered_map>
- C++ Library - <unordered_set>
- C++ Library - <vector>
- C++ Library - <algorithm>
- C++ Library - <iterator>
- C++ Programming Resources
- C++ Programming Tutorial
- C++ Useful Resources
- C++ Discussion
C++ Valarray::abs Function
The C++ Valarray::abs()function is used to determine the absolute value of each element in the valarray and returns a valarray that contains the absolute values of all the elements. The magnitude value of a number is its absolute value.
This function is overloaded for integral types in the <cstdlib> abs() function, floating-point types in the <cmath> abs() function, and complex values in the <complex> abs() function.
Syntax
Following is the syntax for C++ Valarray::abs Function −
abs (const valarray<T>& x);
Parameters
x − It is containing elements of a type for which the unary function abs is defined.
Examples
Example 1
Let's look into the following example, where we are going to use the abs() function and retrieving the output.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<int> varr = { 1, 2, -3, 4, -5 }; valarray<int> valarray1; valarray1 = abs(varr); cout << "The New Valarray "<< "value : "; for (int& A : valarray1) { cout << A << " "; } cout << endl; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The New Valarray value : 1 2 3 4 5
Example 2
In the following example, we are going to use the abs() function and retrieving the output with comparison of original and manipulated Valarray.
#include <iostream> #include <valarray> using namespace std; int main() { valarray<double> myvalarr = { 10, -12, -13, -5, 9.5 }; cout << "The Orignal Valarray Is : "; for (double& ele : myvalarr) cout << ele << " "; valarray<double> absValarray; absValarray = abs(myvalarr); cout << "\nThe Manipulated Valarray Is : "; for (double& ele : absValarray) cout << ele << " "; return 0; }
Output
Let us compile and run the above program, this will produce the following result −
The Orignal Valarray Is : 10 -12 -13 -5 9.5 The Manipulated Valarray Is : 10 12 13 5 9.5
Example 3
Considering the another scenario, where we are going to check how abs() function work.
#include <iostream> #include <cstdlib> using namespace std; int main() { cout << abs(-143); return 0; }
Output
Let us compile and run the above program, this will produce the following result −
143
To Continue Learning Please Login