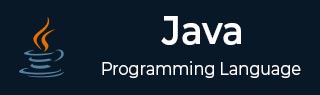
Java Tutorial
- Java - Home
- Java - Overview
- Java - History
- Java - Features
- Java vs C++
- Java Virtual Machine(JVM)
- Java - JDK vs JRE vs JVM
- Java - Hello World Program
- Java - Environment Setup
- Java - Basic Syntax
- Java - Variable Types
- Java - Data Types
- Java - Type Casting
- Java - Unicode System
- Java - Basic Operators
- Java - Comments
- Java - User Input
Java Control Statements
- Java - Loop Control
- Java - Decision Making
- Java - If-else
- Java - Switch
- Java - For Loops
- Java - For-Each Loops
- Java - While Loops
- Java - do-while Loops
- Java - Break
- Java - Continue
Object Oriented Programming
- Java - OOPs Concepts
- Java - Object & Classes
- Java - Class Attributes
- Java - Class Methods
- Java - Methods
- Java - Variables Scope
- Java - Constructors
- Java - Access Modifiers
- Java - Inheritance
- Java - Aggregation
- Java - Polymorphism
- Java - Overriding
- Java - Method Overloading
- Java - Dynamic Binding
- Java - Static Binding
- Java - Instance Initializer Block
- Java - Abstraction
- Java - Encapsulation
- Java - Interfaces
- Java - Packages
- Java - Inner Classes
- Java - Static Class
- Java - Anonymous Class
- Java - Singleton Class
- Java - Wrapper Classes
- Java - Enums
- Java - Enum Constructor
- Java - Enum Strings
Java Built-in Classes
Java File Handling
- Java - Files
- Java - Create a File
- Java - Write to File
- Java - Read Files
- Java - Delete Files
- Java - Directories
- Java - I/O Streams
Java Error & Exceptions
- Java - Exceptions
- Java - try-catch Block
- Java - try-with-resources
- Java - Multi-catch Block
- Java - Nested try Block
- Java - Finally Block
- Java - throw Exception
- Java - Exception Propagation
- Java - Built-in Exceptions
- Java - Custom Exception
Java Multithreading
- Java - Multithreading
- Java - Thread Life Cycle
- Java - Creating a Thread
- Java - Starting a Thread
- Java - Joining Threads
- Java - Naming Thread
- Java - Thread Scheduler
- Java - Thread Pools
- Java - Main Thread
- Java - Thread Priority
- Java - Daemon Threads
- Java - Thread Group
- Java - Shutdown Hook
Java Synchronization
- Java - Synchronization
- Java - Block Synchronization
- Java - Static Synchronization
- Java - Inter-thread Communication
- Java - Thread Deadlock
- Java - Interrupting a Thread
- Java - Thread Control
- Java - Reentrant Monitor
Java Networking
- Java - Networking
- Java - Socket Programming
- Java - URL Processing
- Java - URL Class
- Java - URLConnection Class
- Java - HttpURLConnection Class
- Java - Socket Class
- Java - Generics
Java Collections
Java List Interface
Java Queue Interface
Java Map Interface
- Java - Map Interface
- Java - HashMap
- Java - LinkedHashMap
- Java - WeakHashMap
- Java - EnumMap
- Java - SortedMap Interface
- Java - TreeMap
- Java - The IdentityHashMap Class
Java Set Interface
- Java - Set Interface
- Java - HashSet
- Java - EnumSet
- Java - LinkedHashSet
- Java - SortedSet Interface
- Java - TreeSet
Java Data Structures
- Java - Data Structures
- Java - Enumeration
- Java - BitSet Class
- Java - Dictionary
- Java - Hashtable
- Java - Properties
Java Collections Algorithms
Advanced Java
- Java - Command-Line Arguments
- Java - Lambda Expressions
- Java - Sending Email
- Java - Applet Basics
- Java - Javadoc Comments
- Java - Autoboxing and Unboxing
- Java - File Mismatch Method
- Java - REPL (JShell)
- Java - Multi-Release Jar Files
- Java - Private Interface Methods
- Java - Inner Class Diamond Operator
- Java - Multiresolution Image API
- Java - Collection Factory Methods
- Java - Module System
- Java - Nashorn JavaScript
- Java - Optional Class
- Java - Method References
- Java - Functional Interfaces
- Java - Default Methods
- Java - Base64 Encode Decode
- Java - Switch Expressions
- Java - Teeing Collectors
- Java - Microbenchmark
- Java - Text Blocks
- Java - Null Pointer Exception
- Java - Packaging Tools
- Java - Sealed Classes
- Java - Record Classes
- Java - Hidden Classes
- Java - Compact Number Formatting
Java Miscellaneous
- Java - Recursion
- Java - Regular Expressions
- Java - Serialization
- Java - Strings
- Java - Process API Improvements
- Java - Stream API Improvements
- Java - Enhanced @Deprecated Annotation
- Java - CompletableFuture API Improvements
- Java - Array Methods
- Java - Streams
- Java - Datetime Api
- Java 8 - New Features
- Java 9 - New Features
Java APIs & Frameworks
Java Useful Resources
Java - Boolean class
Java Boolean Class
The Java Boolean class wraps a value of the primitive type boolean in an object. An object of type Boolean contains a single field whose type is boolean.
Boolean Class Declaration in Java
Following is the declaration for java.lang.Boolean class −
public final class Boolean extends Object implements Serializable, Comparable<Boolean>
Boolean Class Fields
Following are the fields for java.lang.Boolean class −
static Boolean FALSE − This is the Boolean object corresponding to the primitive value false.
static Boolean TRUE − This is the Boolean object corresponding to the primitive value true.
static Class<Boolean> TYPE − This is the Class object representing the primitive type boolean.
Boolean Class Constructors
Sr.No. | Constructor & Description |
---|---|
1 |
Boolean(boolean value) This allocates a Boolean object representing the value argument. |
2 |
Boolean(String s) This allocates a Boolean object representing the value true if the string argument is not null and is equal, ignoring case, to the string "true". |
Boolean Class Methods
Sr.No. | Method & Description |
---|---|
1 | boolean booleanValue()
This method returns the value of this Boolean object as a boolean primitive. |
2 | int compareTo(Boolean b)
This method compares this Boolean instance with another. |
3 | boolean equals(Object obj)
This method returns true if and only if the argument is not null and is a Boolean object that represents the same boolean value as this object. |
4 | static boolean getBoolean(String name)
This method returns true if and only if the system property named by the argument exists and is equal to the string "true". |
5 | int hashCode()
This method returns a hash code for this Boolean object. |
6 | int hashCode(boolean value)
This method returns a hash code for a given boolean value. It is compatible with Boolean.hashCode(). |
7 | static boolean logicalAnd​(boolean a, boolean b)
This method returns the result of applying the logical AND operator to the specified boolean operands. |
8 | static boolean logicalOr(boolean a, boolean b)
This method returns the result of applying the logical OR operator to the specified boolean operands. |
9 | static boolean logicalXor(boolean a, boolean b)
This method returns the result of applying the logical XOR operator to the specified boolean operands. |
10 | static boolean parseBoolean(String s)
This method parses the string argument as a boolean. |
11 | String toString()
This method returns a String object representing this Boolean's value. |
12 | static String toString(boolean b)
This method returns a String object representing the specified boolean. |
13 | static Boolean valueOf(boolean b)
This method returns a Boolean instance representing the specified boolean value. |
14 | static Boolean valueOf(String s)
This method returns a Boolean with a value represented by the specified string. |
Methods Inherited
This class inherits methods from the following classes −
- java.lang.Object
Example of Java Boolean Class
The following example shows the usage of some important methods provided by Boolean class.
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 2 Boolean objects b1, b2 Boolean b1, b2; // assign values to b1, b2 b1 = Boolean.valueOf(true); b2 = Boolean.valueOf(false); // create an int res int res; // compare b1 with b2 res = b1.compareTo(b2); String str1 = "Both values are equal "; String str2 = "Object value is true"; String str3 = "Argument value is true"; if( res == 0 ) { System.out.println( str1 ); } else if( res > 0 ) { System.out.println( str2 ); } else if( res < 0 ) { System.out.println( str3 ); } } }
Output
Let us compile and run the above program, this will produce the following result −
Object value is true
To Continue Learning Please Login