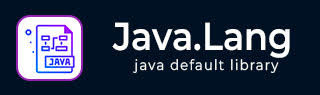
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Boolean toString(boolean) method
Description
The Java Boolean toString(boolean) returns a String object representing the specified boolean. If the specified boolean is true, then the string "true" will be returned, otherwise the string "false" will be returned.
Declaration
Following is the declaration for java.lang.Boolean.toString() method
public static String toString(boolean b)
Parameters
b − the boolean to be converted
Return Value
This method returns the string representation of the specified boolean.
Exception
NA
Example 1
The following example shows the usage of Boolean toString() method for a boolean value as true.
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 1 bool variable b1 boolean b1; // assign value to b1 b1 = true; // create 1 String s1 String s1; // assign string value of object b1 to s1 s1 = Boolean.toString(b1); String str1 = "String value of boolean object " + b1 + " is " + s1; // print s1 value System.out.println( str1 ); } }
Output
Let us compile and run the above program, this will produce the following result −
String value of boolean value true is true
Example 2
The following example shows the usage of Boolean toString() method for a boolean value as false.
package com.tutorialspoint; public class BooleanDemo { public static void main(String[] args) { // create 1 bool variable b1 boolean b1; // assign value to b1 b1 = false; // create 1 String s1 String s1; // assign string value of object b1 to s1 s1 = Boolean.toString(b1); String str1 = "String value of boolean object " + b1 + " is " + s1; // print s1 value System.out.println( str1 ); } }
Output
Let us compile and run the above program, this will produce the following result −
String value of boolean value false is false
java_lang_boolean.htm
Advertisements