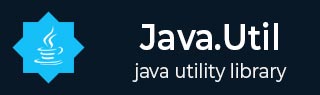
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TreeSet Class
Introduction
The Java TreeSet class implements the Set interface. Following are the important points about TreeSet −
The TreeSet class guarantees that the Map will be in ascending key order and backed by a TreeMap.
The Map is sorted according to the natural sort method for the key Class, or by the Comparator provided at set creation time, that will depend on which constructor used.
The ordering must be total in order for the Tree to function properly.
Class declaration
Following is the declaration for java.util.TreeSet class −
public class TreeSet<E> extends AbstractSet<E> implements NavigableSet<E>, Cloneable, Serializable
Parameters
Following is the parameter for java.util.TreeSet class −
E − This is the type of elements maintained by this set.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | TreeSet() This constructor constructs a new, empty tree set, sorted according to the natural ordering of its elements. |
2 | TreeSet(Collection<? extends E> c) This constructor constructs a new tree set containing the elements in the specified collection, sorted according to the natural ordering of its elements. |
3 | TreeSet(Comparator<? super E> comparator) This constructor constructs a new, empty tree set, sorted according to the specified comparator. |
4 | TreeSet(SortedSet<E> s) This constructor constructs a new tree set containing the same elements and using the same ordering as the specified sorted set. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean add(E e)
This method adds the specified element to this set if it is not already present. |
2 | boolean addAll(Collection<? extends E> c)
This method adds all of the elements in the specified collection to this set. |
3 | E ceiling(E e)
This method returns the least element in this set greater than or equal to the given element, or null if there is no such element. |
4 | void clear()
This method removes all of the elements from this set. |
5 | Object clone()
This method returns a shallow copy of this TreeSet instance. |
6 | boolean contains(Object o)
This method returns true if this set contains the specified element. |
7 | Iterator<E> descendingIterator()
This method returns an iterator over the elements in this set in descending order. |
8 | NavigableSet<E> descendingSet()
This method returns a reverse order view of the elements contained in this set. |
9 | E first()
This method returns the first (lowest) element currently in this set. |
10 | E floor(E e)
This method Returns the greatest element in this set less than or equal to the given element, or null if there is no such element. |
11 | SortedSet<E> headSet(E toElement)
This method returns a view of the portion of this set whose elements are strictly less than toElement. |
12 | E higher(E e)
This method returns the least element in this set strictly greater than the given element, or null if there is no such element. |
13 | boolean isEmpty()
This method returns true if this set contains no elements. |
14 | Iterator<E> iterator()
This method returns an iterator over the elements in this set in ascending order. |
15 | E last()
This method returns the last (highest) element currently in this set. |
16 | E lower(E e)
This method returns the greatest element in this set strictly less than the given element, or null if there is no such element. |
17 | E pollFirst()
This method retrieves and removes the first (lowest) element, or returns null if this set is empty. |
18 | E pollLast()
This method retrieves and removes the last (highest) element, or returns null if this set is empty. |
19 | boolean remove(Object o)
This method removes the specified element from this set if it is present. |
20 | int size()
This method returns the number of elements in this set (its cardinality). |
21 | Spliterator<E> spliterator()
Creates a late-binding and fail-fast Spliterator over the elements in this set. |
22 | SortedSet<E> subSet(E fromElement, E toElement)
This method returns a view of the portion of this set whose elements range from fromElement, inclusive, to toElement, exclusive. |
23 | SortedSet<E> tailSet(E fromElement)
This method returns a view of the portion of this set whose elements are greater than or equal to fromElement. |
Methods inherited
This class inherits methods from the following classes −
- java.util.AbstractSet
- java.util.AbstractCollection
- java.util.Object
- java.util.Set
Adding Entries to a TreeSet Example
The following example shows the usage of Java TreeSet add() method to add entries to the treeset. We've created a TreeSet object of Integer. Then few entries are added using add() method and treeset object is printed to check its content.
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<Integer> treeset = new TreeSet<>(); // adding in the tree set treeset.add(12); treeset.add(13); treeset.add(14); treeset.add(15); // displaying the Tree set data System.out.print("Tree set : " + treeset); } }
Output
Let us compile and run the above program, this will produce the following result.
Tree set : [12, 13, 14, 15]