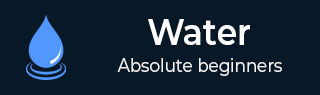
- Watir Tutorial
- Watir - Home
- Watir - Overview
- Watir - Introduction
- Watir - Environment Setup
- Watir - Installing Drivers for Browsers
- Watir - Working with Browsers
- Watir - Web Elements
- Watir - Locating Web Elements
- Watir - Working with Iframes
- Watir - Automatic Waits
- Watir - Headless Testing
- Watir - Mobile Testing
- Watir - Capturing Screenshots
- Watir - Page Objects
- Watir - Page Performance
- Watir - Cookies
- Watir - Proxies
- Watir - Alerts
- Watir - Downloads
- Watir - Browser Windows
- Watir Useful Resources
- Watir - Quick Guide
- Watir - Useful Resources
- Watir - Discussion
Watir - Automatic Waits
In this chapter, let us understand waits in detail. To understand automatic waits, we have created a simple test page. When user enters text in the textbox onchange event is fired and after 3-seconds the button is enabled.
Watir has a wait_unit api call which waits on a particular event or property. We will test the same for the test page as given below −
Syntax
browser.button(id: 'btnsubmit').wait_until(&:enabled?) //here the wait is on the button with id : btnsubmit to be enabled.
testwait.html
<html> <head> <title>Testing UI using Watir</title> </head> <body> <script type = "text/javascript"> function wsentered() { setTimeout(function() { document.getElementById("btnsubmit").disabled = false; }, 3000); } function wsformsubmitted() { document.getElementById("showmessage").style.display = ""; } </script> <div id = "divfirstname"> Enter First Name : <input type = "text" id = "firstname" name = "firstname" onchange = "wsentered()" /> </div> <br/> <br/> <button id = "btnsubmit" disabled onclick = "wsformsubmitted();">Submit</button> <br/< <br/< <div id = "showmessage" style = "display:none;color:green;font-size:25px;">l; Button is clicked </div> </body> </html>
Output
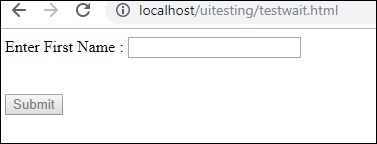
When you enter the text in the textbox, you will have to wait for 3 seconds for the button to be enabled.

When you click the Submit button, the following text is displayed −
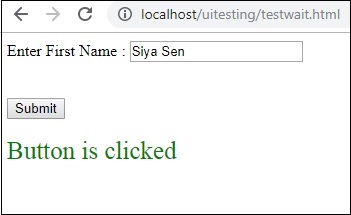
Now since we have added delay for the button to be enabled, it is difficult for the automation to handle such cases. Whenever we have some delay or have to wait on some event or property of the element to be located, we can make use of wait_until as shown below −
Watir code using wait_until
require 'watir' b = Watir::Browser.new :chrome b.goto('http://localhost/uitesting/testwait.html') t = b.text_field(name: 'firstname') t.exists? t.set 'Riya Kapoor' b.screenshot.save 'waittestbefore.png' t.value t.fire_event('onchange') btn = b.button(id: 'btnsubmit').wait_until(&:enabled?) btn.fire_event('onclick'); b.screenshot.save 'waittestafter.png'
Next, use the following command
btn = b.button(id: 'btnsubmit').wait_until(&:enabled?)
Watir is going to wait for the button to get enabled and later go for click event to be fired. The screenshots captured are shown below −
Waittestbefore.png
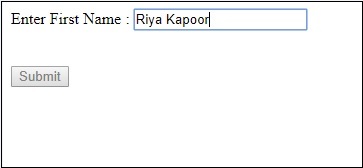
waittestafter.png
