
- TypeScript Tutorial
- TypeScript - Home
- TypeScript - Overview
- TypeScript - Environment Setup
- TypeScript - Basic Syntax
- TypeScript - Types
- TypeScript - Variables
- TypeScript - Operators
- TypeScript - Decision Making
- TypeScript - Loops
- TypeScript - Functions
- TypeScript - Numbers
- TypeScript - Strings
- TypeScript - Arrays
- TypeScript - Tuples
- TypeScript - Union
- TypeScript - Interfaces
- TypeScript - Classes
- TypeScript - Objects
- TypeScript - Namespaces
- TypeScript - Modules
- TypeScript - Ambients
- TypeScript Useful Resources
- TypeScript - Quick Guide
- TypeScript - Useful Resources
- TypeScript - Discussion
TypeScript - Interfaces
An interface is a syntactical contract that an entity should conform to. In other words, an interface defines the syntax that any entity must adhere to.
Interfaces define properties, methods, and events, which are the members of the interface. Interfaces contain only the declaration of the members. It is the responsibility of the deriving class to define the members. It often helps in providing a standard structure that the deriving classes would follow.
Let’s consider an object −
var person = { FirstName:"Tom", LastName:"Hanks", sayHi: ()=>{ return "Hi"} };
If we consider the signature of the object, it could be −
{ FirstName:string, LastName:string, sayHi()=>string }
To reuse the signature across objects we can define it as an interface.
Declaring Interfaces
The interface keyword is used to declare an interface. Here is the syntax to declare an interface −
Syntax
interface interface_name { }
Example: Interface and Objects
interface IPerson { firstName:string, lastName:string, sayHi: ()=>string } var customer:IPerson = { firstName:"Tom", lastName:"Hanks", sayHi: ():string =>{return "Hi there"} } console.log("Customer Object ") console.log(customer.firstName) console.log(customer.lastName) console.log(customer.sayHi()) var employee:IPerson = { firstName:"Jim", lastName:"Blakes", sayHi: ():string =>{return "Hello!!!"} } console.log("Employee Object ") console.log(employee.firstName); console.log(employee.lastName);
The example defines an interface. The customer object is of the type IPerson. Hence, it will now be binding on the object to define all properties as specified by the interface.
Another object with following signature, is still considered as IPerson because that object is treated by its size or signature.
On compiling, it will generate following JavaScript code.
//Generated by typescript 1.8.10 var customer = { firstName: "Tom", lastName: "Hanks", sayHi: function () { return "Hi there"; } }; console.log("Customer Object "); console.log(customer.firstName); console.log(customer.lastName); console.log(customer.sayHi()); var employee = { firstName: "Jim", lastName: "Blakes", sayHi: function () { return "Hello!!!"; } }; console.log("Employee Object "); console.log(employee.firstName); console.log(employee.lastName);
The output of the above example code is as follows −
Customer object Tom Hanks Hi there Employee object Jim Blakes Hello!!!
Interfaces are not to be converted to JavaScript. It’s just part of TypeScript. If you see the screen shot of TS Playground tool there is no java script emitted when you declare an interface unlike a class. So interfaces have zero runtime JavaScript impact.
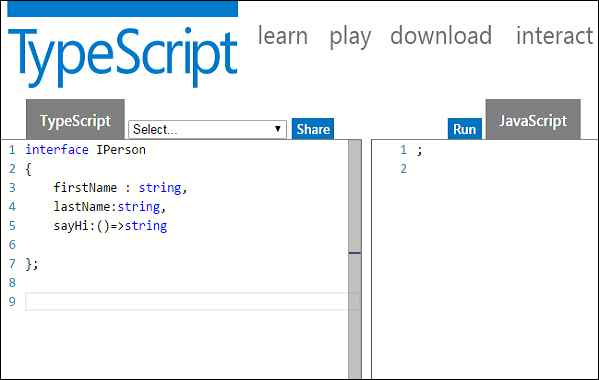
Union Type and Interface
The following example shows the use of Union Type and Interface −
interface RunOptions { program:string; commandline:string[]|string|(()=>string); } //commandline as string var options:RunOptions = {program:"test1",commandline:"Hello"}; console.log(options.commandline) //commandline as a string array options = {program:"test1",commandline:["Hello","World"]}; console.log(options.commandline[0]); console.log(options.commandline[1]); //commandline as a function expression options = {program:"test1",commandline:()=>{return "**Hello World**";}}; var fn:any = options.commandline; console.log(fn());
On compiling, it will generate following JavaScript code.
//Generated by typescript 1.8.10 //commandline as string var options = { program: "test1", commandline: "Hello" }; console.log(options.commandline); //commandline as a string array options = { program: "test1", commandline: ["Hello", "World"] }; console.log(options.commandline[0]); console.log(options.commandline[1]); //commandline as a function expression options = { program: "test1", commandline: function () { return "**Hello World**"; } }; var fn = options.commandline; console.log(fn());
Its output is as follows −
Hello Hello World **Hello World**
Interfaces and Arrays
Interface can define both the kind of key an array uses and the type of entry it contains. Index can be of type string or type number.
Example
interface namelist { [index:number]:string } var list2:namelist = ["John",1,"Bran"] //Error. 1 is not type string interface ages { [index:string]:number } var agelist:ages; agelist["John"] = 15 // Ok agelist[2] = "nine" // Error
Interfaces and Inheritance
An interface can be extended by other interfaces. In other words, an interface can inherit from other interface. Typescript allows an interface to inherit from multiple interfaces.
Use the extends keyword to implement inheritance among interfaces.
Syntax: Single Interface Inheritance
Child_interface_name extends super_interface_name
Syntax: Multiple Interface Inheritance
Child_interface_name extends super_interface1_name, super_interface2_name,…,super_interfaceN_name
Example: Simple Interface Inheritance
interface Person { age:number } interface Musician extends Person { instrument:string } var drummer = <Musician>{}; drummer.age = 27 drummer.instrument = "Drums" console.log("Age: "+drummer.age) console.log("Instrument: "+drummer.instrument)
On compiling, it will generate following JavaScript code.
//Generated by typescript 1.8.10 var drummer = {}; drummer.age = 27; drummer.instrument = "Drums"; console.log("Age: " + drummer.age); console.log("Instrument: " + drummer.instrument);
Its output is as follows −
Age: 27 Instrument: Drums
Example: Multiple Interface Inheritance
interface IParent1 { v1:number } interface IParent2 { v2:number } interface Child extends IParent1, IParent2 { } var Iobj:Child = { v1:12, v2:23} console.log("value 1: "+this.v1+" value 2: "+this.v2)
The object Iobj is of the type interface leaf. The interface leaf by the virtue of inheritance now has two attributes- v1 and v2 respectively. Hence, the object Iobj must now contain these attributes.
On compiling, it will generate following JavaScript code.
//Generated by typescript 1.8.10 var Iobj = { v1: 12, v2: 23 }; console.log("value 1: " + this.v1 + " value 2: " + this.v2);
The output of the above code is as follows −
value 1: 12 value 2: 23