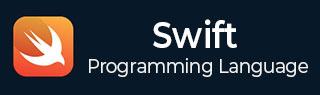
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Nested If Statements
In Swift, you are allowed to nest an if statement inside another if statement. So when the condition of the outer if statement is true, then only controls can access the nested if statement. Otherwise, the controls skipped the nested if statement and executed the block of code present right after the outer if statement.
You are also allowed to nest an if statement inside an if-else statement or vice-versa. You can also nest as many if statements as you want but try to avoid excessive nesting because it's hard to maintain if you encounter an error.
Syntax
Following is the syntax of the nested if statement −
if boolean_expression_1{ /* statement(s) will execute if the boolean expression 1 is true */ If boolean_expression_2{ /* statement(s) will execute if the boolean expression 2 is true */ } }
You can nest else if...else in a similar way as you have nested if statement.
Flow Diagram
The following flow diagram will show how the nested if statement works.

Example
Swift program to demonstrate the use of nested if statements.
import Foundation var varA:Int = 100; var varB:Int = 200; /* Check the boolean condition using if statement */ if varA == 100 { /* If the condition is true then print the following */ print("First condition is satisfied") if varB == 200 { /* If the condition is true then print the following */ print("Second condition is also satisfied") } } print("Value of variable varA is \(varA)") print("Value of variable varB is \(varB)")
Output
It will produce the following output −
First condition is satisfied Second condition is also satisfied Value of variable varA is 100 Value of variable varB is 200
Example
Swift program to find the leap year using nested if-else statements.
import Foundation let myYear = 2027 // Checking leap year if myYear % 4 == 0 { if myYear % 100 == 0 { if myYear % 400 == 0 { print("\(myYear) is a leap year.") } else { print("\(myYear) is not a leap year.") } } else { print("\(myYear) is a leap year.") } } else { print("\(myYear) is not a leap year.") }
Output
It will produce the following output −
2027 is not a leap year.
Example
Swift program to check if the given number is positive or negative even or odd number.
import Foundation let num = -11 // Checking if the given number is positive // or negative even or odd number if num > 0 { if num % 2 == 0 { print("Positive even number.") } else { print("Positive odd number.") } } else if num < 0 { if num % 2 == 0 { print("Negative even number.") } else { print("Negative odd number.") } } else { print("Number is zero.") }
Output
It will produce the following output −
Negative odd number.