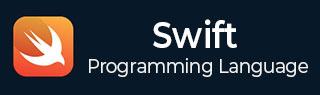
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - If... Else Statement
An if-else statement allows you to execute different blocks of code according to the given expression. If the given condition is true, then the code present inside the if statement will execute and if the given condition is false, then the code present inside the else statement will execute.
Or we can say that an if-else statement is the modified version of an if statement where an if statement can be followed by an optional else statement, which executes when the Boolean expression is false.
For example, Ram is going to market and his mother tells him that if you find apples on sale then buy them otherwise buy grapes. So here the if condition is "Apple on sale" and the else condition is "buy Grapes". Hence Ram will buy Apple only when the if condition is true. Otherwise, he will buy grapes.
Syntax
Following is the syntax of the if…else statement −
if boolean_expression{ /* statement(s) will execute if the boolean expression is true */ } else{ /* statement(s) will execute if the boolean expression is false */ }
If the Boolean expression evaluates to true, then the if block of code will be executed, otherwise else block of code will be executed.
Flow Diagram
The following flow diagram will show how if…else statement works.
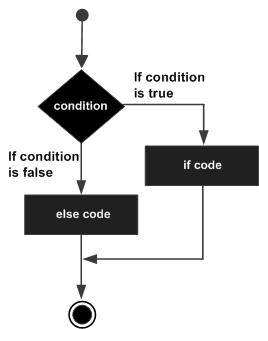
Example
Swift program to demonstrate the use of if… else statement.
import Foundation var varA:Int = 100; /* Check the boolean condition using if statement */ if varA < 20 { /* If the condition is true then print the following */ print("varA is less than 20"); } else { /* If the condition is false then print the following */ print("varA is not less than 20"); } print("Value of variable varA is \(varA)");
Output
It will produce the following output −
varA is not less than 20 Value of variable varA is 100
Example
Swift program to check even or odd numbers using if-else statement.
import Foundation let num = 41 if num % 2 == 0 { print("Entered number is even.") } else { print("Entered number is odd.") }
Output
It will produce the following output −
Entered number is odd.
Example
Swift program to check the correct username using an if-else statement.
import Foundation let username = "123Admin22" let inputUsername = "123Admin22" if username == inputUsername { print("Login successful.") } else { print("Invalid username.") }
Output
It will produce the following output −
Login successful.