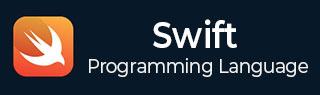
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - If... Else Statement
An if statement can be followed by an optional else statement, which executes when the Boolean expression is false.
Syntax
The syntax of an if...else statement in Swift is as follows −
if boolean_expression { /* statement(s) will execute if the boolean expression is true */ } else { /* statement(s) will execute if the boolean expression is false */ }
If the Boolean expression evaluates to true, then the if block of code will be executed, otherwise else block of code will be executed.
Flow Diagram
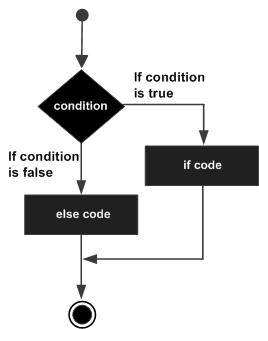
Example
var varA:Int = 100; /* Check the boolean condition using if statement */ if varA < 20 { /* If condition is true then print the following */ println("varA is less than 20"); } else { /* If condition is false then print the following */ println("varA is not less than 20"); } println("Value of variable varA is \(varA)");
When the above code is compiled and executed, it produces the following result −
varA is not less than 20 Value of variable varA is 100
swift_decision_making.htm
Advertisements
To Continue Learning Please Login