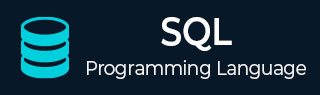
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Databases
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
SQL - UPDATE View
SQL UPDATE View Statement
A view is a database object that can contain rows (all or selected) from an existing table. It can be created from one or many tables which depends on the provided SQL query to create a view.
Unlike CREATE VIEW and DROP VIEW there is no direct statement to update the records of an existing view. We can use the SQL UPDATE Statement to modify the existing records in a table or a view.
Syntax
The basic syntax of the UPDATE query with a WHERE clause is as follows −
UPDATE view_name SET column1 = value1, column2 = value2...., columnN = valueN WHERE [condition];
You can combine N number of conditions using the AND or the OR operators.
Example
Assume we have created a table named CUSTOMERS using the CREATE TABLE statement using the following query −
CREATE TABLE CUSTOMERS( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, ADDRESS CHAR (25) , SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
Now, insert values into this table using the INSERT statement as follows −
INSERT INTO CUSTOMERS VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00 ), (2, 'Khilan', 25, 'Delhi', 1500.00 ), (3, 'Kaushik', 23, 'Kota', 2000.00 ), (4, 'Chaitali', 25, 'Mumbai', 6500.00 ), (5, 'Hardik', 27, 'Bhopal', 8500.00 ), (6, 'Komal', 22, 'Hyderabad', 4500.00 ), (7, 'Muffy', 24, 'Indore', 10000.00 );
Following query creates a view based on the above created table −
CREATE VIEW CUSTOMERS_VIEW AS SELECT * FROM CUSTOMERS;
You can verify the contents of a view using the SELECT query as shown below −
SELECT * FROM CUSTOMERS_VIEW;
The view will be displayed as follows −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
4 | Chaitali | 25 | Mumbai | 6500.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | 4500.00 |
7 | Muffy | 24 | Indore | 10000.00 |
Following query updates the age of Ramesh to 35 in the above created CUSTOMERS_VIEW −
UPDATE CUSTOMERS_VIEW SET AGE = 35 WHERE name = 'Ramesh';
Verification
You can verify the contents of the CUSTOMERS_VIEW using the SELECT statement as follows −
SELECT * FROM CUSTOMERS_VIEW WHERE NAME ='Ramesh';
The resultant view would have the following record(s) −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 35 | Ahmedabad | 2000.00 |
Example
The following query will update the ADDRESS of a customer whose ID is 6 in the CUSTOMERS_VIEW.
UPDATE CUSTOMERS_VIEW SET ADDRESS = 'Pune' WHERE ID = 6;
Output
The query produces the following output −
Query OK, 1 row affected (0.21 sec) Rows matched: 1 Changed: 1 Warnings: 0
Verification
If you retrieve the record with ID value 6 using the SELECT statement as −
SELECT * FROM CUSTOMERS_VIEW WHERE ID=6;
The record returned would be −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
6 | Komal | 22 | Hyderabad | 4500.00 |
Updating Multiple Rows and Columns
Using UPDATE statement, multiple rows and columns in a view/table can also be updated. While updating multiple rows, specify the condition in a WHERE clause such that only required rows would satisfy it.
Example
Following query updates the NAME and AGE column values in the CUSTOMERS_VIEW of the record with ID value 3.
UPDATE CUSTOMERS_VIEW SET NAME = 'Kaushik Ramanujan', AGE = 24 WHERE ID = 3;
Output
The query produces the following output −
Query OK, 1 row affected (0.07 sec) Rows matched: 1 Changed: 1 Warnings: 0
Verification
You can verify whether the record is updated or not, using the following query −
SELECT * FROM CUSTOMERS_VIEW WHERE ID = 3;
The record returned would be −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
3 | Kaushik Ramanujan | 24 | Kota | 2000.00 |
Example
But if you want to modify/update the age values of all the records in the CUSTOMERS_VIEW, there is no need to use the WHERE clause.
UPDATE CUSTOMERS_VIEW SET AGE = AGE+6;
Output
This query produces the following output −
Query OK, 7 rows affected (0.10 sec) Rows matched: 7 Changed: 7 Warnings: 0
Verification
To verify whether the records of the CUSTOMERS_VIEW are modified or not, use the following SELECT query −
SELECT * FROM CUSTOMERS_VIEW;
The resultant CUSTOMERS_VIEW would have the following records −
ID | NAME | AGE |
---|---|---|
1 | Ramesh | 41 |
2 | Khilan | 31 |
3 | Kaushik Ramanujan | 30 |
4 | Chaitali | 31 |
5 | Hardik | 33 |
6 | Komal | 28 |
7 | Muffy | 30 |