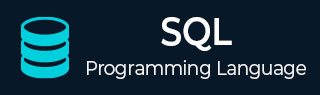
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Databases
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
SQL - Handling Duplicates
Sometimes, tables or result sets contain duplicate records. While in most cases, duplicates are allowed, there are situations where it is necessary to prevent duplicate records and remove them from a database table.
Why is Handling Duplicates in SQL Necessary?
Handling duplicates in an SQL database becomes necessary to prevent the following consequences −
The existence of duplicates in an organizational database will lead to logical errors.
Duplicate data occupies space in the storage, which leads to decrease in usage efficiency of a database.
Due to the increased use of resources, the overall cost of the handling resources rises.
With increase in logical errors due to the presence of duplicates, the conclusions derived from data analysis in a database will also be erroneous.
This chapter will describe how to prevent the occurrence of duplicate records in a table and how to remove the already existing duplicate records.
Preventing Duplicate Entries
To prevent the entry of duplicate records into a table, we can define a PRIMARY KEY or a UNIQUE Index on the relevant fields. These database constraints ensure that each entry in the specified column or set of columns is unique.
Example
Let us create a CUSTOMERS table using the following query −
CREATE TABLE CUSTOMERS ( FIRST_NAME CHAR(20), LAST_NAME CHAR(20), SEX CHAR(10) );
As we have not defined any constraints on the table, duplicate records can be inserted into it. To prevent such cases, add a PRIMARY KEY constraint on relevant fields (say LAST_NAME and FIRST_NAME together) −
ALTER TABLE CUSTOMERS ADD PRIMARY KEY (LAST_NAME, FIRST_NAME);
Using INSERT IGNORE Query:
Alternatively, we can use the INSERT IGNORE statement to insert records without generating an error for duplicates as shown below −
INSERT IGNORE INTO CUSTOMERS (LAST_NAME, FIRST_NAME) VALUES ( 'Jay', 'Thomas'), ( 'Jay', 'Thomas');
As you can see below, the table will only consist of a single record (ignoring the duplicate value).
FIRST_NAME | LAST_NAME | SEX |
---|---|---|
Thomas | Jay | NULL |
Using REPLACE Query:
Or, use the REPLACE statement to replace duplicates as shown in the following query −
REPLACE INTO CUSTOMERS (LAST_NAME, FIRST_NAME) VALUES ( 'Ajay', 'Kumar'), ( 'Ajay', 'Kumar');
The table will contain the following records −
FIRST_NAME | LAST_NAME | SEX |
---|---|---|
Kumar | Ajay | NULL |
Thomas | Jay | NULL |
The choice between the INSERT IGNORE and REPLACE statements should be made based on the desired duplicate-handling behaviour. The INSERT IGNORE statement retains the first set of duplicate records and discards any subsequent duplicates. Conversely, the REPLACE statement preserves the last set of duplicates and erases any earlier ones.
Using UNIQUE Constraint:
Another way to enforce uniqueness in a table is by adding a UNIQUE constraint rather than a PRIMARY KEY constraint −
CREATE TABLE BUYERS ( FIRST_NAME CHAR(20) NOT NULL, LAST_NAME CHAR(20) NOT NULL, SEX CHAR(10), UNIQUE (LAST_NAME, FIRST_NAME) );
Counting and Identifying Duplicates
To count and identify duplicate records based on specific columns, we can use the COUNT function and GROUP BY clause.
Example
Following is the query to count duplicate records with FIRST_NAME and LAST_NAME in the BUYERS −
SELECT COUNT(*) as repetitions, LAST_NAME, FIRST_NAME FROM BUYERS GROUP BY LAST_NAME, FIRST_NAME HAVING repetitions > 1;
This query will return a list of all the duplicate records in the PERSON_TABLE table. To identify sets of values that are duplicated, follow the steps given below −
Determine which columns contain the values that may be duplicated.
List those columns in the column selection list, along with the COUNT(*).
List the columns in the GROUP BY clause as well.
Add a HAVING clause that eliminates the unique values by requiring the group counts to be greater than one.
Eliminating Duplicates from a Table
We can use the DISTINCT keyword along with the SELECT statement to retrieve unique records from a table.
SELECT DISTINCT LAST_NAME, FIRST_NAME FROM BUYERS ORDER BY LAST_NAME;
Alternatively, you can include a GROUP BY clause specifying the columns you are selecting to eliminate duplicates −
SELECT LAST_NAME, FIRST_NAME FROM BUYERS GROUP BY LAST_NAME, FIRST_NAME;