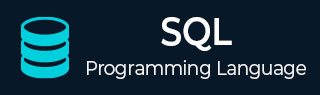
- SQL Tutorial
- SQL - Home
- SQL - Overview
- SQL - RDBMS Concepts
- SQL - Databases
- SQL - Syntax
- SQL - Data Types
- SQL - Operators
- SQL - Expressions
- SQL Database
- SQL - Create Database
- SQL - Drop Database
- SQL - Select Database
- SQL - Rename Database
- SQL - Show Databases
- SQL - Backup Database
- SQL Table
- SQL - Create Table
- SQL - Show Tables
- SQL - Rename Table
- SQL - Truncate Table
- SQL - Clone Tables
- SQL - Temporary Tables
- SQL - Alter Tables
- SQL - Drop Table
- SQL - Delete Table
- SQL - Constraints
- SQL Queries
- SQL - Insert Query
- SQL - Select Query
- SQL - Select Into
- SQL - Insert Into Select
- SQL - Update Query
- SQL - Delete Query
- SQL - Sorting Results
- SQL Views
- SQL - Create Views
- SQL - Update Views
- SQL - Drop Views
- SQL - Rename Views
- SQL Operators and Clauses
- SQL - Where Clause
- SQL - Top Clause
- SQL - Distinct Clause
- SQL - Order By Clause
- SQL - Group By Clause
- SQL - Having Clause
- SQL - AND & OR
- SQL - BOOLEAN (BIT) Operator
- SQL - LIKE Operator
- SQL - IN Operator
- SQL - ANY, ALL Operators
- SQL - EXISTS Operator
- SQL - CASE
- SQL - NOT Operator
- SQL - NOT EQUAL
- SQL - IS NULL
- SQL - IS NOT NULL
- SQL - NOT NULL
- SQL - BETWEEN Operator
- SQL - UNION Operator
- SQL - UNION vs UNION ALL
- SQL - INTERSECT Operator
- SQL - EXCEPT Operator
- SQL - Aliases
- SQL Joins
- SQL - Using Joins
- SQL - Inner Join
- SQL - Left Join
- SQL - Right Join
- SQL - Cross Join
- SQL - Full Join
- SQL - Self Join
- SQL - Delete Join
- SQL - Update Join
- SQL - Left Join vs Right Join
- SQL - Union vs Join
- SQL Keys
- SQL - Unique Key
- SQL - Primary Key
- SQL - Foreign Key
- SQL - Composite Key
- SQL - Alternate Key
- SQL Indexes
- SQL - Indexes
- SQL - Create Index
- SQL - Drop Index
- SQL - Show Indexes
- SQL - Unique Index
- SQL - Clustered Index
- SQL - Non-Clustered Index
- Advanced SQL
- SQL - Wildcards
- SQL - Comments
- SQL - Injection
- SQL - Hosting
- SQL - Min & Max
- SQL - Null Functions
- SQL - Check Constraint
- SQL - Default Constraint
- SQL - Stored Procedures
- SQL - NULL Values
- SQL - Transactions
- SQL - Sub Queries
- SQL - Handling Duplicates
- SQL - Using Sequences
- SQL - Auto Increment
- SQL - Date & Time
- SQL - Cursors
- SQL - Common Table Expression
- SQL - Group By vs Order By
- SQL - IN vs EXISTS
- SQL - Database Tuning
- SQL Function Reference
- SQL - Date Functions
- SQL - String Functions
- SQL - Aggregate Functions
- SQL - Numeric Functions
- SQL - Text & Image Functions
- SQL - Statistical Functions
- SQL - Logical Functions
- SQL - Cursor Functions
- SQL - JSON Functions
- SQL - Conversion Functions
- SQL - Datatype Functions
- SQL Useful Resources
- SQL - Questions and Answers
- SQL - Quick Guide
- SQL - Useful Functions
- SQL - Useful Resources
- SQL - Discussion
SQL - Alias Syntax
You can rename a table or a column in a database temporarily by giving them another pseudo name. This pseudo name is known as Alias. The use of aliases is to address a specific table or a column in an SQL statement without changing their original name in the database. Aliases are created with the AS keyword.
Aliases can be especially useful when working with complex queries involving multiple tables or columns with similar names. By assigning temporary names to these tables or columns, you can make your SQL query more readable and easier to understand.
The SQL Aliasing
Aliases are used to address database tables with a shorter or more meaningful name within an SQL query. The basic syntax of a table alias is as follows.
SELECT column1, column2.... FROM table_name AS alias_name;
Example
Assume we have created a table with name CUSTOMERS in MySQL database using CREATE TABLE statement as shown below −
CREATE TABLE CUSTOMERS ( ID INT NOT NULL, NAME VARCHAR (20) NOT NULL, AGE INT NOT NULL, ADDRESS CHAR (25), SALARY DECIMAL (18, 2), PRIMARY KEY (ID) );
Following query inserts values into this table using the INSERT statement −
INSERT INTO CUSTOMERS VALUES (1, 'Ramesh', 32, 'Ahmedabad', 2000.00), (2, 'Khilan', 25, 'Delhi', 1500.00), (3, 'Kaushik', 23, 'Kota', 2000.00), (4, 'Chaitali', 25, 'Mumbai', 6500.00), (5, 'Hardik', 27, 'Bhopal', 8500.00), (6, 'Komal', 22, 'Hyderabad', 4500.00), (7, 'Muffy', 24, 'Indore', 10000.00);
The CUSTOMERS table obtained is as follows −
ID | NAME | AGE | ADDRESS | SALARY |
---|---|---|---|---|
1 | Ramesh | 32 | Ahmedabad | 2000.00 |
2 | Khilan | 25 | Delhi | 1500.00 |
3 | Kaushik | 23 | Kota | 2000.00 |
4 | Chaitali | 25 | Mumbai | 6500.00 |
5 | Hardik | 27 | Bhopal | 8500.00 |
6 | Komal | 22 | Hyderabad | 4500.00 |
7 | Muffy | 24 | Indore | 10000.00 |
Now, we are creating the second table ORDERS using CREATE TABLE statement as shown below −
CREATE TABLE ORDERS ( OID INT NOT NULL, DATES DATETIME NOT NULL, CUSTOMER_ID INT NOT NULL, AMOUNT INT NOT NULL, PRIMARY KEY (OID) );
Following query inserts values into this table using the INSERT statement −
INSERT INTO ORDERS VALUES (102, '2009-10-08 00:00:00', 3, 3000), (100, '2009-10-08 00:00:00', 3, 1500), (101, '2009-11-20 00:00:00', 2, 1560), (103, '2008-05-20 00:00:00', 4, 2060);
The ORDERS table obtained is as shown below −
OID | DATE | CUSTOMER_ID | AMOUNT |
---|---|---|---|
102 | 2009-10-08 00:00:00 | 3 | 3000.00 |
100 | 2009-10-08 00:00:00 | 3 | 1500.00 |
101 | 2009-11-20 00:00:00 | 2 | 1560.00 |
103 | 2008-05-20 00:00:00 | 4 | 2060.00 |
Now, the following query shows the usage of a table alias. The CUSTOMERS table is aliased as 'C' and the ORDERS table is aliased as 'O' −
SELECT C.ID, C.NAME, C.AGE, O.AMOUNT FROM CUSTOMERS AS C, ORDERS AS O WHERE C.ID = O.CUSTOMER_ID;
Output
This would produce the following result −
ID | NAME | AGE | AMOUNT |
---|---|---|---|
3 | Kaushik | 23 | 3000.00 |
3 | Kaushik | 23 | 1500.00 |
2 | Khilan | 25 | 1560.00 |
4 | Chaitali | 25 | 2060.00 |
Aliasing Column Names
We can also use an alias for a column name in SQL to give it a different name in the result set of a query. The basic syntax of a column alias is as follows −
SELECT column_name AS alias_name FROM table_name;
Example
Following is the usage of a column alias. Here, the NAME column is aliased as 'CUSTOMER_NAME' −
SELECT ID AS CUSTOMER_ID, NAME AS CUSTOMER_NAME FROM CUSTOMERS;
Output
This would produce the following result −
CUSTOMER_ID | CUSTOMER_NAME |
---|---|
1 | Ramesh |
2 | Khilan |
3 | Kaushik |
4 | Chaitali |
5 | Hardik |
6 | Komal |
7 | Muffy |
Aliasing with Self Join
The SQL Self Join is used to join a table to itself as if the table were two tables. During this process, we need to use alias for one of the tables with a temporary name to avoid misunderstandings. This renaming is done using aliases.
Syntax
Following is the syntax for performing a self-join with aliases −
SELECT column_name(s) FROM my_table a, my_table b ON a.join_column = b.join_column;
Example
Now, let us join the CUSTOMERS table to itself using the following Self Join query. Our aim is to establish a relationship among customers on the basis of their earnings. In here, we are using aliases with column names as well as with the table names −
SELECT a.ID, b.NAME as EARNS_HIGHER, a.NAME as EARNS_LESS, a.SALARY as LOWER_SALARY FROM CUSTOMERS a, CUSTOMERS b WHERE a.SALARY < b.SALARY;
Output
Output of the above query is as follows −
ID | EARNS_HIGHER | EARNS_LESS | LOWER_SALARY |
---|---|---|---|
2 | Ramesh | Khilan | 1500.00 |
2 | Kaushik | Khilan | 1500.00 |
6 | Chaitali | Komal | 4500.00 |
3 | Chaitali | Kaushik | 2000.00 |
2 | Chaitali | Khilan | 1500.00 |
1 | Chaitali | Ramesh | 2000.00 |
6 | Hardik | Komal | 4500.00 |
4 | Hardik | Chaitali | 6500.00 |
3 | Hardik | Kaushik | 2000.00 |
2 | Hardik | Khilan | 1500.00 |
1 | Hardik | Ramesh | 2000.00 |
3 | Komal | Kaushik | 2000.00 |
2 | Komal | Khilan | 1500.00 |
1 | Komal | Ramesh | 2000.00 |
6 | Muffy | Komal | 4500.00 |
5 | Muffy | Hardik | 8500.00 |
4 | Muffy | Chaitali | 6500.00 |
3 | Muffy | Kaushik | 2000.00 |
2 | Muffy | Khilan | 1500.00 |
1 | Muffy | Ramesh | 2000.00 |