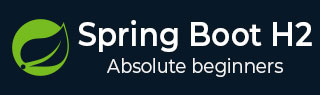
- Spring Boot & H2 Tutorial
- Spring Boot & H2 - Home
- Spring Boot & H2 - Overview
- Spring Boot & H2 - Environment Setup
- Spring Boot & H2 - Project Setup
- Spring Boot & H2 - REST APIs
- Spring Boot & H2 - H2 Console
- Spring Boot & H2 Examples
- Spring Boot & H2 - Add Record
- Spring Boot & H2 - Get Record
- Spring Boot & H2 - Get All Records
- Spring Boot & H2 - Update Record
- Spring Boot & H2 - Delete Record
- Spring Boot & H2 - Unit Test Controller
- Spring Boot & H2 - Unit Test Service
- Spring Boot & H2 - Unit Test Repository
- Spring Boot & H2 Useful Resources
- Spring Boot & H2 - Quick Guide
- Spring Boot & H2 - Useful Resources
- Spring Boot & H2 - Discussion
Spring Boot & H2 - Get Record
Let's now update the project created so far to prepare a complete Get Record API and test it.
Update Service
// Use repository.findById() to get Employee entity by Id public Employee getEmployeeById(int id) { return repository.findById(id).get(); }
EmployeeService
package com.tutorialspoint.service; import java.util.ArrayList; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.tutorialspoint.entity.Employee; import com.tutorialspoint.repository.EmployeeRepository; @Service public class EmployeeService { @Autowired EmployeeRepository repository; public Employee getEmployeeById(int id) { return repository.findById(id).get(); } public List<Employee> getAllEmployees(){ return null; } public void saveOrUpdate(Employee employee) { repository.save(employee); } public void deleteEmployeeById(int id) { } }
Update Controller
// Use service.getEmployeeById() to get Employee entity from database @GetMapping("/employee/{id}") public Employee getEmployee(@PathVariable("id") int id) { return employeeService.getEmployeeById(id); }
EmployeeController
package com.tutorialspoint.controller; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.DeleteMapping; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.PutMapping; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import com.tutorialspoint.entity.Employee; import com.tutorialspoint.service.EmployeeService; @RestController @RequestMapping(path = "/emp") public class EmployeeController { @Autowired EmployeeService employeeService; @GetMapping("/employees") public List<Employee> getAllEmployees(){ return null; } @GetMapping("/employee/{id}") public Employee getEmployee(@PathVariable("id") int id) { return employeeService.getEmployeeById(id); } @DeleteMapping("/employee/{id}") public void deleteEmployee(@PathVariable("id") int id) { } @PostMapping("/employee") public void addEmployee(@RequestBody Employee employee) { employeeService.saveOrUpdate(employee); } @PutMapping("/employee") public void updateEmployee(@RequestBody Employee employee) { } }
Run the application
In eclipse, run the Employee Application configuration as prepared during Application Setup
Eclipse console will show the similar output.
[INFO] Scanning for projects... ... 2021-07-24 20:51:14.823 INFO 9760 --- [ restartedMain] c.t.s.SprintBootH2Application : Started SprintBootH2Application in 7.353 seconds (JVM running for 8.397)
Once server is up and running, Use Postman to make a POST request to add a record first.
Set the following parameters in POSTMAN.
HTTP Method − POST
URL − http://localhost:8080/emp/employee
BODY − An employee JSON
{ "id": "1", "age": "35", "name": "Julie", "email": "julie@gmail.com" }
Click on Send Button and check the response status to be OK. Now make a GET Request to get that record.
Set the following parameters in POSTMAN.
HTTP Method − GET
URL − http://localhost:8080/emp/employee/1
Click the send button and verify the response.
{ "id": "1", "age": "35", "name": "Julie", "email": "julie@gmail.com" }
Advertisements