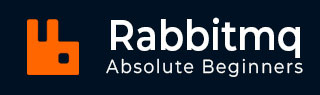
- RabbitMQ Tutorial
- RabbitMQ - Home
- RabbitMQ - Overview
- RabbitMQ - Environment Setup
- RabbitMQ - Features
- RabbitMQ - Installation
- Queue Based Example
- RabbitMQ - Producer Application
- RabbitMQ - Consumer Application
- RabbitMQ - Test Application
- Topic Based Example
- RabbitMQ - Publisher Application
- RabbitMQ - Subscriber Application
- RabbitMQ - Test Application
- RabbitMQ Useful Resources
- RabbitMQ - Quick Guide
- RabbitMQ - Useful Resources
- RabbitMQ - Discussion
RabbitMQ - Consumer Application
Now let's create a consumer application which will receive message from the RabbitMQ Queue.
Create Project
Using eclipse, select File → New → Maven Project. Tick the Create a simple project(skip archetype selection) and click Next.
Enter the details, as shown below:
groupId − com.tutorialspoint
artifactId − consumer
version − 0.0.1-SNAPSHOT
name − RabbitMQ Consumer
Click on Finish button and a new project will be created.
pom.xml
Now update the content of pom.xml to include dependencies for ActiveMQ.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint.activemq</groupId> <artifactId>consumer</artifactId> <version>0.0.1-SNAPSHOT</version> <name>RabbitMQ Consumer</name> <properties> <java.version>11</java.version> </properties> <dependencies> <dependency> <groupId>com.rabbitmq</groupId> <artifactId>amqp-client</artifactId> <version>5.14.2</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.26</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.26</version> </dependency> </dependencies> </project>
Now create a Consumer class which will receive message from the RabbitMQ Queue.
package com.tutorialspoint.rabbitmq; import java.io.IOException; import java.nio.charset.StandardCharsets; import java.util.concurrent.TimeoutException; import com.rabbitmq.client.Channel; import com.rabbitmq.client.Connection; import com.rabbitmq.client.ConnectionFactory; import com.rabbitmq.client.DeliverCallback; public class Consumer { private static String QUEUE = "MyFirstQueue"; public static void main(String[] args) throws IOException, TimeoutException { ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); Connection connection = factory.newConnection(); Channel channel = connection.createChannel(); channel.queueDeclare(QUEUE, false, false, false, null); System.out.println("Waiting for messages. To exit press CTRL+C"); DeliverCallback deliverCallback = (consumerTag, delivery) -> { String message = new String(delivery.getBody(), StandardCharsets.UTF_8); System.out.println("Received '" + message + "'"); }; channel.basicConsume(QUEUE, true, deliverCallback, consumerTag -> { }); } }
Consumer class creates a connection, creates a channel, creates a queue if not existent then receives message from queue if there is any and it will keep polling queue for messages. Once a message is delivered, it is handled by basicConsume() method using deliverCallback.
We'll run this application in RabbitMQ - Test Application chapter.