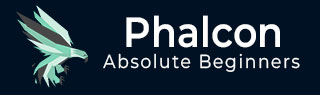
- Phalcon Tutorial
- Phalcon - Home
- Phalcon - Overview
- Phalcon - Environmental Setup
- Phalcon - Application Structure
- Phalcon - Functionality
- Phalcon - Configuration
- Phalcon - Controllers
- Phalcon - Models
- Phalcon - Views
- Phalcon - Routing
- Phalcon - Database Connectivity
- Phalcon - Switching Databases
- Phalcon - Scaffolding Application
- Phalcon - Query Language
- Phalcon - Database Migration
- Phalcon - Cookie Management
- Phalcon - Session Management
- Phalcon - Multi-Lingual Support
- Phalcon - Asset Management
- Phalcon - Working with Forms
- Phalcon - Object Document Mapper
- Phalcon - Security Features
- Phalcon Useful Resources
- Phalcon - Quick Guide
- Phalcon - Useful Resources
- Phalcon - Discussion
Phalcon - Designing the Login Page
UsersController.php
<?php class UsersController extends Phalcon\Mvc\Controller { public function indexAction() { } public function loginAction() { if ($this->request->isPost()) { $user = Users::findFirst(array( 'login = :login: and password = :password:', 'bind' => array( 'login' => $this->request->getPost("login"), 'password' => $this->request->getPost("password") ) )); if ($user === false) { $this->flash->error("Incorrect credentials"); return $this->dispatcher->forward(array( 'controller' => 'users', 'action' => 'index' )); } $this->session->set('auth', $user->id); $this->flash->success("You've been successfully logged in"); } return $this->dispatcher->forward(array( 'controller' => 'posts', 'action' => 'index' )); } public function logoutAction() { $this->session->remove('auth'); return $this->dispatcher->forward(array( 'controller' => 'posts', 'action' => 'index' )); } }
The UsersController includes functionality with log in and log out features. It checks for the associated value in the records for “Users” table. If the value gets authenticated, the user successfully logs in or else gets an error message.
Following is the output of the above code.

Once logged in to the web application, the output will look as shown in the following screenshot.
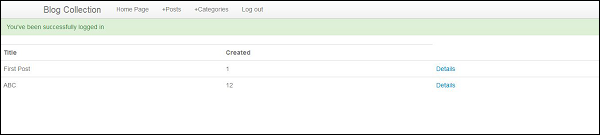
We will look at implementing views in the next chapter which will focus on categories and posts management.
phalcon_scaffolding_application.htm
Advertisements
To Continue Learning Please Login