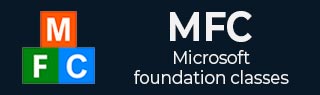
- MFC Tutorial
- MFC - Home
- MFC - Overview
- MFC - Environment Setup
- MFC - VC++ Projects
- MFC - Getting Started
- MFC - Windows Fundamentals
- MFC - Dialog Boxes
- MFC - Windows Resources
- MFC - Property Sheets
- MFC - Windows Layout
- MFC - Controls Management
- MFC - Windows Controls
- MFC - Messages & Events
- MFC - Activex Controls
- MFC - File System
- MFC - Standard I/O
- MFC - Document View
- MFC - Strings
- MFC - Carray
- MFC - Linked Lists
- MFC - Database Classes
- MFC - Serialization
- MFC - Multithreading
- MFC - Internet Programming
- MFC - GDI
- MFC - Libraries
- MFC Useful Resources
- MFC - Quick Guide
- MFC - Useful Resources
- MFC - Discussion
MFC - Slider Controls
A Slider Control (also known as a trackbar) is a window containing a slider and optional tick marks. When the user moves the slider, using either the mouse or the direction keys, the control sends notification messages to indicate the change. There are two types of sliders — horizontal and vertical. It is represented by CSliderCtrl class.
Let us look into a simple example by creating a new MFC dialog based project.
Step 1 − Once the project is created you will see the TODO line which is the Caption of Text Control. Remove the Caption and set its ID to IDC_STATIC_TXT.
Step 2 − Add a value variable m_strSliderVal for the Static Text control.
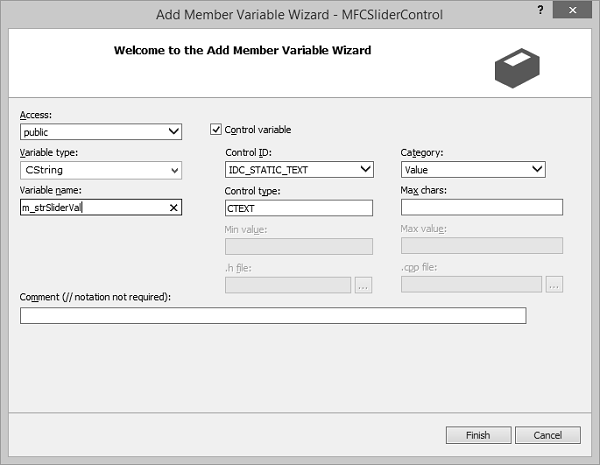
Step 3 − Drag the slider control from the Toolbox.
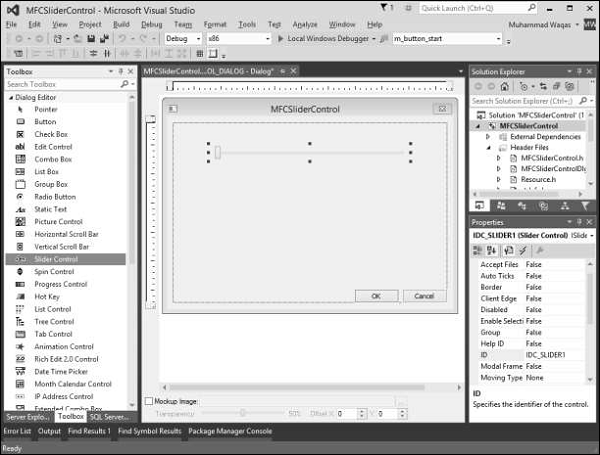
Step 4 − Add a control variable m_sliderCtrl for slider.

Step 5 − Go to the class view in solution.
Step 6 − Select the CMFCSliderControlDlg class.
Step 7 − In the Properties window, click Messages.
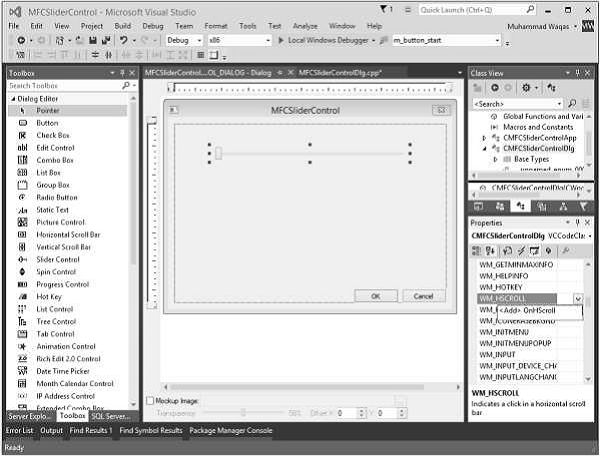
Step 8 − Scroll down to "WM_HSCROLL" and click on the drop-down menu. Click "<Add> OnHScroll".
Step 9 − Initialize the Slider and Static Text control inside the OnInitDialog() function.
BOOL CMFCSliderControlDlg::OnInitDialog() { CDialogEx::OnInitDialog(); // Set the icon for this dialog. The framework does this automatically // when the application's main window is not a dialog SetIcon(m_hIcon, TRUE); // Set big icon SetIcon(m_hIcon, FALSE); // Set small icon // TODO: Add extra initialization here m_sliderCtrl.SetRange(0, 100, TRUE); m_sliderCtrl.SetPos(0); m_strSliderVal.Format(_T("%d"), 0); return TRUE; // return TRUE unless you set the focus to a control }
Step 10 − Add the following code inside the function code block for OnVScroll()
void CMFCSliderControlDlg::OnHScroll(UINT nSBCode, UINT nPos, CScrollBar* pScrollBar) { // TODO: Add your message handler code here and/or call default if (pScrollBar == (CScrollBar *)&m_sliderCtrl) { int value = m_sliderCtrl.GetPos(); m_strSliderVal.Format(_T("%d"), value); UpdateData(FALSE); }else { CDialog::OnHScroll(nSBCode, nPos, pScrollBar); } }
Step 11 − When the above code is compiled and executed, you will see the following output.
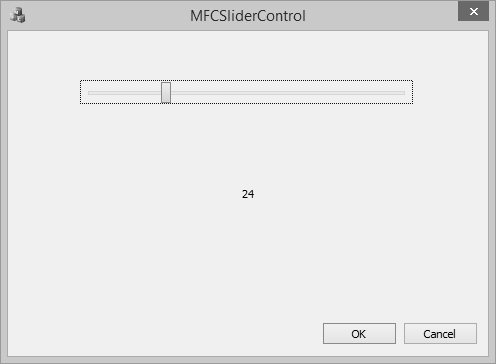