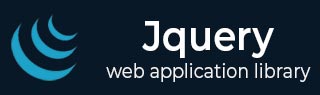
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - Traversing Ancestors
jQuery provides methods to traverse upwards inside the DOM tree to find ancestor(s) of a given element. These methods can be used to find a parent, grandparent, great-grandparent, and so on for a given element inside the DOM.
There are following three methods to traverse upward inside the DOM tree:
parent() - returns the direct parent of the each matched element.
parents() - returns all the ancestor elements of the matched element.
parentsUntil() - returns all ancestor elements until it finds the element given as selector argument.
jQuery parent() Method
The jQuery parent() method returns the direct parent of the each matched element. Following is a simple syntax of the method:
$(selector).parent([filter])
We can optionally provide a filter selector in the method. If the filter is supplied, the elements will be filtered by testing whether they match it.
Synopsis
Consider the following HTML content:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Now if we use the parent() method as follows:
$( ".child-two" ).parent().css( "border", "2px solid red" );
It will produce following result:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Example
Let's try the following example and verify the result:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parent().css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parent</button> </body> </html>
You can try the same example by creating another block of parent and child elements with the same classes and then verify that parent() will apply given CSS to all the matched elements.
jQuery parents() Method
The jQuery parents() method returns all the ancestor elements of the matched element. Following is a simple syntax of the method:
$(selector).parents([filter])
We can optionally provide a filter selector in the method. If the filter is supplied, the elements will be filtered by testing whether they match it.
The parents() and parent() methods are similar, except that the parent() method only travels a single level up the DOM tree. Also, $( "html" ).parent() method returns a set containing document whereas $( "html" ).parents() returns an empty set.
Synopsis
Consider the following HTML content:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Now if we use the parents() method as follows:
$( ".child-two" ).parents().css( "border", "2px solid red" );
It will produce following result:
<div class="great-grand-parent" style="border:2px solid red"> <div class="grand-parent" style="border:2px solid red"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Example
Let's try the following example and verify the result. Here we are going to filter only <div> elements for clarity purpose:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parents("div").css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div style="width:525px;" class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parents</button> </body> </html>
jQuery parentsUntil() Method
The jQuery parentsUntil() method returns all the ancestor elements available between two selectors. Following is a simple syntax of the method:
$(selector1).parentsUntil([selector2][,filter])
We can optionally provide a filter selector in the method. If the filter is supplied, the elements will be filtered by testing whether they match it.
Synopsis
Consider the following HTML content:
<div class="great-grand-parent"> <div class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Now if we use the parentsUntil() method as follows:
$( ".child-two" ).parentsUntil(".great-grand-parent").css( "border", "2px solid red" );
It will produce following result:
<div class="great-grand-parent"> <div class="grand-parent" style="border:2px solid red"> <ul class="parent" style="border:2px solid red"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div>
Example
Let's try the following example and verify the result:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $( ".child-two" ).parentsUntil(".great-grand-parent").css( "border", "2px solid red" ); }); }); </script> <style> .great-grand-parent, .great-grand-parent *{display:block; border:2px solid #aaa; color:#aaa; padding:5px; margin:5px;} </style> </head> <body> <div style="width:525px;" class="great-grand-parent"> <div style="width:500px;" class="grand-parent"> <ul class="parent"> <li class="child-one">Child One</li> <li class="child-two">Child Two</li> </ul> </div> </div> <br> <button>Mark Parents</button> </body> </html>
jQuery Traversing Reference
You can get a complete reference of all the jQuery Methods to traverse the DOM at the following page: jQuery Traversing Reference.