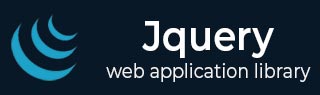
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - Attributes Manipulation
jQuery is being heavily used in manipulating various attributes associated with HTML elements. Every HTML element can have various standard and custom attributes (i.e. properties) which are used to define the characteristics of that HTML element.
jQuery gives us the means to easily manipulate (Get and Set) an element's attributes. First let's try to understand a little about HTML standard and custom attributes.
Standard Attributes
Some of the more common attributes are −
- className
- tagName
- id
- href
- title
- rel
- src
- style
Example
Let's have a look at the following code snippet for HTML markup for an image element −
<img id="id" src="image.gif" alt="Image" class="class" title="This is an image"/>
In this element's markup, the tag name is img, and the markup for id, src, alt, class, and title represents the element's attributes, each of which consists of a name and a value.
Custom data-* Attributes
HTML specification allows us to add our own custom attributes with the DOM elements to provide additional detail about the element. These attributes names start with data-.
Example
Below is an example where we provided information about copyright of the image using data-copyright which is a custom attribute −
<img data-copyright="Tutorials Point" id="imageid" src="image.gif" alt="Image"/>
jQuery - Get Standard Attributes
jQuery attr() method is used to fetch the value of any standard attribute from the matched HTML element(s). We will use jQuery Selectors to match the desired element(s) and then we will apply attr() method to get the attribute value for the element.
If given selector matches more than one elements then it returns the list of values which you can iterate through using jQuery Array methods.
Example
Following is a jQuery program to get href and title attributes of an anchor <a> element:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ alert( "Href = " + $("#home").attr("href")); alert( "Title = " + $("#home").attr("title")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <p><a id="home" href="index.htm" title="Tutorials Point">Home</a></p> <button>Get Attribute</button> </body> </html>
jQuery - Get Data Attributes
jQuery data() method is used to fetch the value of any custom data attribute from the matched HTML element(s). We will use jQuery Selectors to match the desired element(s) and then we will apply data() method to get the attribute value for the element.
Example
Following is a jQuery program to get author-name and year attributes of a <div> element:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ alert( "Author = " + $("#home").data("author-name")); alert( "Year = " + $("#home").data("year")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <div id="home" data-author-name="Zara Ali" data-year="2022"> Just an Example Content </div> <br> <button>Get Attribute</button> </body> </html>
jQuery - Set Standard Attributes
jQuery attr(name, value) method is used to set the value of any standard attribute of the matched HTML element(s). We will use jQuery Selectors to match the desired element(s) and then we will apply attr(key, value) method to set the attribute value for the element.
If given selector matches more than one elements then it will set the value of the attribute for all the matched elements.
Example
Following is a jQuery program to set the title attribute of an anchor <a> element:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("#home").attr("title", "New Anchor Title"); /* Let's get and display changed title */ alert( "Changed Title = " + $("#home").attr("title")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <p><a id="home" href="index.htm" title="Tutorials Point">Home</a></p> <button>Set Attribute</button> <p>You can hover the Home link to verify the title before and after the change.</p> </body> </html>
jQuery - Set Custom Attributes
jQuery data(name, value) method is used to set the value of any custom attribute of the matched HTML element(s). We will use jQuery Selectors to match the desired element(s) and then we will apply attr(key, value) method to set the attribute value for the element.
If given selector matches more than one elements then it will set the value of the attribute for all the matched elements.
Example
Following is a jQuery program to set author-name attribute of a <div> element:
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("button").click(function(){ $("#home").data("author-name", "Nuha Ali"); /* Let's get and display changed author name */ alert( "Changed Name = " + $("#home").data("author-name")); }); }); </script> </head> <body> <p>Click the below button to see the result:</p> <div id="home" data-author-name="Zara Ali" data-year="2022"> Just an Example Content </div> <br> <button>Set Attribute</button> </body> </html>
jQuery HTML/CSS Reference
You can get a complete reference of all the jQuery Methods to manipulate HTML and CSS content at the following page: jQuery HTML/CSS Reference.