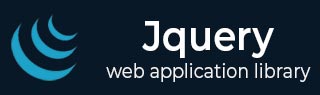
- jQuery Tutorial
- jQuery - Home
- jQuery - Overview
- jQuery - Basics
- jQuery - Syntax
- jQuery - Selectors
- jQuery - Events
- jQuery - Attributes
- jQuery - AJAX
- jQuery DOM Manipulation
- jQuery - DOM
- jQuery - Add Elements
- jQuery - Remove Elements
- jQuery - Replace Elements
- jQuery CSS Manipulation
- jQuery - CSS Classes
- jQuery - Dimensions
- jQuery - CSS Properties
- jQuery Traversing
- jQuery - Traversing
- jQuery - Traversing Ancestors
- jQuery - Traversing Descendants
- jQuery References
- jQuery - Utilities
- jQuery Plugins
- jQuery - Plugins
- jQuery - PagePiling.js
- jQuery - Flickerplate.js
- jQuery - Multiscroll.js
- jQuery - Slidebar.js
- jQuery - Rowgrid.js
- jQuery - Alertify.js
- jQuery - Progressbar.js
- jQuery - Slideshow.js
- jQuery - Drawsvg.js
- jQuery - Tagsort.js
- jQuery - LogosDistort.js
- jQuery - Filer.js
- jQuery - Whatsnearby.js
- jQuery - Checkout.js
- jQuery - Blockrain.js
- jQuery - Producttour.js
- jQuery - Megadropdown.js
- jQuery - Weather.js
- jQuery Useful Resources
- jQuery - Questions and Answers
- jQuery - Quick Guide
- jQuery - Useful Resources
- jQuery - Discussion
jQuery - Effects
jQuery effects add an X factor to your website interactivity. jQuery provides a trivially simple interface for doing various kind of amazing effects like show, hide, fade-in, fade-out, slide-up, slide-down, toggle etc. jQuery methods allow us to quickly apply commonly used effects with a minimum configuration. This tutorial covers all the important jQuery methods to create visual effects.
jQuery Effect - Hiding Elements
jQuery gives simple syntax to hide an element with the help of hide() method:
$(selector).hide( [speed, callback] );
You can apply any jQuery selector to select any DOM element and then apply jQuery hide() method to hide it. Here is the description of all the parameters which gives you a solid control over the hiding effect −
speed − This optional parameter represents one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
The default speed duration 'normal' is 400 milliseconds. The strings 'fast' and 'slow' can be supplied to indicate durations of 200 and 600 milliseconds, respectively. Higher values indicate slower animations.
Example
Following is an example where a <div> will hide itself when we click over it. We have used 1000 as speed parameter which means it will take 1 second to apply the hide effect on the clicked element.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("div").click(function(){ $(this).hide(1000); }); }); </script> <style> div{ margin:10px;padding:12px; border:2px solid #666; width:60px; cursor:pointer} </style> </head> <body> <p>Click on any of the squares to see the result:</p> <div>Hide Me</div> <div>Hide Me</div> <div>Hide Me</div> </body> </html>
jQuery Effect - Show Elements
jQuery gives simple syntax to show a hidden element with the help of show() method:
$(selector).show( [speed, callback] );
You can apply any jQuery selector to select any DOM element and then apply jQuery show() method to show it. Here is the description of all the parameters which gives you a control over the show effect −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Box with the help of two buttons. We will use these two buttons to show and hide this Box. We have used different speeds for the two effects hide(5000) and show(1000) to show the difference in effect speed.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#show").click(function(){ $("#box").show(1000); }); $("#hide").click(function(){ $("#box").hide(5000); }); }); </script> <style> button{cursor:pointer;} #box{margin-bottom:5px;padding:12px;height:100px; width:125px; background-color:#9c9cff;} </style> </head> <body> <p>Click on Show and Hide buttons to see the result:</p> <div id="box">This is Box</div> <button id="hide">Hide Box</button> <button id="show">Show Box</button> </body> </html>
jQuery Effect - Toggle Elements
jQuery provides toggle() methods to toggle the display state of elements between revealed or hidden. If the element is initially displayed, it will be hidden; if hidden, it will be shown.
$(selector).toggle( [speed, callback] );
You can apply any jQuery selector to select any DOM element and then apply jQuery toggle() method to toggle it. Here is the description of all the parameters which gives you a solid control over the toggle effect −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Square Box with the help of a single Toggle button. When we click this button for the first time, square box becomes invisible, and next time when we click the button then square box becomes visible. We have used 1000 as speed parameter which means it will take 1 second to apply the toggle effect.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#button").click(function(){ $("#box").toggle(1000); }); }); </script> <style> button{margin:3px;width:125px;cursor:pointer;} #box{margin:3px;padding:12px; height:100px; width:100px;background-color:#9c9cff;} </style> </head> <body> <p>Click on the Toggle Box button to see the box toggling:</p> <div id="box">This is Box</div> <button id="button">Toggle Box</button> </body> </html>
jQuery Effect - Fading Elements
jQuery gives us two methods - fadeIn() and fadeOut() to fade the DOM elements in and out of visibility.
$(selector).fadeIn( [speed, callback] ); $(selector).fadeOut( [speed, callback] );
The jQuery fadeIn() method is used to fade in a hidden element where as fadeOut() method is used to fade out a visible element. Here is the description of all the parameters which gives you a control over the fading effects −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Box with the help of two buttons. We will use these two buttons to show and hide this Box. We have used 1000 as speed parameter which means it will take 1 second to apply the effect.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#show").click(function(){ $("#box").fadeIn(1000); }); $("#hide").click(function(){ $("#box").fadeOut(1000); }); }); </script> <style> button{cursor:pointer;} #box{margin-bottom:5px;padding:12px;height:100px; width:150px; background-color:#9c9cff;} </style> </head> <body> <p>Click on fadeOut and fadeIn buttons to see the result:</p> <div id="box">This is Box</div> <button id="hide">fadeOut Box</button> <button id="show">fadeIn Box</button> </body> </html>
jQuery Effect - Toggle with Fading
jQuery provides fadeToggle() methods to toggle the display state of elements between the fadeIn() and fadeOut() methods. If the element is initially displayed, it will be hidden (ie. fadeOut()); if hidden, it will be shown (ie. fadeIn()).
$(selector).fadeToggle( [speed, callback] );
This method gives the same functionality what we can have using toggle() method, but additionally, it gives fade in and fade out effect while toggling the element.
Here is the description of all the parameters which gives you more control over the effect −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Square Box with the help of a single button. When we click this button for the first time, square box fades out (hidden), and next time when we click the button then square box fades in (visible). We have used 1000 as speed parameter which means it will take 1 second to apply the toggle effect.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#button").click(function(){ $("#box").fadeToggle(1000); }); }); </script> <style> button{margin:3px;width:125px;cursor:pointer;} #box{margin:3px;padding:12px; height:100px; width:100px;background-color:#9c9cff;} </style> </head> <body> <p>Click on the Toggle Box button to see the box toggling:</p> <div id="box">This is Box</div> <button id="button">Toggle Box</button> </body> </html>
Try using jQuery $(selector).toggle() and $(selector).fadeToggle() methods to understand the minor difference between these two methods.
jQuery Effect - Sliding Elements
jQuery gives us two methods - slideUp() and slideDown() to slide up and slide down the DOM elements respectively. Following is the simple syntax for these two methods:
$(selector).slideUp( [speed, callback] ); $(selector).slideDown( speed, [callback] );
The jQuery slideUp() method is used to slide up an element where as slideDown() method is used to slide down. Here is the description of all the parameters which gives you more control over the effects −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Box with the help of two buttons. We will use these two buttons to show and hide this Box. We have used 1000 as speed parameter which means it will take 1 second to apply the toggle effect.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#show").click(function(){ $("#box").slideDown(1000); }); $("#hide").click(function(){ $("#box").slideUp(1000); }); }); </script> <style> button{cursor:pointer;} #box{margin-bottom:5px;padding:12px;height:100px; width:120px; background-color:#9c9cff;} </style> </head> <body> <p>Click on slideUp and slideDown buttons to see the result:</p> <div id="box">This is Box</div> <button id="hide">slideUp </button> <button id="show">slideDown </button> </body> </html>
jQuery Effect - Toggle with Sliding
jQuery provides slideToggle() methods to toggle the display state of elements between the slideUp() and slideDown() methods. If the element is initially displayed, it will be hidden (ie. slideUp()); if hidden, it will be shown (ie. slideDown()).
$(selector).slideToggle( [speed, callback] );
This method gives the same functionality what we can have using toggle() method, but additionally, it gives slide up and slide down effect while toggling the element.
Here is the description of all the parameters which gives you more control over the effect −
speed − An optional string representing one of the three predefined speeds ("slow", "normal", or "fast") or the number of milliseconds to run the animation (e.g. 1000).
callback − This optional parameter represents a function to be executed whenever the animation completes; executes once for each element animated against.
Example
Following is an example where we will play with a Square Box with the help of a single button. When we click this button for the first time, square box fades out (hidden), and next time when we click the button then square box fades in (visible). We have used 1000 as speed parameter which means it will take 1 second to apply the toggle effect.
<!doctype html> <html> <head> <title>The jQuery Example</title> <script src="https://www.tutorialspoint.com/jquery/jquery-3.6.0.js"></script> <script> $(document).ready(function() { $("#button").click(function(){ $("#box").slideToggle(1000); }); }); </script> <style> button{margin:3px;width:125px;cursor:pointer;} #box{margin:3px;padding:12px; height:100px; width:100px;background-color:#9c9cff;} </style> </head> <body> <p>Click on the Toggle Box button to see the box toggling:</p> <div id="box">This is Box</div> <button id="button">Toggle Box</button> </body> </html>
Try using jQuery $(selector).toggle(), $(selector).slideToggle() and $(selector).fadeToggle() methods to understand the minor difference among these three methods.
jQuery Effects Reference
This tutorial covered only a few most frequently used jQuery effects, You can get a complete reference of all the jQuery Effect Methods at the following page: jQuery Effects Reference.