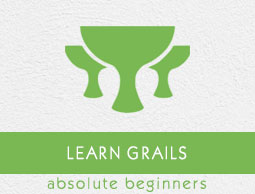
- Grails Tutorial
- Grails - Home
- Grails - Overview
- Grails - MVC Architecture
- Grails - Creating an Application
- Grails - The Command Line
- Grails - Application Profiles
- Object Relational Mapping (GORM)
- Grails - The Web Layer
- Grails - Groovy Server Pages
- Grails - Web Services
- Grails - Validation
- Grails - Plugins
Grails - Validating Constraints
Description
The constraints can be validated by using the validate() method on domain class instance.
For instance:
def info = new UserInfo(params) if (info.validate()) { //code here } else { info.errors.allErrors.each { println it } }
The errors property is an instance of the Spring Errors interface on domain classes which displays the validation errors by using methods and fetches the original values.
Validation Phases
Grails includes two types of validation phases. The first phase can be used in the data binding process in which request parameters can be bind on to an instance as shown below:
def info = new UserInfo(params)
If you get any errors in the errors property, then you can check these errors and get the actual input values by using the Errors API:
if (info.hasErrors()) { if (info.errors.hasFieldErrors("fname")) { println info.errors.getFieldError("fname").rejectedValue } }
When you call the validate() or save() method, the second phase of validation takes place, when you validate the bound values opposed to defined constraints.
For instance:
By default, validate will be called by the save() method before executing as written in the below code:
if (info.save()) { return info } else { info.errors.allErrors.each { println it } }
To Continue Learning Please Login