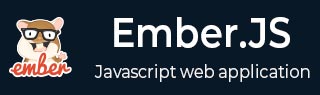
- EmberJS Tutorial
- EmberJS - Home
- EmberJS - Overview
- EmberJS - Installation
- EmberJS - Core Concepts
- Creating and Running Application
- EmberJS - Object Model
- EmberJS - Router
- EmberJS - Templates
- EmberJS - Components
- EmberJS - Models
- EmberJS - Managing Dependencies
- EmberJS - Application Concerns
- EmberJS - Configuring Ember.js
- EmberJS - Ember Inspector
- EmberJS Useful Resources
- EmberJS - Quick Guide
- EmberJS - Useful Resources
- EmberJS - Discussion
EmberJS - Pushing Records
You can push the records into the store's cache without requesting the records from an application. The store has the ability to return the record, if it is asked by a route or controller only when record is in the cache.
Example
The example given below shows the pushing of records into the ember firebase. Open the application.hbs file created under app/templates/ with the following code −
<h2>Pushing Record into Store</h2> <form> {{input type = "name" value = nameAddress placeholder = "Enter the text" autofocus = "autofocus"}} //when user clicks the send button, the 'saveInvitation' action will get triggered <button {{action 'saveInvitation'}} >Send</button> </form> {{#if responseMessage}} //display the response sessage after sending the text successfully {{responseMessage}} {{/if}} {{outlet}}
Create a model with the name invitation, which will get created under app/models/. Open the file and include the following code −
import DS from 'ember-data'; export default DS.Model.extend ({ //specifying attribute using 'attr()' method name: DS.attr('string') });
Next, create a controller with the name application, which will be created under app/controllers/. Open the file and add the following code −
import Ember from 'ember'; export default Ember.Controller.extend ({ headerMessage: 'Coming Soon', //displays the response message after sending record to store responseMessage: '', nameAddress: '', actions: { //this action name which fires when user clicks send button saveInvitation() { const name = this.get('nameAddress'); //create the records on the store by calling createRecord() method const newInvitation = this.store.createRecord('invitation', { name: name }); newInvitation.save(); //call the save() method to persist the record to the backend this.set('responseMessage', `Thank you! We have saved your Name: ${this.get('nameAddress')}`); this.set('nameAddress', ''); } } });
You can store the information in JSON format on Ember Firebase. To do this, you need to create account by using the Firebase's website. For more information about how to create and configure the Firebase in your application, click this link.
Output
Run the ember server and you will get the input box to enter the value as shown in the screenshot below −
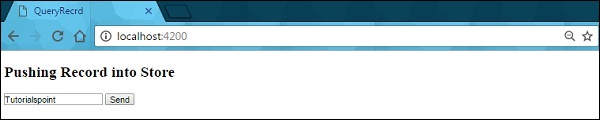
After clicking the send button, it will display the text entered by the user −
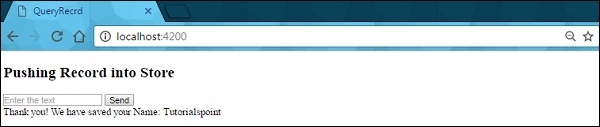
Now open your firebase database, you will see the stored value under the Database section −
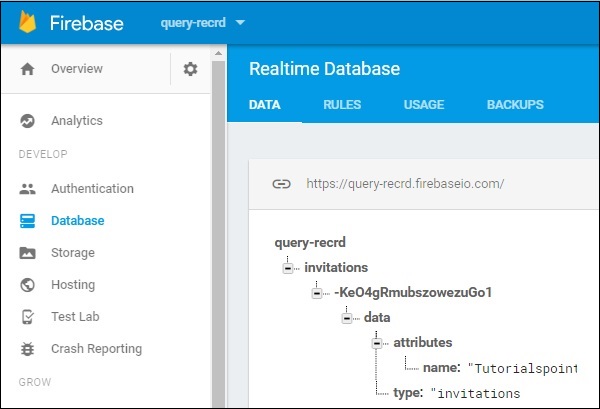