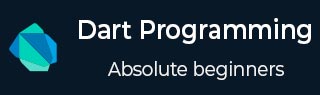
- Dart Programming Tutorial
- Dart Programming - Home
- Dart Programming - Overview
- Dart Programming - Environment
- Dart Programming - Syntax
- Dart Programming - Data Types
- Dart Programming - Variables
- Dart Programming - Operators
- Dart Programming - Loops
- Dart Programming - Decision Making
- Dart Programming - Numbers
- Dart Programming - String
- Dart Programming - Boolean
- Dart Programming - Lists
- Dart Programming - Lists
- Dart Programming - Map
- Dart Programming - Symbol
- Dart Programming - Runes
- Dart Programming - Enumeration
- Dart Programming - Functions
- Dart Programming - Interfaces
- Dart Programming - Classes
- Dart Programming - Object
- Dart Programming - Collection
- Dart Programming - Generics
- Dart Programming - Packages
- Dart Programming - Exceptions
- Dart Programming - Debugging
- Dart Programming - Typedef
- Dart Programming - Libraries
- Dart Programming - Async
- Dart Programming - Concurrency
- Dart Programming - Unit Testing
- Dart Programming - HTML DOM
- Dart Programming Useful Resources
- Dart Programming - Quick Guide
- Dart Programming - Resources
- Dart Programming - Discussion
Dart Programming - Enumeration
An enumeration is used for defining named constant values. An enumerated type is declared using the enum keyword.
Syntax
enum enum_name { enumeration list }
Where,
- The enum_name specifies the enumeration type name
- The enumeration list is a comma-separated list of identifiers
Each of the symbols in the enumeration list stands for an integer value, one greater than the symbol that precedes it. By default, the value of the first enumeration symbol is 0.
For example
enum Status { none, running, stopped, paused }
Example
enum Status { none, running, stopped, paused } void main() { print(Status.values); Status.values.forEach((v) => print('value: $v, index: ${v.index}')); print('running: ${Status.running}, ${Status.running.index}'); print('running index: ${Status.values[1]}'); }
It will produce the following output −
[Status.none, Status.running, Status.stopped, Status.paused] value: Status.none, index: 0 value: Status.running, index: 1 value: Status.stopped, index: 2 value: Status.paused, index: 3 running: Status.running, 1 running index: Status.running
Advertisements