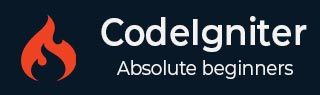
- CodeIgniter Tutorial
- CodeIgniter - Home
- CodeIgniter - Overview
- CodeIgniter - Installing CodeIgniter
- CodeIgniter - Application Architecture
- CodeIgniter - MVC Framework
- CodeIgniter - Basic Concepts
- CodeIgniter - Configuration
- CodeIgniter - Working with Database
- CodeIgniter - Libraries
- CodeIgniter - Error Handling
- CodeIgniter - File Uploading
- CodeIgniter - Sending Email
- CodeIgniter - Form Validation
- CodeIgniter - Session Management
- CodeIgniter - Flashdata
- CodeIgniter - Tempdata
- CodeIgniter - Cookie Management
- CodeIgniter - Common Functions
- CodeIgniter - Page Caching
- CodeIgniter - Page Redirection
- CodeIgniter - Application Profiling
- CodeIgniter - Benchmarking
- CodeIgniter - Adding JS and CSS
- CodeIgniter - Internationalization
- CodeIgniter - Security
- CodeIgniter Useful Resources
- CodeIgniter - Quick Guide
- CodeIgniter - Useful Resources
- CodeIgniter - Discussion
CodeIgniter - Security
XSS Prevention
XSS means cross-site scripting. CodeIgniter comes with XSS filtering security. This filter will prevent any malicious JavaScript code or any other code that attempts to hijack cookie and do malicious activities. To filter data through the XSS filter, use the xss_clean() method as shown below.
$data = $this->security->xss_clean($data);
You should use this function only when you are submitting data. The optional second Boolean parameter can also be used to check image file for XSS attack. This is useful for file upload facility. If its value is true, means image is safe and not otherwise.
SQL Injection Prevention
SQL injection is an attack made on database query. In PHP, we are use mysql_real_escape_string() function to prevent this along with other techniques but CodeIgniter provides inbuilt functions and libraries to prevent this.
We can prevent SQL Injection in CodeIgniter in the following three ways −
- Escaping Queries
- Query Biding
- Active Record Class
Escaping Queries
<?php $username = $this->input->post('username'); $query = 'SELECT * FROM subscribers_tbl WHERE user_name = '. $this->db->escape($email); $this->db->query($query); ?>
$this->db->escape() function automatically adds single quotes around the data and determines the data type so that it can escape only string data.
Query Biding
<?php $sql = "SELECT * FROM some_table WHERE id = ? AND status = ? AND author = ?"; $this->db->query($sql, array(3, 'live', 'Rick')); ?>
In the above example, the question mark(?) will be replaced by the array in the second parameter of query() function. The main advantage of building query this way is that the values are automatically escaped which produce safe queries. CodeIgniter engine does it for you automatically, so you do not have to remember it.
Active Record Class
<?php $this->db->get_where('subscribers_tbl',array ('status'=> active','email' => 'info@arjun.net.in')); ?>
Using active records, query syntax is generated by each database adapter. It also allows safer queries, since the values escape automatically.
Hiding PHP Errors
In production environment, we often do not want to display any error message to the users. It is good if it is enabled in the development environment for debugging purposes. These error messages may contain some information, which we should not show to the site users for security reasons.
There are three CodeIgniter files related with errors.
PHP Error Reporting Level
Different environment requires different levels of error reporting. By default, development will show errors but testing and live will hide them. There is a file called index.php in root directory of CodeIgniter, which is used for this purpose. If we pass zero as argument to error_reporting() function then that will hide all the errors.
Database Error
Even if you have turned off the PHP errors, MySQL errors are still open. You can turn this off in application/config/database.php. Set the db_debug option in $db array to FALSE as shown below.
$db['default']['db_debug'] = FALSE;
Error log
Another way is to transfer the errors to log files. So, it will not be displayed to users on the site. Simply, set the log_threshold value in $config array to 1 in application/cofig/config.php file as shown below.
$config['log_threshold'] = 1;
CSRF Prevention
CSRF stands for cross-site request forgery. You can prevent this attack by enabling it in the application/config/config.php file as shown below.
$config['csrf_protection'] = TRUE;
When you are creating form using form_open() function, it will automatically insert a CSRF as hidden field. You can also manually add the CSRF using the get_csrf_token_name() and get_csrf_hash() function. The get_csrf_token_name() function will return the name of the CSRF and get_csrf_hash() will return the hash value of CSRF.
The CSRF token can be regenerated every time for submission or you can also keep it same throughout the life of CSRF cookie. By setting the value TRUE, in config array with key ‘csrf_regenerate’ will regenerate token as shown below.
$config['csrf_regenerate'] = TRUE;
You can also whitelist URLs from CSRF protection by setting it in the config array using the key ‘csrf_exclude_uris’ as shown below. You can also use regular expression.
$config['csrf_exclude_uris'] = array('api/person/add');
Password Handling
Many developers do not know how to handle password in web applications, which is probably why numerous hackers find it so easy to break into the systems. One should keep in mind the following points while handling passwords −
DO NOT store passwords in plain-text format.
Always hash your passwords.
DO NOT use Base64 or similar encoding for storing passwords.
DO NOT use weak or broken hashing algorithms like MD5 or SHA1. Only use strong password hashing algorithms like BCrypt, which is used in PHP’s own Password Hashing functions.
DO NOT ever display or send a password in plain-text format.
DO NOT put unnecessary limits on your users’ passwords.