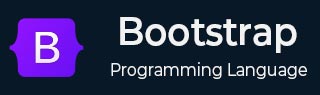
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Tab Plugin
Tabs were introduced in the chapter Bootstrap Navigation Elements. By combining a few data attributes, you can easily create a tabbed interface. With this plug-in you can transition through panes of local content in tabs or pills, even via drop down menus.
If you want to include this plugin functionality individually, then you will need tab.js. Else, as mentioned in the chapter Bootstrap Plugins Overview, you can include bootstrap.js or the minified bootstrap.min.js.
Usage
You can enable tabs in the following two ways −
Via data attributes − you need to add data-toggle = "tab" or data-toggle = "pill" to the anchors.
Adding the nav and nav-tabs classes to the tab ul will apply the Bootstrap tab styling, while adding the nav and nav-pills classes will apply pill styling.
<ul class = "nav nav-tabs"> <li><a href = "#identifier" data-toggle = "tab">Home</a></li> ... </ul>
Via JavaScript − you can enable tabs using Javscript as below −
$('#myTab a').click(function (e) { e.preventDefault() $(this).tab('show') })
Here’s an example of different ways to activate individual tabs −
// Select tab by name $('#myTab a[href = "#profile"]').tab('show') // Select first tab $('#myTab a:first').tab('show') // Select last tab $('#myTab a:last').tab('show') // Select third tab (0-indexed) $('#myTab li:eq(2) a').tab('show')
Fade Effect
To get a fade effect for tabs, add .fade to each .tab-pane. The first tab pane must also have .in to properly fade in initial content −
<div class = "tab-content"> <div class = "tab-pane fade in active" id = "home">...</div> <div class = "tab-pane fade" id = "svn">...</div> <div class = "tab-pane fade" id = "ios">...</div> <div class = "tab-pane fade" id = "java">...</div> </div>
Example
An example of tab plugin using data attributes and fade effect is as shown in the following example −
<ul id = "myTab" class = "nav nav-tabs"> <li class = "active"> <a href = "#home" data-toggle = "tab"> Tutorial Point Home </a> </li> <li><a href = "#ios" data-toggle = "tab">iOS</a></li> <li class = "dropdown"> <a href = "#" id = "myTabDrop1" class = "dropdown-toggle" data-toggle = "dropdown"> Java <b class = "caret"></b> </a> <ul class = "dropdown-menu" role = "menu" aria-labelledby = "myTabDrop1"> <li><a href = "#jmeter" tabindex = "-1" data-toggle = "tab">jmeter</a></li> <li><a href = "#ejb" tabindex = "-1" data-toggle = "tab">ejb</a></li> </ul> </li> </ul> <div id = "myTabContent" class = "tab-content"> <div class = "tab-pane fade in active" id = "home"> <p>Tutorials Point is a place for beginners in all technical areas. This website covers most of the latest technologies and explains each of the technology with simple examples.</p> </div> <div class = "tab-pane fade" id = "ios"> <p>iOS is a mobile operating system developed and distributed by Apple Inc. Originally released in 2007 for the iPhone, iPod Touch, and Apple TV. iOS is derived from OS X, with which it shares the Darwin foundation. iOS is Apple's mobile version of the OS X operating system used on Apple computers.</p> </div> <div class = "tab-pane fade" id = "jmeter"> <p>jMeter is an Open Source testing software. It is 100% pure Java application for load and performance testing.</p> </div> <div class = "tab-pane fade" id = "ejb"> <p>Enterprise Java Beans (EJB) is a development architecture for building highly scalable and robust enterprise level applications to be deployed on J2EE compliant Application Server such as JBOSS, Web Logic etc.</p> </div> </div>
Methods
.$().tab − This method activates a tab element and content container. Tab should have either a data-target or an href targeting a container node in the DOM.
<ul class = "nav nav-tabs" id = "myTab"> <li class = "active"><a href = "#identifier" data-toggle = "tab">Home</a></li> ..... </ul> <div class = "tab-content"> <div class = "tab-pane active" id = "home">...</div> ..... </div> <script> $(function () { $('#myTab a:last').tab('show') }) </script>
Example
The following example shows the use of tab plugin method .tab. Here in the example the second tab iOS is activated −
<ul id = "myTab" class = "nav nav-tabs"> <li class = "active"> <a href = "#home" data-toggle = "tab"> Tutorial Point Home </a> </li> <li><a href = "#ios" data-toggle = "tab">iOS</a></li> <li class = "dropdown"> <a href = "#" id = "myTabDrop1" class = "dropdown-toggle" data-toggle = "dropdown"> Java <b class = "caret"></b> </a> <ul class = "dropdown-menu" role = "menu" aria-labelledby = "myTabDrop1"> <li> <a href = "#jmeter" tabindex = "-1" data-toggle = "tab"> jmeter </a> </li> <li> <a href = "#ejb" tabindex = "-1" data-toggle = "tab"> ejb </a> </li> </ul> </li> </ul> <div id = "myTabContent" class = "tab-content"> <div class = "tab-pane fade in active" id = "home"> <p>Tutorials Point is a place for beginners in all technical areas. This website covers most of the latest technologies and explains each of the technology with simple examples.</p> </div> <div class = "tab-pane fade" id = "ios"> <p>iOS is a mobile operating system developed and distributed by Apple Inc. Originally released in 2007 for the iPhone, iPod Touch, and Apple TV. iOS is derived from OS X, with which it shares the Darwin foundation. iOS is Apple's mobile version of the OS X operating system used on Apple computers.</p> </div> <div class = "tab-pane fade" id = "jmeter"> <p>jMeter is an Open Source testing software. It is 100% pure Java application for load and performance testing.</p> </div> <div class = "tab-pane fade" id = "ejb"> <p>Enterprise Java Beans (EJB) is a development architecture for building highly scalable and robust enterprise level applications to be deployed on J2EE compliant Application Server such as JBOSS, Web Logic etc.</p> </div> </div> <script> $(function () { $('#myTab li:eq(1) a').tab('show'); }); </script>
Events
Following table lists the events to work with tab plugin. This event may be used to hook into the function.
Event | Description | Example |
---|---|---|
show.bs.tab | This event fires on tab show, but before the new tab has been shown. Use event.target and event.relatedTarget to target the active tab and the previous active tab (if available) respectively. |
$('a[data-toggle = "tab"]').on('show.bs.tab', function (e) { e.target // activated tab e.relatedTarget // previous tab }) |
shown.bs.tab | This event fires on tab show after a tab has been shown. Use event.target and event.relatedTarget to target the active tab and the previous active tab (if available) respectively. |
$('a[data-toggle = "tab"]').on('shown.bs.tab', function (e) { e.target // activated tab e.relatedTarget // previous tab }) |
Example
The following example shows use of tab plugin events. Here in the example we will display the current and previous visited tabs −
<hr> <p class = "active-tab"><strong>Active Tab</strong>: <span></span></p> <p class = "previous-tab"><strong>Previous Tab</strong>: <span></span></p> <hr> <ul id = "myTab" class = "nav nav-tabs"> <li class = "active"> <a href = "#home" data-toggle = "tab"> Tutorial Point Home </a> </li> <li><a href = "#ios" data-toggle = "tab">iOS</a></li> <li class = "dropdown"> <a href = "#" id = "myTabDrop1" class = "dropdown-toggle" data-toggle = "dropdown"> Java <b class = "caret"></b> </a> <ul class = "dropdown-menu" role = "menu" aria-labelledby = "myTabDrop1"> <li> <a href = "#jmeter" tabindex = "-1" data-toggle = "tab">jmeter</a> </li> <li> <a href = "#ejb" tabindex = "-1" data-toggle = "tab">ejb</a> </li> </ul> </li> </ul> <div id = "myTabContent" class = "tab-content"> <div class = "tab-pane fade in active" id = "home"> <p>Tutorials Point is a place for beginners in all technical areas. This website covers most of the latest technologies and explains each of the technology with simple examples.</p> </div> <div class = "tab-pane fade" id = "ios"> <p>iOS is a mobile operating system developed and distributed by Apple Inc. Originally released in 2007 for the iPhone, iPod Touch, and Apple TV. iOS is derived from OS X, with which it shares the Darwin foundation. iOS is Apple's mobile version of the OS X operating system used on Apple computers.</p> </div> <div class = "tab-pane fade" id = "jmeter"> <p>jMeter is an Open Source testing software. It is 100% pure Java application for load and performance testing.</p> </div> <div class = "tab-pane fade" id = "ejb"> <p>Enterprise Java Beans (EJB) is a development architecture for building highly scalable and robust enterprise level applications to be deployed on J2EE compliant Application Server such as JBOSS, Web Logic etc.</p> </div> </div> <script> $(function(){ $('a[data-toggle = "tab"]').on('shown.bs.tab', function (e) { // Get the name of active tab var activeTab = $(e.target).text(); // Get the name of previous tab var previousTab = $(e.relatedTarget).text(); $(".active-tab span").html(activeTab); $(".previous-tab span").html(previousTab); }); }); </script>
To Continue Learning Please Login