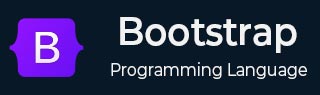
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Form Controls
This chapter will discuss about Bootstrap form controls. Custom styles, size, focus states, and other features can be used to upgrade textual form controls like <input> and <textarea>.
Basic example
Use the classes .form-control and .form-label to style the form controls.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="mb-3"> <label for="formControlName" class="form-label">Full Name</label> <input type="text" class="form-control" id="formControlName" placeholder="name"> </div> <div class="mb-3"> <label for="formControlEmail" class="form-label">Email id</label> <input type="text" class="form-control" id="formControlEmail" placeholder="tutorialspoint@example.com"> </div> <div class="mb-3"> <label for="formControlTextarea" class="form-label">Add a comment</label> <textarea class="form-control" id="formControlTextarea" rows="3"></textarea> </div> <button type="submit" class="btn btn-success">Submit</button> </body> </html>
Sizing
Use classes like .form-control-lg and .form-control-sm to specify the size for the form input field.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <input class="form-control form-control-lg mt-2" type="text" placeholder="Large size input box" aria-label=".form-control-lg example"> <input class="form-control mt-2" type="text" placeholder="Default size input box" aria-label="default input example"> <input class="form-control form-control-sm mt-2" type="text" placeholder="Small size input box" aria-label=".form-control-sm example"> </body> </html>
Form text
Use .form-text to create block-level or inline-level form text .
A top margin is added to easily space the inputs above a block-level element.
Associate form text with form controls using aria-labelledby or aria-describedby attributes to ensure it is announced by assistive technologies such as screen readers.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <label for="inputUsername" class="form-label mt-2">Username</label> <input type="text" id="inputUsername" class="form-control" aria-labelledby="UsernameHelpBlock"> <div id="UsernameHelpBlock" class="form-text"> Your username 6–20 characters long and can be any combination of letters, numbers or symbols. </div> <label for="inputPassword" class="form-label mt-2">Password</label> <input type="text" id="inputPassword" class="form-control" aria-labelledby="PasswordHelpBlock"> <div id="PasswordHelpBlock" class="form-text"> must be 6-20 characters long. </div> <button type="submit" class="btn btn-primary mt-2">Submit</button> </body> </html>
Inline text can use any typical inline HTML element (such as, <span>, <small>...etc) with only .form-text class.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="row g-3 align-items-center mt-2"> <div class="col-auto"> <label for="inputUsername" class="col-form-label">Username</label> </div> <div class="col-auto"> <input type="text" id="inputUsername" class="form-control" aria-labelledby="usernameHelpInline"> </div> <div class="col-auto"> <span id="usernameHelpInline" class="form-text"> Username 6–20 characters long. </span> </div> </div> </body> </html>
Disabled
For a grayed-out appearance and to remove pointer events use the disabled attribute to an input.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <input class="form-control mt-2" type="text" placeholder="Disable Input Field" aria-label="Disabled input example" disabled> </body> </html>
Readonly
Use the readonly attribute to prevent changes to an input's value.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <input class="form-control mt-2" type="text" value="Tutorialspoint" aria-label="readonly example" readonly> </body> </html>
Readonly plain text
.form-control-plaintext creates a readonly input field as a plaintext. This removes styling and preserves the proper margin and padding.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="mb-3 row"> <label for="name" class="col-sm-2 col-form-label">Name</label> <div class="col-sm-3"> <input type="text" readonly class="form-control-plaintext" id="name" value="Tutorialspoint"> </div> </div> <div class="mb-3 row"> <label for="bootstrap" class="col-sm-2 col-form-label">Language</label> <div class="col-sm-3"> <input type="text" class="form-control" id="bootstrap" placeholder="Bootstrap"> </div> </div> </body> </html>
In readonly plaintext inline, you can make form labels and inputs will appear inline and horizontally.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <form class="row g-2 mt-2"> <div class="col-auto"> <input type="text" readonly class="form-control-plaintext" id="staticName" value="Tutorialspoint"> </div> <div class="col-auto"> <input type="text" class="form-control" id="inputLanguage" placeholder="Bootstrap"> </div> </form> </body> </html>
File input
File input is used to browse the file as an input on your computer.
File input using sizes
Use .form-control-sm to make the size of file input smaller.
Use .form-control-lg to make the size of file input bigger.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="mb-3"> <label for="fileInputsm" class="form-label">Small size file input</label> <input class="form-control form-control-sm" id="fileInputsm" type="file"> </div> <div> <label for="fileInputlg" class="form-label">Large size file input</label> <input class="form-control form-control-lg" id="fileInputlg" type="file"> </div> </body> </html>
File input using attribute
There is no need to explicitly define the default attribute.
Use disabled attribute which give grayed-out appearance and remove pointer events.
Multiple files can be accepted for input at once with multiple attribute.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="mb-3"> <label for="defaultFileInput" class="form-label">Default file input</label> <input class="form-control" type="file" id="defaultFileInput"> </div> <div class="mb-3"> <label for="disabledFileInput" class="form-label">Disabled file input</label> <input class="form-control" type="file" id="disabledFileInput" disabled> </div> <div class="mb-3"> <label for="multipleFileInput" class="form-label">Multiple files input</label> <input class="form-control" type="file" id="multipleFileInput" multiple> </div> </body> </html>
Color
Use .form-control-color class and type="color" attribute in the <input> element to add color to the input field.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <label for="colorInput" class="form-label">Select a color</label> <input type="color" class="form-control form-control-color" id="colorInput" value="#228b22"> </body> </html>
Datalists
You can create a group of <option>'s that can be accessed from a <input> by using datalists. Browsers and operating systems provide limited and inconsistent styling for <datalist> elements.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Form Control</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <label for="dataList" class="form-label">Languages</label> <input class="form-control" list="datalistOptions" id="dataList" placeholder="Search languages..."> <datalist id="datalistOptions"> <option value="Bootstrap"> <option value="HTML"> <option value="CSS"> </datalist> </body> </html>