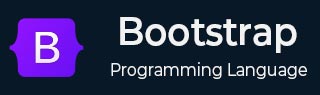
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Progress Bars
This chapter discusses about Bootstrap progress bars. Progress bars in Bootstrap are UI components that display the progress or completion status of a task or process. They are typically used to visually indicate the progress of an operation, such as file uploads, form submissions, or loading of data.
Bootstrap provides a set of in-built classes to create progress bars with different styles, sizes and animations. Bootstrap also provides additional classes and options for customizing the appearance and behavior of progress bars, such as setting different colors, adding labels, using striped or animated progress bars, and stacking multiple progress bars together.
Use the .progress as a wrapper to show the max value of the progress bar.
Use the inner .progress-bar to show the progress so far.
The .progress-bar requires an inline style, utility class, or custom CSS to set their width.
The .progress-bar also requires some role and aria attributes to make it accessible.
The .progress-stacked can be used to create multiple/stacked progress bars.
Basic Progress Bar
Here's an example of a basic Bootstrap progress bar:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar</h4><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 15%" aria-valuenow="0" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 25%" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 50%" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 75%" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 100%" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100"></div> </div> </body> </html>
Bar Sizing
Width
Bootstrap provides a complete list of utilities for setting width. Let us see an example:
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar</h4><br><br> <div class="progress"> <div class="progress-bar w-25" role="progressbar" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100"></div> </div> </body> </html>
Height
By default the height of a progress bar is 1rem, but it can be changed using the CSS height property. You must set the same height for the progress container and the progress bar.
Height value can be set only on the .progress, so once the value of height is changed in the .progress container, the inner .progress-bar automatically resizes.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar Height</h4><br> <div class="progress" style="height: 20px;"> <div class="progress-bar" role="progressbar" style="width: 25%;height: 20px;" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"></div> </div> <br> <div class="progress" style="height: 30px;"> <div class="progress-bar" role="progressbar" style="width: 25%;height: 30px;" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"></div> </div> </body> </html>
Labels
Labels can be added to the progress bars by placing text within the .progress-bar.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar Label</h4><br> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 50%;" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100">50%</div> </div> </body> </html>
Label Overflow Visible/Hidden
In order to avoid the content getting bleed out of the bar, the content inside the .progress-bar is controlled with overflow: hidden
Use overflow: visible from overflow utilities to make the content capped and become readable. This is useful when the progress bar is shorter than the label.
Define an explicit text color to make the text readable.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar Long Label</h4><br> <div class="progress" role="progressbar" aria-label="Example of label" aria-valuenow="10" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar overflow-visible text-dark" style="width: 20%">Overflow visible on progress bar, but the label is too long, but the label is too long,but the label is too long,but the label is too long,but the label is too long,but the label is too long,but the label is too long</div> </div> <br> <div class="progress" role="progressbar" aria-label="Example of label" aria-valuenow="10" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar overflow-hidden text-dark" style="width: 20%">Overflow hidden on progress bar, but the label is too long, but the label is too long,but the label is too long,;/div> </div> </body> </html>
Background
The appearance of individual progress bar can be changed using the background utility classes.
The progress bar is blue by default (primary).
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar Background</h4><br> <!-- Blue --> <div class="progress"> <div class="progress-bar" style="width:10%"></div> </div><br> <!-- Green --> <div class="progress"> <div class="progress-bar bg-success" style="width:20%"></div> </div><br> <!-- Turquoise --> <div class="progress"> <div class="progress-bar bg-info" style="width:30%"></div> </div><br> <!-- Orange --> <div class="progress"> <div class="progress-bar bg-warning" style="width:40%"></div> </div><br> <!-- Red --> <div class="progress"> <div class="progress-bar bg-danger" style="width:50%"></div> </div><br> <!-- White --> <div class="progress border"> <div class="progress-bar bg-white" style="width:60%"></div> </div><br> <!-- Grey --> <div class="progress"> <div class="progress-bar bg-secondary" style="width:70%"></div> </div><br> <!-- Light Grey --> <div class="progress border"> <div class="progress-bar bg-light" style="width:80%"></div> </div><br> <!-- Dark Grey --> <div class="progress"> <div class="progress-bar bg-dark" style="width:90%"></div> </div> </body> </html>
Accessibility tip: Use of color to the progress bars just provides a visual indication, and this will not be helpful to users of assistive technologies like screen readers. Make sure that the meaning is clear from the content itself.
Use alternative means to add clarity to the content using the .visually-hidden class.
In order to make the labels readable on a colored background, choose appropriate text colors.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar - Text Color</h4> <br> </div><div class="progress" role="progressbar" aria-label="Success example" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar bg-success" style="width: 25%">Default text color</div> </div><br> <div class="progress" role="progressbar" aria-label="Info example" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar bg-info text-dark" style="width: 50%">Dark text on Info</div> </div><br> <div class="progress" role="progressbar" aria-label="Warning example" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar bg-warning text-dark" style="width: 75%">Dark text on warning</div> </div><br> <div class="progress" role="progressbar" aria-label="Danger example" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar bg-danger" style="width: 100%">Light text on danger</div> </div> </body> </html>
You can also use the new combined helper classes for text and background color i.e. Color and background.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar - Text & Background Color</h4> <br> <div class="progress" role="progressbar" aria-label="Success example" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100"> <div class="progress-bar text-bg-success" style="width: 75%">Text and Background Color</div> </div> </body> </html>
Multiple Bars
A progress bar can have multiple progress bars stacked in it. Use the Bootstrap class .progress-stacked to create multiple bars.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Progress Bar - Multiple</h4> <br> <div class="progress-stacked"> <div class="progress"> <div class="progress-bar" role="progressbar" style="width: 15%" aria-valuenow="15" aria-valuemin="0" aria-valuemax="100"></div> <div class="progress-bar bg-success" role="progressbar" style="width: 30%" aria-valuenow="30" aria-valuemin="0" aria-valuemax="100"></div> <div class="progress-bar bg-info" role="progressbar" style="width: 20%" aria-valuenow="20" aria-valuemin="0" aria-valuemax="100"></div> <div class="progress-bar bg-danger" role="progressbar" style="width: 20%" aria-valuenow="20" aria-valuemin="0" aria-valuemax="100"></div> </div> </div> </body> </html>
Striped Progress Bar
Stripes can be added to a progress bar using .progress-bar-striped class to any .progress-bar.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Striped Progress Bar</h4><br> <div class="progress"> <div class="progress-bar progress-bar-striped" role="progressbar" style="width: 10%" aria-valuenow="10" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar progress-bar-striped bg-success" role="progressbar" style="width: 25%" aria-valuenow="25" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar progress-bar-striped bg-info" role="progressbar" style="width: 50%" aria-valuenow="50" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar progress-bar-striped bg-warning" role="progressbar" style="width: 75%" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100"></div> </div><br> <div class="progress"> <div class="progress-bar progress-bar-striped bg-danger" role="progressbar" style="width: 100%" aria-valuenow="100" aria-valuemin="0" aria-valuemax="100"></div> </div> </body> </html>
Animated Stripes
Animation can be added to a progress bar, where the stripes get animated right to left via CSS3 animations. Add.progress-bar-animated class to .progress-bar.
Example
You can edit and try running this code using the Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Progress</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <h4>Animated Progress Bar</h4><br> <div class="progress"> <div class="progress-bar progress-bar-striped progress-bar-animated" role="progressbar" aria-valuenow="75" aria-valuemin="0" aria-valuemax="100" style="width: 75%"></div> </div> </body> </html>