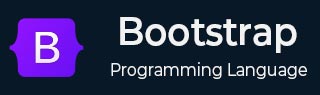
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Form Layout
This chapter will discuss about Bootstrap form layout. Add structure to your forms with form layout options, from inline to horizontal to implementing custom grids.
Form layout
Bootstrap gives no default styling for the <form> element, but there are a few capable browser highlights that are given by default. Each group of form fields must be inside a <form> element.
Ensure to be specify and include a type attribute for <button>s within a <form> as they default to type="submit".
Forms are stacked vertically by default. Bootstrap uses display: block and width: 100% to nearly all form controls. To change layout of each form, use additional classes.
Utilities
To add some structure to forms should be use margin utilities. Use basic grouping of labels, controls, optional form text, and form validation messages.
For consistency, sticking to margin-bottom utilities and employing a single direction all through the form is recommended.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout </title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="mb-3"> <label for="formGroupExampleInput" class="form-label">Username</label> <input type="text" class="form-control" id="formGroupExampleInput" placeholder="Username"> </div> <div class="mb-3"> <label for="formGroupExampleInput2" class="form-label">Password</label> <input type="text" class="form-control" id="formGroupExampleInput2" placeholder="password"> </div> </body> </html>
Form grid
Use grid classes for building the more complex forms. Form a grid for form layouts that require multiple columns, varied widths, and additional alignment options. You need to enable Sass variable using $enable-grid-classes.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"></script> </head> <body> <div class="row mt-2"> <div class="col"> <input type="text" class="form-control" placeholder="Username" aria-label="Username"> </div> <div class="col"> <input type="text" class="form-control" placeholder="Password" aria-label="Password"> </div> </div> </body> </html>
Gutters
To control over the gutter width in the inline as block direction use the gutter modifier classes. By using $enable-grid-classes you can enable Sass variable.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="row g-3 mt-2"> <div class="col"> <input type="text" class="form-control" placeholder="Username" aria-label="Username"> </div> <div class="col"> <input type="text" class="form-control" placeholder="Password" aria-label="Password"> </div> </div> </body> </html>
Create more complex layouts using the grid system.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <form class="row g-3"> <div class="col-md-6"> <label for="inputFirst" class="form-label">First name</label> <input type="text" class="form-control" id="inputFirst"> </div> <div class="col-md-6"> <label for="inputLast" class="form-label">Last name</label> <input type="text" class="form-control" id="inputLast"> </div> <div class="col-md-6"> <label for="inputEmail" class="form-label">Email</label> <input type="email" class="form-control" id="inputEmail"> </div> <div class="col-6"> <label for="inputPassword" class="form-label">Password</label> <input type="Password" class="form-control" id="inputPassword" placeholder="password"> </div> <div class="col-12"> <label for="inputAddress" class="form-label">Address</label> <input type="text" class="form-control" id="inputAddress" placeholder="Address"> </div> <div class="col-md-6"> <label for="inputAdharno" class="form-label">Adharcard no</label> <input type="text" class="form-control" id="inputAdharno"> </div> <div class="col-md-6"> <label for="inputMobno" class="form-label">Mobile no</label> <input type="text" class="form-control" id="inputMobno"> </div> <div class="col-12"> <div class="form-check"> <input class="form-check-input" type="checkbox" id="gridCheck"> <label class="form-check-label" for="gridCheck"> I agree to terms and conditions </label> </div> </div> <div class="col-12"> <button type="submit" class="btn btn-primary">Submit</button> </div> </form> </body> </html>
Horizontal form
Use the class .row to form groups to create horizontal forms with the grid. Use the class .col-*-* to define the width of the labels and controls.
To make a form vertically centered with their associated form controls, use the class .col-form-label to <label>.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <form> <div class="row mb-3"> <label for="inputEmail3" class="col-sm-2 col-form-label">Email</label> <div class="col-sm-10"> <input type="email" class="form-control" id="inputEmail3"> </div> </div> <div class="row mb-3"> <label for="inputPassword3" class="col-sm-2 col-form-label">Password</label> <div class="col-sm-10"> <input type="password" class="form-control" id="inputPassword3"> </div> </div> <fieldset class="row mb-3"> <legend class="col-form-label col-sm-2 pt-0">Language Known</legend> <div class="col-sm-10"> <div class="form-check"> <input class="form-check-input" type="checkbox" name="gridCheck" id="gridCheck1" value="option1" checked> <label class="form-check-label" for="gridCheck1"> English </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" name="gridCheck" id="gridCheck2" value="option2"> <label class="form-check-label" for="gridCheck2"> Hindi </label> </div> <div class="form-check"> <input class="form-check-input" type="checkbox" name="gridCheck" id="gridCheck2" value="option2"> <label class="form-check-label" for="gridCheck2"> marathi </label> </div> <div class="form-check disabled"> <input class="form-check-input"type="checkbox" name="gridCheck" id="gridCheck3" value="option3" disabled> <label class="form-check-label" for="gridCheck3"> Others </label> </div> </div> </fieldset> <div class="row mb-3"> <div class="col-sm-10 offset-sm-2"> <div class="form-check"> <input class="form-check-input" type="radio" id="gridRadio1"> <label class="form-check-label" for="gridRadio1"> Radio Button </label> </div> </div> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> </body> </html>
Horizontal form label sizing
Use the classes .col-form-label-sm or .col-form-label-lg to your <label> or <legend> to follow the size of .form-control-sm and .form-control-lg respectively.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="row mb-3"> <label for="colFormSm" class="col-sm-2 col-form-label col-form-label-sm">Username</label> <div class="col-sm-10"> <input type="email" class="form-control form-control-sm" id="colFormSm" placeholder="Username"> </div> </div> <div class="row mb-3"> <label for="colFormLabel" class="col-sm-2 col-form-label">Password</label> <div class="col-sm-10"> <input type="text" class="form-control" id="colFormLabel" placeholder="Password"> </div> </div> <div class="row"> <label for="colFormLg" class="col-sm-2 col-form-label col-form-label-lg">Email</label> <div class="col-sm-10"> <input type="email" class="form-control form-control-lg" id="colFormLg" placeholder="email"> </div> </div> </body> </html>
Column sizing
As mentioned in the previous example, the grid system allows any number of .cols within a .row. Divide the available width evenly between them. You can also use a specific column class like .col-sm-7 to select a subset of columns to occupy more or less space while the remaining .col divides the rest evenly.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <div class="row g-3"> <div class="col-sm-6"> <input type="text" class="form-control" placeholder="Email" aria-label="Email"> </div> <div class="col-sm-3"> <input type="text" class="form-control" placeholder="Mobile no" aria-label="Mobile no"> </div> <div class="col-sm-2"> <input type="text" class="form-control" placeholder="Age" aria-label="Age"> </div> </div> </body> </html>
Auto-sizing
In the below example, use flexbox utility to center the content vertically and change .col to .col-auto. So that the column only takes up as much space as it needs. In other words, the column size depends on the content.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <form class="row gy-2 gx-3 align-items-center"> <div class="col-auto"> <label class="visually-hidden" for="inputName">Name</label> <input type="text" class="form-control" id="inputName" placeholder="Name"> </div> <div class="col-auto"> <label class="visually-hidden" for="inputGroup"></label> <div class="input-group"> <input type="text" class="form-control" id="inputGroup" placeholder="Username"> <div class="input-group-text">@gmail.com</div> </div> </div> <div class="col-auto"> <label class="visually-hidden" for="selectLanguage">Language</label> <select class="form-select" id="selectLanguage"> <option selected>Choose...</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> </div> <div class="col-auto"> <div class="form-check"> <input class="form-check-input" type="checkbox" id="autoSizingCheck"> <label class="form-check-label" for="autoSizingCheck"> Remember me </label> </div> </div> <div class="col-auto"> <button type="submit" class="btn btn-primary">Submit</button> </div> </form> </body> </html>
Then you can remix again with size-specific column classes.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <form class="row gx-3 gy-2 align-items-center mt-2"> <div class="col-sm-3"> <label class="visually-hidden" for="inputName">Name</label> <input type="text" class="form-control" id="inputName" placeholder="Name"> </div> <div class="col-sm-3"> <label class="visually-hidden" for="specificSizeInputGroupUsername">Username</label> <div class="input-group"> <input type="text" class="form-control" id="specificSizeInputGroupUsername" placeholder="Email"> <div class="input-group-text">@gmail.com</div> </div> </div> <div class="col-sm-3"> <label class="visually-hidden" for="selectLanguage">Language</label> <select class="form-select" id="selectLanguage"> <option selected>Choose...</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> </div> <div class="col-auto"> <div class="form-check"> <input class="form-check-input" type="checkbox" id="autoSizingCheck2"> <label class="form-check-label" for="autoSizingCheck2"> Remember me </label> </div> </div> <div class="col-auto"> <button type="submit" class="btn btn-primary">Submit</button> </div> </form> </body> </html>
Inline forms
To build responsive horizontal layouts use the classes .row-cols-*.
Use gutter modifier classes to have gutters in horizontal and vertical directions.
In narrow mobile viewports, .col-12 is useful for stacking form controls, etc.
To align the form elements at the middle and make the .form-check align properly use the class .align-items-center.
Example
You can edit and try running this code using Edit & Run option.
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap - Layout</title> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha3/dist/js/bootstrap.bundle.min.js"> </script> </head> <body> <form class="row row-cols-lg-auto g-3 align-items-center"> <div class="col-12"> <label class="visually-hidden" for="inlineFormInputGroupUsername">Username</label> <div class="input-group"> <input type="text" class="form-control" id="inlineFormInputGroupUsername" placeholder="Username"> <div class="input-group-text">@gmail.com</div> </div> </div> <div class="col-12"> <label class="visually-hidden" for="selectLanguage">Language</label> <select class="form-select" id="selectLanguage"> <option selected>Choose...</option> <option value="1">English</option> <option value="2">Hindi</option> <option value="3">Marathi</option> </select> </div> <div class="col-12"> <div class="form-check"> <input class="form-check-input" type="checkbox" id="inlineFormCheck"> <label class="form-check-label" for="inlineFormCheck"> Remember me </label> </div> </div> <div class="col-12"> <button type="submit" class="btn btn-primary">Submit</button> </div> </form> </body> </html>