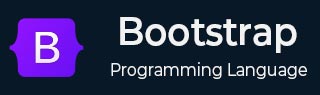
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Popover Plugin
The popover is similar to tooltip, offering an extended view complete with a heading. For the popover to activate, a user just needs to hover the cursor over the element. The content of the popover can be populated entirely using the Bootstrap Data API. This method requires a tooltip.
If you want to include this plugin functionality individually, then you will need the popover.js and it has a dependency of the tooltip plugin. Else, as mentioned in the chapter Bootstrap Plugins Overview, you can include bootstrap.js or the minified bootstrap.min.js.
Usage
The popover plugin generates content and markup on demand, and by default places popover after their trigger element. You can add popover in the following two ways −
Via data attributes − To add a popover, add data-toggle = "popover" to an anchor/button tag. The title of the anchor will be the text of a popover. By default, popover is set to top by the plugin.
<a href = "#" data-toggle = "popover" title = "Example popover"> Hover over me </a>
Via JavaScript − Enable popovers via JavaScript using the following syntax −
$('#identifier').popover(options)
Popover plugin is NOT only-css plugins like dropdown or other plugins discussed in previous chapters. To use this plugin you MUST activate it using jquery (read javascript). To enable all the popovers on your page just use this script −
$(function () { $("[data-toggle = 'popover']").popover(); });
Example
The following example demonstrates the use of popover plugin via data attributes.
<div class = "container" style = "padding: 100px 50px 10px;" > <button type = "button" class = "btn btn-default" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "left" data-content = "Some content in Popover on left"> Popover on left </button> <button type = "button" class = "btn btn-primary" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "top" data-content = "Some content in Popover on top"> Popover on top </button> <button type = "button" class = "btn btn-success" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "bottom" data-content = "Some content in Popover on bottom"> Popover on bottom </button> <button type = "button" class = "btn btn-warning" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "right" data-content = "Some content in Popover on right"> Popover on right </button> </div> <script> $(function (){ $("[data-toggle = 'popover']").popover(); }); </script>
Options
There are certain options which can be added via the Bootstrap Data API or invoked via JavaScript. Following table lists the options −
Option Name | Type/Default Value | Data attribute name | Description |
---|---|---|---|
animation | boolean Default − true | data-animation | Applies a CSS fade transition to the popover. |
html | boolean Default − false | data-html | Inserts HTML into the popover. If false, jQuery’s text method will be used to insert content into the dom. Use text if you’re worried about XSS attacks. |
placement | string|function Default − top | data-placement | Specifies how to position the popover (i.e., top|bottom|left|right|auto). When auto is specified, it will dynamically reorient the popover. For example, if placement is "auto left", the popover will display to the left when possible, otherwise it will display right. |
selector | string Default − false | data-selector | If a selector is provided, popover objects will be delegated to the specified targets. |
title | string | function Default − " | data-title | The title option is the default title value if the title attribute isn’t present. |
trigger | string Default − 'hover focus' | data-trigger | Defines how the popover is triggered − click| hover | focus | manual. You may pass multiple triggers; separate them with a space. |
delay | number | object Default − 0 | data-delay | Delays showing and hiding the popover in ms — does not apply to manual trigger type. If a number is supplied, delay is applied to both hide/show. Object structure is − delay: { show: 500, hide: 100 } |
container | string | false Default − false | data-container | Appends the popover to a specific element. Example: container: 'body' |
Methods
The following are some useful methods for popover −
Method | Description | Example |
---|---|---|
Options − .popover(options) |
Attaches a popover handler to an element collection. |
$().popover(options) |
Toggle − .popover('toggle') |
Toggles an element's popover. |
$('#element').popover('toggle') |
Show − .popover('show') |
Reveals an element's popover. |
$('#element').popover('show') |
Hide − .popover('hide') |
Hides an element's popover. |
$('#element').popover('hide') |
Destroy − .popover('destroy') |
Hides and destroys an element's popover. |
$('#element').popover('destroy') |
Example
The following example demonstrates the popover plugin methods −
<div class = "container" style = "padding: 100px 50px 10px;" > <button type = "button" class = "btn btn-default popover-show" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "left" data-content = "Some content in Popover with show method"> Popover on left </button> <button type = "button" class = "btn btn-primary popover-hide" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "top" data-content = "Some content in Popover-hide method"> Popover on top </button> <button type = "button" class = "btn btn-success popover-destroy" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "bottom" data-content = "Some content in Popover-destroy method"> Popover on bottom </button> <button type = "button" class = "btn btn-warning popover-toggle" title = "Popover title" data-container = "body" data-toggle = "popover" data-placement = "top" data-content = "Some content in Popover-toggle method"> Popover on right </button> <br><br><br><br><br><br> <p class = "popover-options"> <a href = "#" type = "button" class = "btn btn-warning" title = "<h2>Title</h2>" data-container = "body" data-toggle = "popover" data-content = " <h4>Some content in Popover-options method</h4>"> Popover </a> </p> <script> $(function () { $('.popover-show').popover('show');}); $(function () { $('.popover-hide').popover('hide');}); $(function () { $('.popover-destroy').popover('destroy');}); $(function () { $('.popover-toggle').popover('toggle');}); $(function () { $(".popover-options a").popover({html : true });}); </script> </div>
Events
Following table lists the events to work with the popover plugin. This event may be used to hook into the function.
Event | Description | Example |
---|---|---|
show.bs.popover | This event fires immediately when the show instance method is called. |
$('#mypopover').on('show.bs.popover', function () { // do something }) |
shown.bs.popover | This event is fired when the popover has been made visible to the user (will wait for CSS transitions to complete). |
$('#mypopover').on('shown.bs.popover', function () { // do something }) |
hide.bs.popover | This event is fired immediately when the hide instance method has been called. |
$('#mypopover').on('hide.bs.popover', function () { // do something }) |
hidden.bs.popover | This event is fired when the popover has finished being hidden from the user (will wait for CSS transitions to complete). |
$('#mypopover').on('hidden.bs.popover', function () { // do something }) |
Example
The following example demonstrates the Popover plugin events −
<div clas = "container" style = "padding: 100px 50px 10px;" > <button type = "button" class = "btn btn-primary popover-show" title = "Popover title" data-container = "body" data-toggle = "popover" data-content = "Some content in Popover with show method"> Popover on left </button> </div> <script> $(function () { $('.popover-show').popover('show');}); $(function () { $('.popover-show').on('shown.bs.popover', function () { alert("Alert message on show"); })}); </script>
To Continue Learning Please Login