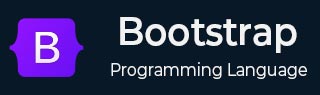
- Bootstrap Tutorial
- Bootstrap - Home
- Bootstrap - Overview
- Bootstrap - Environment Setup
- Bootstrap - RTL
- Bootstrap - CSS Variables
- Bootstrap - Color Modes
- Bootstrap Layouts
- Bootstrap - Breakpoints
- Bootstrap - Containers
- Bootstrap - Grid System
- Bootstrap - Columns
- Bootstrap - Gutters
- Bootstrap - Utilities
- Bootstrap - CSS Grid
- Bootstrap Content
- Bootstrap - Reboot
- Bootstrap - Typography
- Bootstrap - Images
- Bootstrap - Tables
- Bootstrap - Figures
- Bootstrap Components
- Bootstrap - Accordion
- Bootstrap - Alerts
- Bootstrap - Badges
- Bootstrap - Breadcrumb
- Bootstrap - Buttons
- Bootstrap - Button Groups
- Bootstrap - Cards
- Bootstrap - Carousel
- Bootstrap - Close button
- Bootstrap - Collapse
- Bootstrap - Dropdowns
- Bootstrap - List Group
- Bootstrap - Modal
- Bootstrap - Navbars
- Bootstrap - Navs & tabs
- Bootstrap - Offcanvas
- Bootstrap - Pagination
- Bootstrap - Placeholders
- Bootstrap - Popovers
- Bootstrap - Progress
- Bootstrap - Scrollspy
- Bootstrap - Spinners
- Bootstrap - Toasts
- Bootstrap - Tooltips
- Bootstrap Forms
- Bootstrap - Forms
- Bootstrap - Form Control
- Bootstrap - Select
- Bootstrap - Checks & radios
- Bootstrap - Range
- Bootstrap - Input Groups
- Bootstrap - Floating Labels
- Bootstrap - Layout
- Bootstrap - Validation
- Bootstrap Helpers
- Bootstrap - Clearfix
- Bootstrap - Color & background
- Bootstrap - Colored Links
- Bootstrap - Focus Ring
- Bootstrap - Icon Link
- Bootstrap - Position
- Bootstrap - Ratio
- Bootstrap - Stacks
- Bootstrap - Stretched link
- Bootstrap - Text Truncation
- Bootstrap - Vertical Rule
- Bootstrap - Visually Hidden
- Bootstrap Utilities
- Bootstrap - Backgrounds
- Bootstrap - Borders
- Bootstrap - Colors
- Bootstrap - Display
- Bootstrap - Flex
- Bootstrap - Floats
- Bootstrap - Interactions
- Bootstrap - Link
- Bootstrap - Object Fit
- Bootstrap - Opacity
- Bootstrap - Overflow
- Bootstrap - Position
- Bootstrap - Shadows
- Bootstrap - Sizing
- Bootstrap - Spacing
- Bootstrap - Text
- Bootstrap - Vertical Align
- Bootstrap - Visibility
- Bootstrap Demos
- Bootstrap - Grid Demo
- Bootstrap - Buttons Demo
- Bootstrap - Navigation Demo
- Bootstrap - Blog Demo
- Bootstrap - Slider Demo
- Bootstrap - Carousel Demo
- Bootstrap - Headers Demo
- Bootstrap - Footers Demo
- Bootstrap - Heroes Demo
- Bootstrap - Featured Demo
- Bootstrap - Sidebars Demo
- Bootstrap - Dropdowns Demo
- Bootstrap - List groups Demo
- Bootstrap - Modals Demo
- Bootstrap - Badges Demo
- Bootstrap - Breadcrumbs Demo
- Bootstrap - Jumbotrons Demo
- Bootstrap-Sticky footer Demo
- Bootstrap-Album Demo
- Bootstrap-Sign In Demo
- Bootstrap-Pricing Demo
- Bootstrap-Checkout Demo
- Bootstrap-Product Demo
- Bootstrap-Cover Demo
- Bootstrap-Dashboard Demo
- Bootstrap-Sticky footer navbar Demo
- Bootstrap-Masonry Demo
- Bootstrap-Starter template Demo
- Bootstrap-Album RTL Demo
- Bootstrap-Checkout RTL Demo
- Bootstrap-Carousel RTL Demo
- Bootstrap-Blog RTL Demo
- Bootstrap-Dashboard RTL Demo
- Bootstrap Useful Resources
- Bootstrap - Questions and Answers
- Bootstrap - Quick Guide
- Bootstrap - Useful Resources
- Bootstrap - Discussion
Bootstrap - Carousel Plugin
The Bootstrap carousel is a flexible, responsive way to add a slider to your site. In addition to being responsive, the content is flexible enough to allow images, iframes, videos, or just about any type of content that you might want.
If you want to include this plugin functionality individually, then you will need the carousel.js. Else, as mentioned in the chapter Bootstrap Plugins Overview, you can include the bootstrap.js or the minified bootstrap.min.js.
Example
A simple slideshow below shows a generic component for cycling through the elements like a carousel, using the Bootstrap carousel plugin. To implement the carousel, you just need to add the code with the markup. There is no need for data attributes, just simple class-based development.
<div id = "myCarousel" class = "carousel slide"> <!-- Carousel indicators --> <ol class = "carousel-indicators"> <li data-target = "#myCarousel" data-slide-to = "0" class = "active"></li> <li data-target = "#myCarousel" data-slide-to = "1"></li> <li data-target = "#myCarousel" data-slide-to = "2"></li> </ol> <!-- Carousel items --> <div class = "carousel-inner"> <div class = "item active"> <img src = "/bootstrap/images/slide1.png" alt = "First slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide2.png" alt = "Second slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide3.png" alt = "Third slide"> </div> </div> <!-- Carousel nav --> <a class = "carousel-control left" href = "#myCarousel" data-slide = "prev">‹</a> <a class = "carousel-control right" href = "#myCarousel" data-slide = "next">›</a> </div>
Optional Captions
You can add captions to your slides easily with the .carousel-caption element within any .item. Place just about any optional HTML within there and it will be automatically aligned and formatted. The following example demonstrates this −
<div id = "myCarousel" class = "carousel slide"> <!-- Carousel indicators --> <ol class = "carousel-indicators"> <li data-target = "#myCarousel" data-slide-to = "0" class = "active"></li> <li data-target = "#myCarousel" data-slide-to = "1"></li> <li data-target = "#myCarousel" data-slide-to = "2"></li> </ol> <!-- Carousel items --> <div class = "carousel-inner"> <div class = "item active"> <img src = "/bootstrap/images/slide1.png" alt = "First slide"> <div class = "carousel-caption">This Caption 1</div> </div> <div class = "item"> <img src = "/bootstrap/images/slide2.png" alt = "Second slide"> <div class = "carousel-caption">This Caption 2</div> </div> <div class = "item"> <img src = "/bootstrap/images/slide3.png" alt = "Third slide"> <div class = "carousel-caption">This Caption 3</div> </div> </div> <!-- Carousel nav --> <a class = "carousel-control left" href = "#myCarousel" data-slide = "prev">‹</a> <a class = "carousel-control right" href = "#myCarousel" data-slide = "next">›</a>+ </div>
Usage
Via data attributes − Use data attributes to easily control the position of the carousel.
Attribute data-slide accepts the keywords prev or next, which alters the slide position relative to its current position.
Use data-slide-to to pass a raw slide index to the carousel data-slide-to = "2", which shifts the slide position to a particular index beginning with 0.
The data-ride = "carousel" attribute is used to mark a carousel as an animation starting at page load.
Via JavaScript − The carousel can be manually called with JavaScript as below −
$('.carousel').carousel()
Options
There are certain, options which can be passed via data attributes or JavaScript are listed in the following table −
Option Name | Type/Default Value | Data attribute name | Description |
---|---|---|---|
interval | number Default − 5000 | data-interval | The amount of time to delay between automatically cycling an item. If false, carousel will not automatically cycle. |
pause | string Default − "hover" | data-pause | Pauses the cycling of the carousel on mouseenter and resumes the cycling of the carousel on mouseleave. |
wrap | boolean Default − true | data-wrap | Whether the carousel should cycle continuously or have hard stops. |
Methods
Here is a list of useful methods that can be used with carousel code.
Method | Description | Example |
---|---|---|
.carousel(options) | Initializes the carousel with an optional options object and starts cycling through items. |
$('#identifier').carousel({ interval: 2000 }) |
.carousel('cycle') | Cycles through the carousel items from left to right. |
$('#identifier').carousel('cycle') |
.carousel('pause') | Stops the carousel from cycling through items. |
$('#identifier')..carousel('pause') |
.carousel(number) | Cycles the carousel to a particular frame (0 based, similar to an array). |
$('#identifier').carousel(number) |
.carousel('prev') | Cycles to the previous item. |
$('#identifier').carousel('prev') |
.carousel('next') | Cycles to the next item. |
$('#identifier').carousel('next') |
Example
The following example demonstrates the usage of methods −
<div id = "myCarousel" class = "carousel slide"> <!-- Carousel indicators --> <ol class = "carousel-indicators"> <li data-target = "#myCarousel" data-slide-to = "0" class = "active"></li> <li data-target = "#myCarousel" data-slide-to = "1"></li> <li data-target = "#myCarousel" data-slide-to = "2"></li> </ol> <!-- Carousel items --> <div class = "carousel-inner"> <div class = "item active"> <img src = "/bootstrap/images/slide1.png" alt = "First slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide2.png" alt = "Second slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide3.png" alt = "Third slide"> </div> </div> <!-- Carousel nav --> <a class = "carousel-control left" href = "#myCarousel" data-slide = "prev">‹</a> <a class = "carousel-control right" href = "#myCarousel" data-slide = "next">›</a> <!-- Controls buttons --> <div style = "text-align:center;"> <input type = "button" class = "btn prev-slide" value = "Previous Slide"> <input type = "button" class = "btn next-slide" value = "Next Slide"> <input type = "button" class = "btn slide-one" value = "Slide 1"> <input type = "button" class = "btn slide-two" value = "Slide 2"> <input type = "button" class = "btn slide-three" value = "Slide 3"> </div> </div> <script> $(function() { // Cycles to the previous item $(".prev-slide").click(function() { $("#myCarousel").carousel('prev'); }); // Cycles to the next item $(".next-slide").click(function() { $("#myCarousel").carousel('next'); }); // Cycles the carousel to a particular frame $(".slide-one").click(function() { $("#myCarousel").carousel(0); }); $(".slide-two").click(function() { $("#myCarousel").carousel(1); }); $(".slide-three").click(function() { $("#myCarousel").carousel(2); }); }); </script>
Events
Bootstrap's carousel class exposes two events for hooking into carousel functionality which are listed in the following table.
Event | Description | Example |
---|---|---|
slide.bs.carousel | This event fires immediately when the slide instance method is invoked. |
$('#identifier').on('slide.bs.carousel', function () { // do something }) |
slid.bs.carousel | This event is fired when the carousel has completed its slide transition. |
$('#identifier').on('slid.bs.carousel', function () { // do something }) |
Example
The following example demonstrates the usage of events −
<div id = "myCarousel" class = "carousel slide"> <!-- Carousel indicators --> <ol class = "carousel-indicators"> <li data-target = "#myCarousel" data-slide-to = "0" class = "active"></li> <li data-target = "#myCarousel" data-slide-to = "1"></li> <li data-target = "#myCarousel" data-slide-to = "2"></li> </ol> <!-- Carousel items --> <div class = "carousel-inner"> <div class = "item active"> <img src = "/bootstrap/images/slide1.png" alt = "First slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide2.png" alt = "Second slide"> </div> <div class = "item"> <img src = "/bootstrap/images/slide3.png" alt = "Third slide"> </div> </div> <!-- Carousel nav --> <a class = "carousel-control left" href = "#myCarousel" data-slide = "prev">‹</a> <a class = "carousel-control right" href = "#myCarousel" data-slide = "next">›</a> </div> <script> $(function() { $('#myCarousel').on('slide.bs.carousel', function () { alert("This event fires immediately when the slide instance method" +"is invoked."); }); }); </script>