
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 7401 Articles for C++

74 Views
In this article, we will discuss two different approaches to find out the largest number smaller than a given number which is not a perfect square. In the first approach, we will run a loop to check for every number until we find the desired number while in the second approach, we will use the concept of square root to generate the perfect square number just smaller than the given number and based on this, we will find out the largest number smaller than "nums" which is not a perfect square. Let us first understand the problem statement. Problem Statement ... Read More

45 Views
We have an array "nums" consisting of "size" elements and an integer "number" denoting the number of smallest elements we have to return. Our task is to find out the "number" smallest elements from the given array. The order of the elements should be preserved and we are not allowed to use any extra variable space for the solution i.e., the space complexity of the solution should be O(1). Let us understand this using an example, nums = { 4, 2, 6, 5, 1 } The solution should return 4, 2, 5 as they are the smallest 3 ... Read More

52 Views
In this article, we will discuss about a special type of number called fibonomial coefficient and how does a fibonomial triangle looks like. We will also discuss the C++ code approach to print a fibonomial triangle with a given height. Let us first discuss that what is a fibonomial coefficient. Fibonomial Coefficient We can call a fibonomial coefficient a generalisation of well known terms namely , Fibonacci numbers and binomial coefficients. The Fibonacci numbers are a series of numbers in which, each number is the sum of the two preceding numbers (for example − 0, 1, 1, 2, 3, 5, ... Read More

182 Views
In this article, we will discuss a simple program which calculates the factorial of all the numbers present in the Fibonacci series smaller than a given nums. Problem Statement We are given a number and our task is to generate the factorial of all the numbers present in the Fibonacci series and smaller than the given number. Let us first understand the problem statement and requirements from the code solution with the help of examples. Input nums = 13 Output Fibonacci series up to 13 is 0, 1, 1, 2, 3, 5, So, the factorial ... Read More
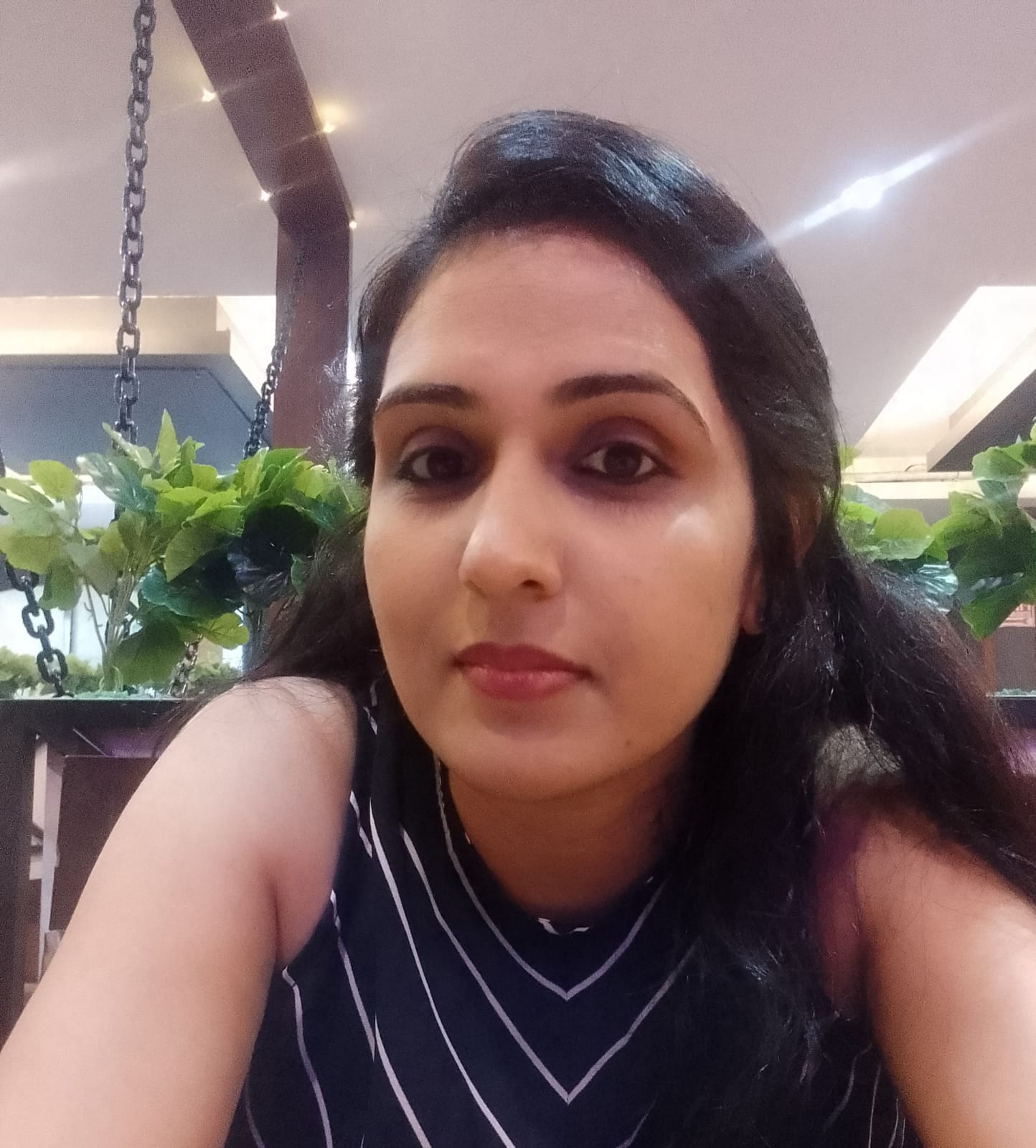
1K+ Views
Introduction In this tutorial, we will understand different types of tries and their uses. Tries are tree-like data structures that are mostly used for operations like string searching. There are various types of trie and they are used as per the task requirement. Generally, trie tries are of three types: Standard trie, compressed trie, and suffix trie. We elaborate on the meaning of each type of trie. What is Trie A trie is a sorted binary tree also called a digital tree or a prefix tree. It has nodes that are used to store data or alphabets. Each node can ... Read More
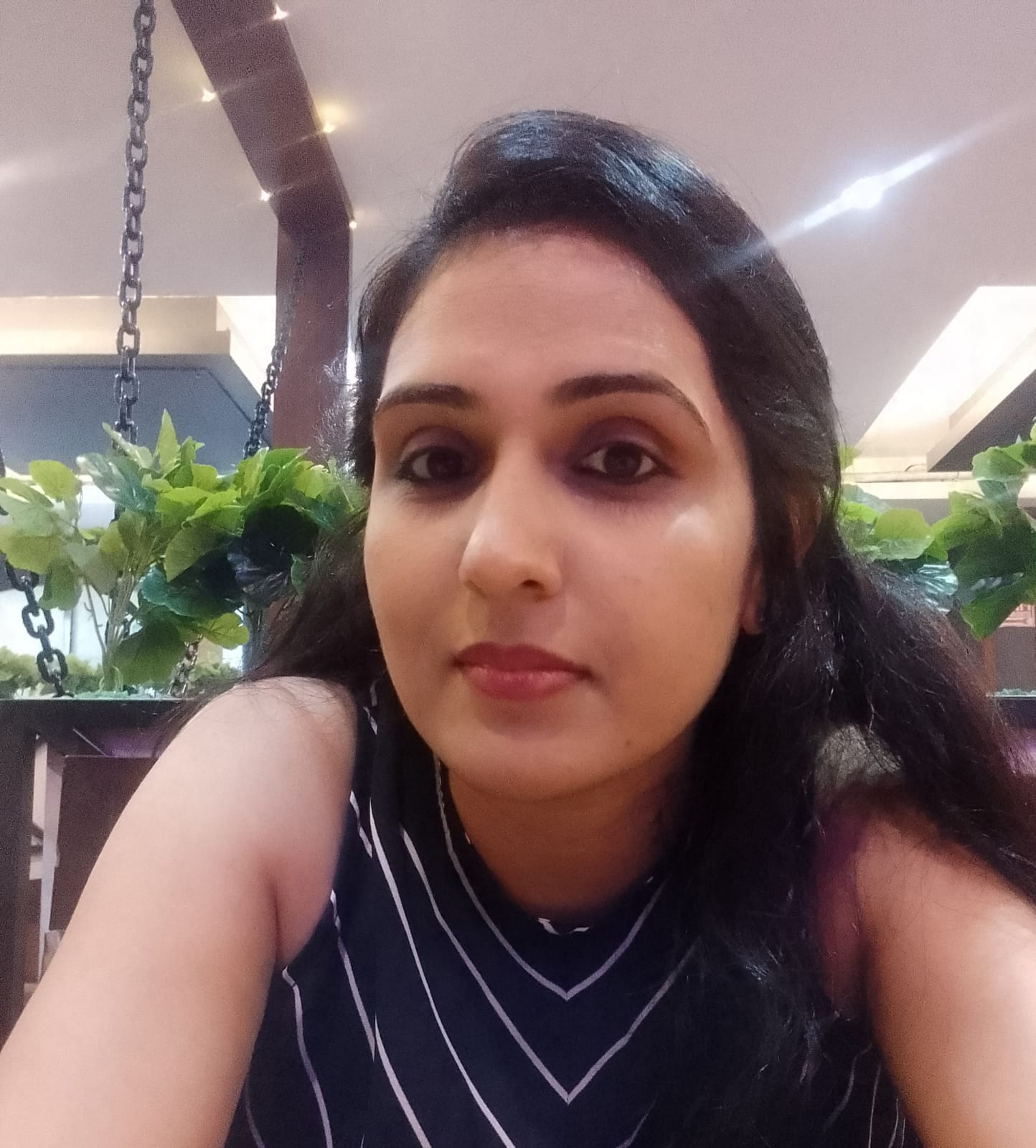
49 Views
Introduction In this tutorial, we implement an approach to sort a string on the basis of the number of matchsticks required for its representation. In this approach, we use N numbers of matchsticks and sort an array. The array can contain numbers, words, or both. Matchsticks are used to arrange them in the shape of a particular number or character. Demonstration 1 Input = Arr = ["1", "3", "4"] Output = The sorted array is 1 4 3 Explanation In the above input array, the array elements are 1, 3, and 4 Number of matchsticks required for ... Read More
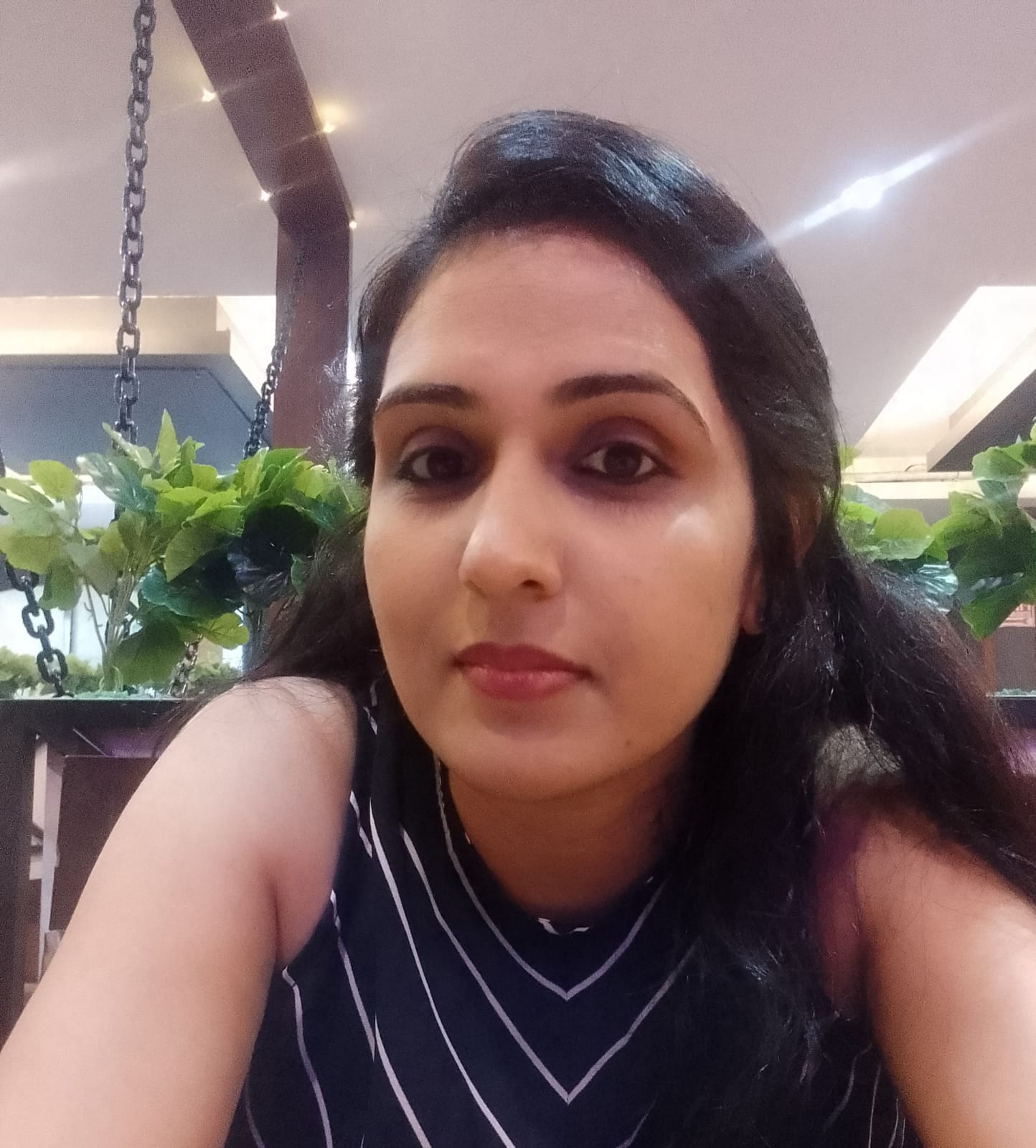
74 Views
Introduction This tutorial deals with the problem of sorting an array alphabetically while each number is converted into words. To translate numbers into words means changing numbers to their number names. Like, 65 is sixty-five. Here, we consider a numerical array, convert all array elements to words and arrange them alphabetically. Print the sorted array elements after converting words to their respective numbers. Demonstration 1 Input = Arr = {13, 1, 6, 7} Output = 1 7 6 13 Explanation The input array elements are 13, 1, 6, 7 The output with alphabetically sorted elements is 1 7 ... Read More
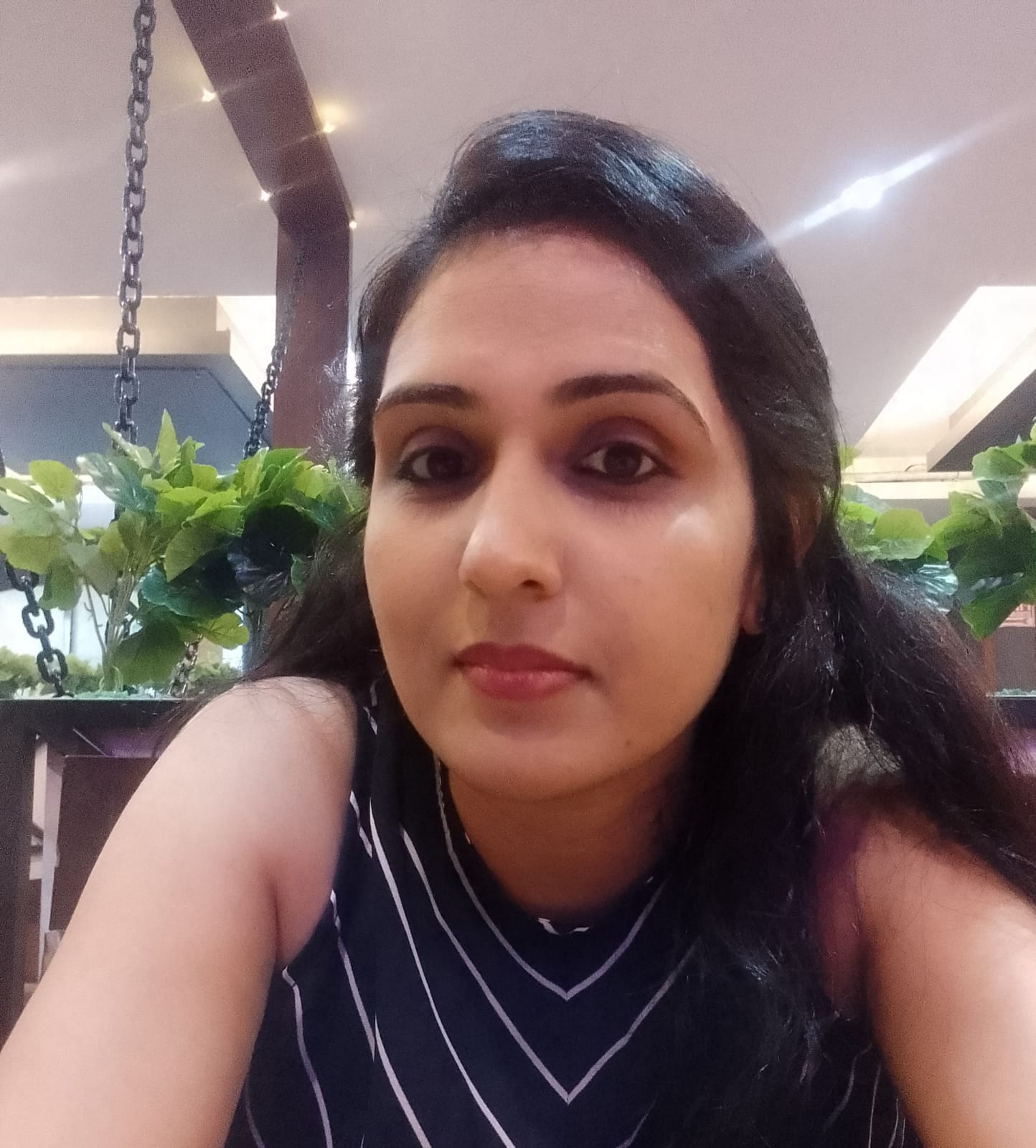
78 Views
Introduction In this tutorial, we find an approach to find the range update Queries to XOR with 1 in a binary array. To implement the approach we use a binary array which is an array consisting of 0 and 1. The range update queries are the queries which are used to modify the binary array within the given upper and lower limit of a range. The upper and lower limits are the index of binary array elements. The elements lying in that range are updated with defined operations. XOR is one of the bitwise operations standing for exclusive OR. Its ... Read More
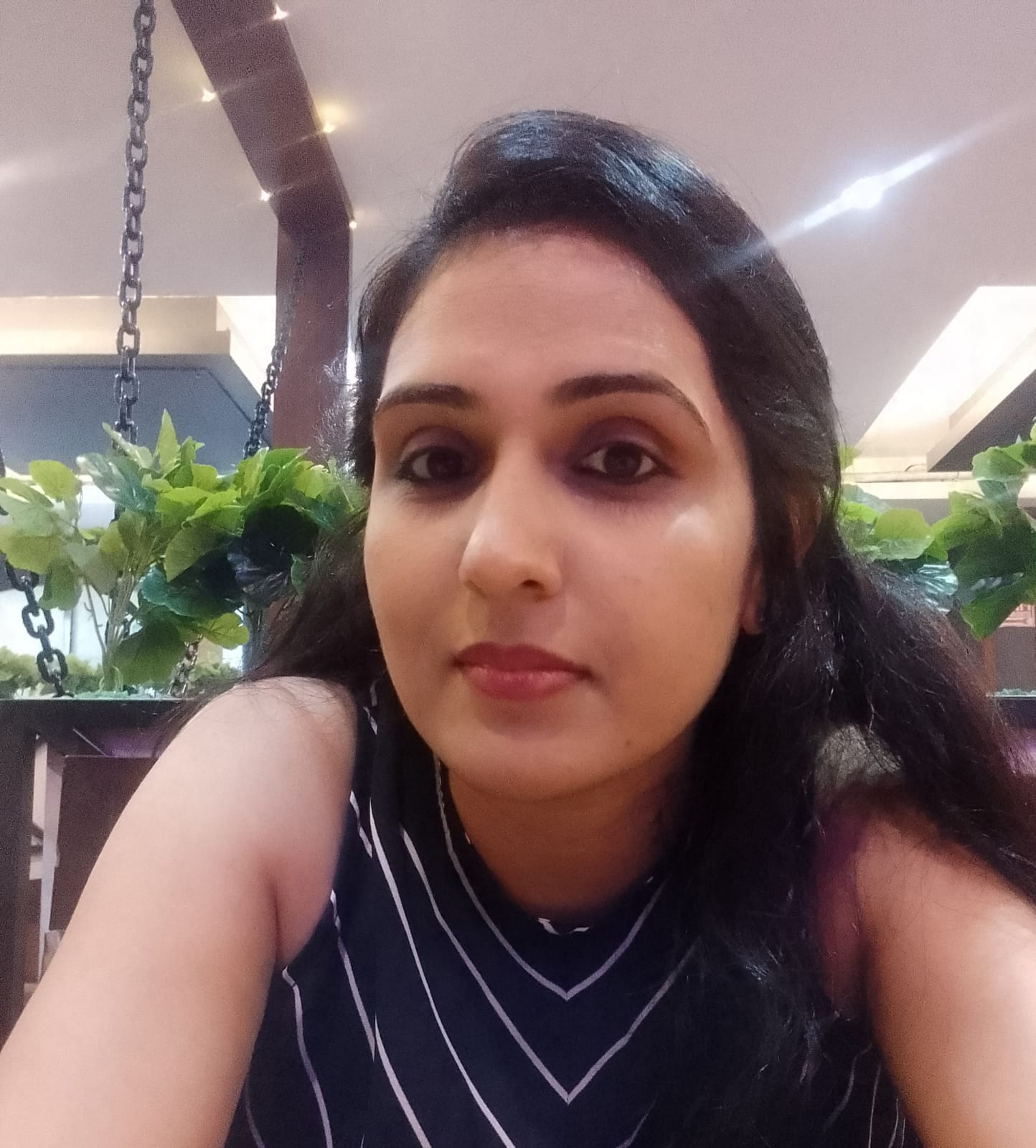
53 Views
Introduction In this tutorial, the task is to use queries to search for an element in an array. It is to push to the front after every query in C++. To implement this task, it takes an array A with elements from 1 to 5 and a query array Q for finding the element in A and moving it to the front of the array. The output is the index number of the searched elements. We use two methods for moving array elements to the front as per the query array. Naive Approach − Iterate through the array of ... Read More
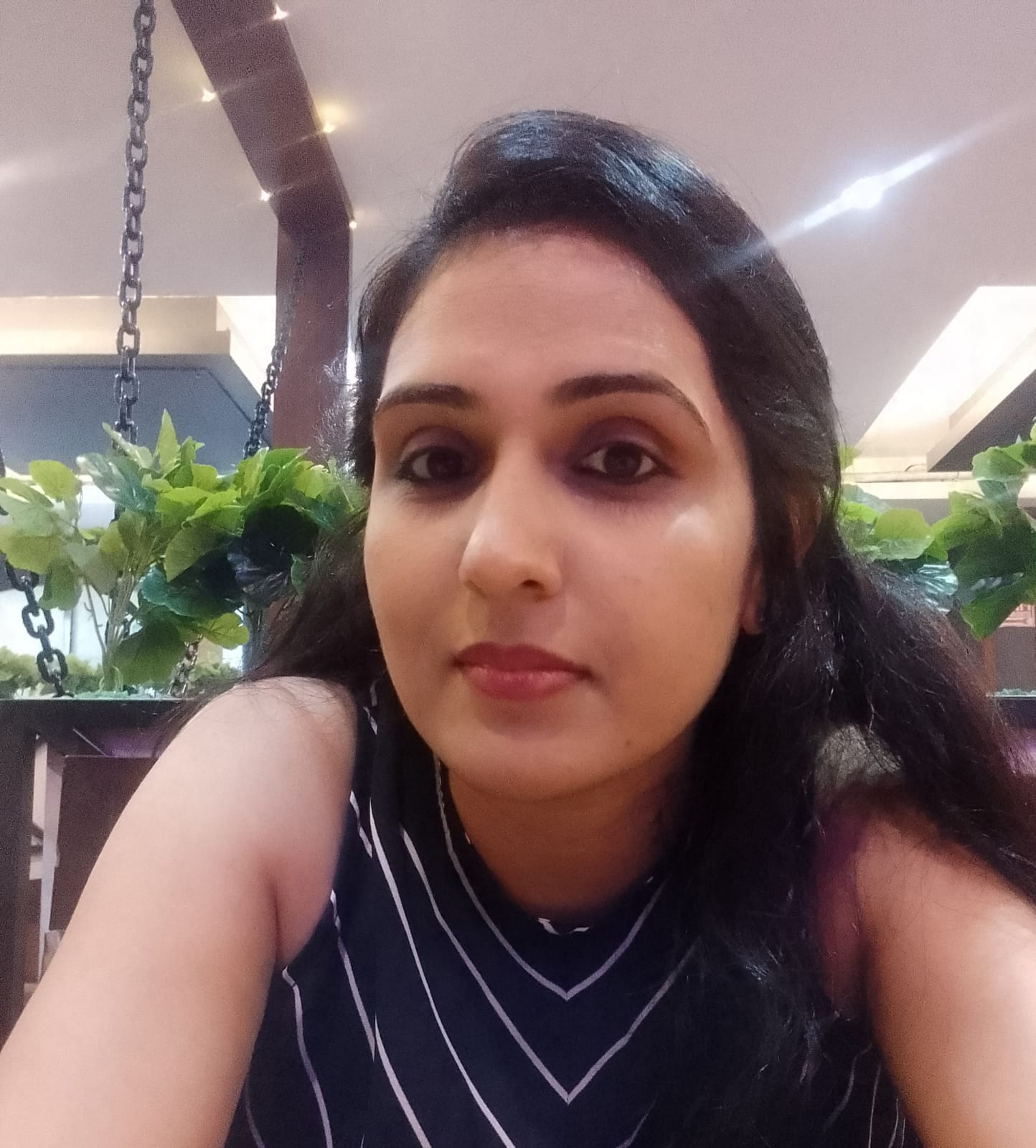
590 Views
Introduction In this tutorial, we will learn about the istringstream and how to use it for processing strings. istringstream is an object of string class defined in the header file. It is used for reading from the stream strings. A stream is a flow of data (collection of characters) between input-output devices and programs. The header file defines 3 string class objects, which are as follows − istringstream ostringstream Stringstream All are used for separate operations, like istringstream is responsible for stream input, ostringstream is for stream output, and stringstream handles both input and output of ... Read More