
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10877 Articles for Web Development
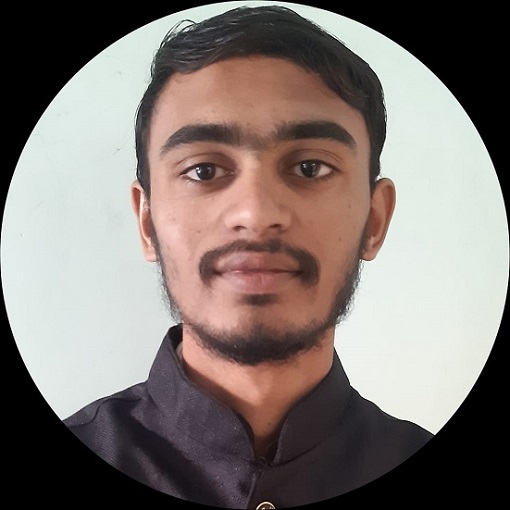
4K+ Views
In this tutorial, we will learn to compare a string to the Boolean in JavaScript. The JavaScript has an equality operator to compare one value with another value.There are two types of equality operators in JavaScript; one is an equality operator (==), and another is a strict equality operator (===). The difference between both of them is they compare operands differently.The equality operator compares the only values of both operands and returns the Boolean value.The strict equality operators first compare the values of both operands, and if the values match, it compares the data type of both operands. In such ... Read More

1K+ Views
The exclamation operator is to perform negation on an expression.ExampleYou can try to run the following code to learn how to implement exclamation(!) operator in JavaScript −Live Demo JavaScript Boolean var answer = true; document.write(answer); document.write(""+!answer);

253 Views
Yes, it is possible to convert Boolean to Integer in JavaScript, using the Ternary Operator.ExampleYou can try to run the following code to convert Boolean to Integer −Live Demo JavaScript Boolean var answer = true; document.write(answer); var pass_marks = answer ? 90 : 40; document.write("Passing Marks: "+pass_marks);
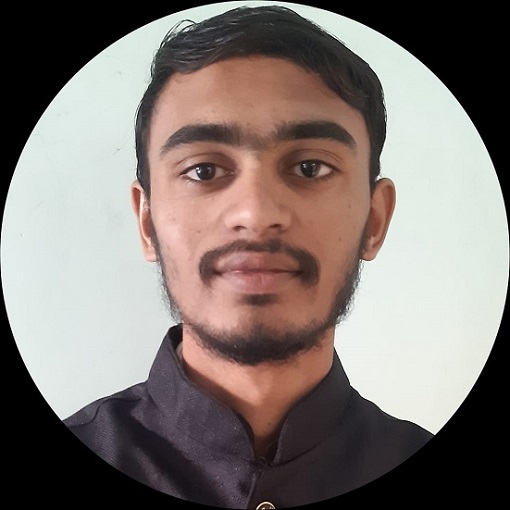
860 Views
This tutorial teaches us to convert the string to the boolean in JavaScript. Suppose that while developing the application, we have stored the boolean value in the database or local storage in the string format. Now, based on the stored string value, we want to convert it to a boolean value and perform a particular operation for the application user; the question arises how can we convert a string to a boolean?There can be many possible ways to solve the above problem. We will learn some useful methods in this tutorial.Using the Comparison (==) and Ternary operator (? :)Using the Boolean classUsing ... Read More

78 Views
To create a String object in JavaScript, use string.constructor. A constructor returns a reference to the string function that created the instance's prototype.ExampleYou can try to run the following code to create a String object with the constructor −Live Demo JavaScript String constructor Method var str = new String( "This is string" ); document.write("str.constructor is:" + str.constructor);

1K+ Views
To get the first index of an occurrence of the specified value in a string, use the JavaScript indexOf() method.ExampleYou can try to run the following code to get the first index −Live Demo JavaScript String indexOf() Method var str = new String( "Learning is fun! Learning is sharing!" ); var index = str.indexOf( "Learning" ); document.write("First index of string Learning :" + index );

15K+ Views
To convert a Boolean value to string value in JavaScript, use the toString() method. This method returns a string of either "true" or "false" depending upon the value of the object.ExampleYou can try to run the following code to convert a boolean value to string value −Live Demo JavaScript toString() Method var flag = new Boolean(true); document.write( "flag.toString is : " + flag.toString() );

10K+ Views
In this tutorial, we will learn how we can change the elements of a JavaScript array in a comma-separated list. Sometimes, we need the elements of a JavaScript array in a format that is not returned by the array itself by default, so we need to write some extra code to implement this task. Fortunately, JavaScript allows us to do so by using some in-built methods. We will discuss these methods in detail. Following are the in-built methods provided by JavaScript to convert the array into a comma-separated list − Array join() method Array toString method Let us ... Read More

4K+ Views
To create a blinking text, use the JavaScript blink() method. This method causes a string to blink as if it were in a BLINK tag.Note − HTML tag deprecated and is not expected to work in every browser.ExampleYou can try to run the following code to create a blinking text with the JavaScript blink() method −Live Demo JavaScript String blink() Method var str = new String("Demo Text"); document.write(str.blink()); document.write(" Note: HTML tag deprecated and is not expected to work in every browser.")

613 Views
To create a big font text, use the JavaScript big() method. This method causes a string to be displayed in a big font as if it were in a BIG tag.ExampleYou can try to run the following code to create a big font text −Live Demo JavaScript String big() Method var str = new String("Demo Text"); document.write("Following is bigger text: "+str.big());
To Continue Learning Please Login