
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Found 10877 Articles for Web Development
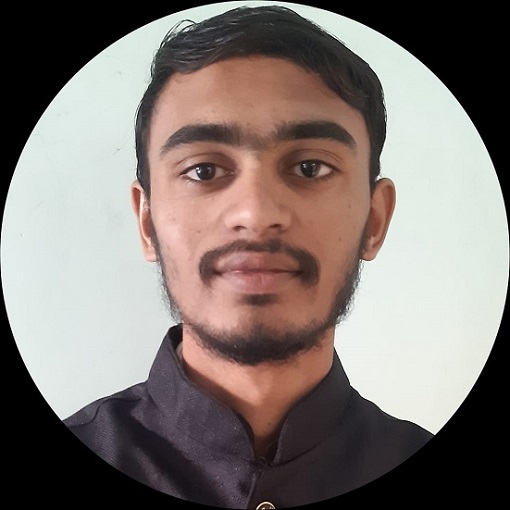
18K+ Views
In this tutorial, we will learn how to get the number of seconds between two dates with JavaScript. There are different methods for checking the number of days, hours, and seconds that are commonly used to provide information related to data. Since you can’t manually change the count of the years and months, we follow some simple tricks to get the number in JavaScript. Using the Date getTime() Method In JavaScript, we use different methods to calculate the days, hours, and seconds. Most popular way to calculate the time is .getTime(). However, you will get the result in milliseconds and ... Read More

533 Views
To convert time in seconds, firstly get the current time. Then, multiply the hours to 3600 and minutes to 60; rest you can see below −(hours*3600) + (min*60) + secExampleYou can try to run the following code to get the current time in seconds −Live Demo JavaScript Get Seconds var dt = new Date(); var sec = dt.getSeconds(); document.write("Seconds: " + sec); var min = dt.getMinutes(); document.write("Minutes: " + min); var hrs = dt.getHours(); document.write("Hours: " + hrs); var total_seconds = (hrs*3600) + (min*60) + sec; document.write("Total seconds: " + total_seconds) ; OutputSeconds: 35 Minutes: 37 Hours: 9 Total seconds: 34655

2K+ Views
To get the time difference between two timestamps, try to run the following code. Here, we are calculating the total number of hours, minutes and seconds between two timestamps −ExampleLive Demo JavaScript Dates var date1, date2; date1 = new Date( "Jan 1, 2018 11:10:05" ); document.write(""+date1); date2 = new Date( "Jan 1, 2018 08:15:10" ); document.write(""+date2); var res ... Read More

26K+ Views
To add months to a date in JavaScript, you can use the Date.setMonth() method. JavaScript date setMonth() method sets the month for a specified date according to local time. This method takes two parameters first is the number of months and the second parameter is the number of days. The counting of the month is start from 0, for example, 0 represents January, 1 represents February ... and so on. Syntax Date.setMonth(monthsValue , [daysValue]); Note − Parameters in the bracket are always optional. Parameter Detail monthsValue − An integer between 0 and 11, representing the months. Although we ... Read More

154 Views
To remove first array element, use the JavaScript shift() method. JavaScript array shift() method removes the first element from an array and returns that element.ExampleYou can try to run the following code to remove first array element and return it −Live Demo JavaScript Array shift Method var element = [30, 45, 70, 90, 110].shift(); document.write("Removed first element is : " + element ); OutputRemoved first element is : 30
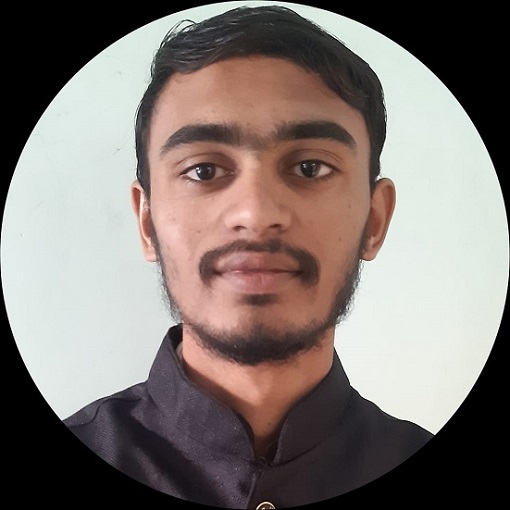
3K+ Views
In this tutorial, we will learn to subtract minutes from the date in JavaScript. While developing the applications, programmers need to play with the dates many times. All JavaScript programmers are lucky enough that JavaScript contains the built-in date class with hundreds of different methods.In this tutorial, we will learn to subtract the minutes from the date in vanilla JavaScript using the date object and the Moment JS library.Using the getMinutes() and setMinutes() MethodsIn this approach, we will create a new date object and extract the minutes from that using the getMinutes() method.After that, we will update the minutes by ... Read More
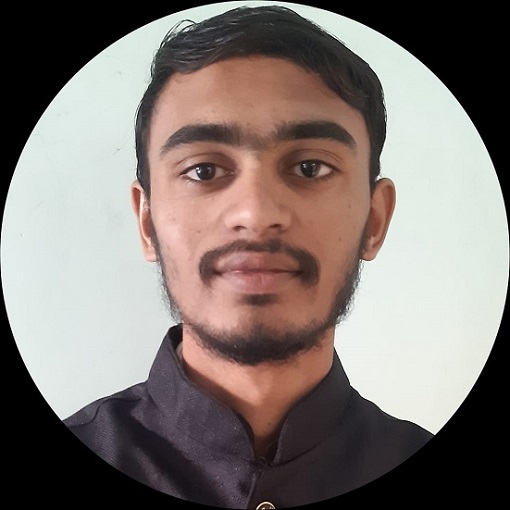
8K+ Views
We will learn to convert the date object to a string with the format hh:mm:ss in JavaScript. It is a rear case that developers don’t use the date and time while developing the application. So, it is also important to learn to manipulate the dates. The default date object returns the date string. Maybe it can be weird as you don’t need to show everything from it. So, users can format the date string according to their requirements. Here, we will see different approaches to format the date string. Format the date using toISOString() method In this approach, we will ... Read More

252 Views
To compare two date objects with JavaScript, create two dates object and get the recent date to compare it with the custom date.ExampleYou can try to run the following code to compare two dates −Live Demo var date1, date2; date1 = new Date(); document.write(date1); date2 = new Date( "Dec 10, 2015 20:15:10" ); document.write(""+date2); if (date1 > date2) { document.write("Date1 is the recent date."); } else { document.write("Date 2 is the recent date."); } OutputMon May 28 2018 09:48:49 GMT+0530 (India Standard Time) Thu Dec 10 2015 20:15:10 GMT+0530 (India Standard Time) Date1 is the recent date

2K+ Views
To get the current year, use the getFullYear() JavaScript method. JavaScript date getFullYear() method returns the year of the specified date according to local time. The value returned by getFullYear() is an absolute number. For dates between the years 1000 and 9999, getFullYear() returns a four-digit number, for example, 2008.ExampleYou can try to run the following code to get the current year −Live Demo JavaScript getFullYear Method var dt = new Date("December 30, 2017 21:25:10"); document.write("getFullYear() : " + dt.getFullYear() ); OutputgetFullYear() : 2017
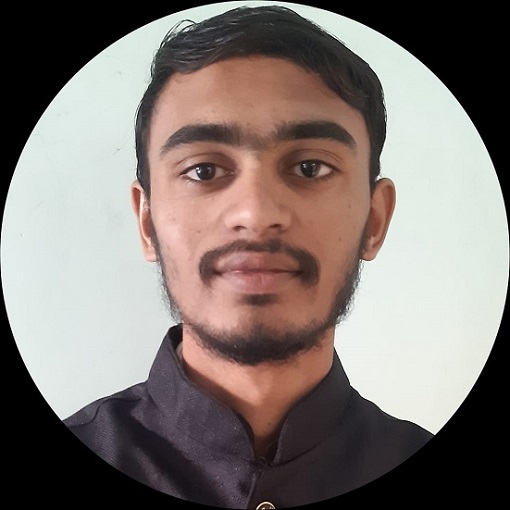
27K+ Views
In this tutorial, we will learn to check whether a JavaScript date is valid or not. Let’s understand why we need to validate the date in JavaScript by example. Suppose you have developed an application that takes some information in the submission form from the user. Users can make a mistake. What if they enter the wrong date in the input box? In such a case, developers need to validate the date. Here, we have three different methods to validate the date. Using the getTime() Method Using the isFinite() Method Using the Moment JS isValid() Method Using the ... Read More
To Continue Learning Please Login