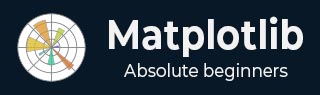
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Setting Font Properties Globally
Setting font properties globally in Matplotlib library involves configuring default font settings for the entire plot using plt.rcParams method. This is useful when we want to apply consistent font styles, sizes or families to all text elements in our visualizations without specifying them individually for each component.
It's important to note that we can tailor these settings based on our preferences and the visual style we want to achieve in our plots. We can adjust the values to match our desired font styles, sizes and families for a consistent and aesthetically pleasing appearance across our visualizations.
The following are the different settings that we can make by using the plt.rcParams method.
plt.rcParams['font.family'] = font_name
This sets the default font family for text elements such as 'sans-serif'. We can replace font_name with the available font families such as 'serif', 'monospace' or specific font names.
Example
In this example we are trying to set the font family to 'sans-serif' by using the plt.rcParams['font.family'].
import matplotlib.pyplot as plt # Set font properties globally plt.rcParams['font.family'] = 'sans-serif' # Create a plot plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('X-axis Label') plt.ylabel('Y-axis Label') plt.title('Font Family setting') plt.show()
Output

plt.rcParams['font.size'] = font_size
We can specify the default font size for text elements in numerical values as per the requirement. This ensures that all text elements use this font size unless overridden for specific components.
Example
In this example we are specifying the fontsize as 8 points to the plt.rcParams['font.size'] to display font size globally all over the plot.
import matplotlib.pyplot as plt # Set font properties globally plt.rcParams['font.size'] = 8 # Create a plot plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('X-axis Label') plt.ylabel('Y-axis Label') plt.title('Font Size setting') plt.show()
Output

plt.rcParams['font.style'] = 'fontstyle'
We can define the font style such as italic etc., as per our interest to the plt.rcParams['font.style']. This applies the defined appearance to text elements throughout the plot.
Example
In this example we are specifying the font style as italic to the plt.rcParams['font.style'].
import matplotlib.pyplot as plt # Set font properties globally plt.rcParams['font.style'] = 'italic' # Create a plot plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('X-axis Label') plt.ylabel('Y-axis Label') plt.title('Font Style setting') plt.show()
Output

plt.rcParams['font.weight'] = font_weight
By using this we can set the font weight such as 'bold' etc. This makes the characters in text elements appear in the defined style as per the user requirement.
Example
Here in this example we are specifying the font weight as bold to the plt.rcParams['font.weight'].
import matplotlib.pyplot as plt # Set font properties globally plt.rcParams['font.weight'] = 'bold' # Create a plot plt.plot([1, 2, 3], [4, 5, 6]) plt.xlabel('X-axis Label') plt.ylabel('Y-axis Label') plt.title('Font weight setting') plt.show()
Output

Note − By setting these parameters globally using plt.rcParams we can ensure that these default font properties are applied to all text elements in our Matplotlib plots. When we create labels, titles or other text components they will inherit these global settings unless we specify different properties locally.
Change the font properties of a Matplotlib colorbar label
In this example we are changing the font properties of a matplotlib colorbar label.
Example
import numpy as np import matplotlib.pyplot as plt plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True x, y = np.mgrid[-1:1:100j, -1:1:100j] z = (x + y) * np.exp(-5.0 * (x ** 2 + y ** 2)) plt.imshow(z, extent=[-1, 1, -1, 1]) cb = plt.colorbar(label='my color bar label') plt.show()
Output

Setting the size of the plotting canvas
Here in this example we are setting the size of the plotting canvas in matplotlib.
Example
import numpy as np from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True x = np.linspace(-2, 2, 100) y = np.sin(x) plt.plot(x, y) plt.show()
Output

Auto adjust font size in Seaborn heatmap using Matplotlib
Here we are auto adjusting the font size in seaborn heatmap by using the Matplotlib library.
Example
import numpy as np import seaborn as sns from matplotlib import pyplot as plt import pandas as pd plt.rcParams["figure.figsize"] = [7.00, 3.50] plt.rcParams["figure.autolayout"] = True d = { 'y=1/x': [1 / i for i in range(1, 10)], 'y=x': [i for i in range(1, 10)], 'y=x^2': [i * i for i in range(1, 10)], 'y=x^3': [i * i * i for i in range(1, 10)] } df = pd.DataFrame(d) ax = sns.heatmap(df, vmax=1) plt.xlabel('Mathematical Expression', fontsize=16) plt.show()
Output

Set the font size of Matplotlib axis Legend
In this example we are setting the font size of matplotlib axis legend
Example
import numpy as np from matplotlib import pyplot as plt import matplotlib plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True x = np.linspace(1, 10, 50) y = np.sin(x) plt.plot(x, y, c="red", lw=7, label="y=sin(x)") plt.title("Sine Curve") matplotlib.rcParams['legend.fontsize'] = 20 plt.legend(loc=1) plt.show()
Output

Modify the font size in Matplotlib-venn
To work with the Matplotlib-venn, first we have to install the package by using the below code. If previously installed we can import directly.
pip install matplotlib-venn
Example
In this example we are modifying the font size in Matplotlib-venn by using the set_fontsize() method.
from matplotlib import pyplot as plt from matplotlib_venn import venn3 plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True set1 = {'a', 'b', 'c', 'd'} set2 = {'a', 'b', 'e'} set3 = {'a', 'd', 'f'} out = venn3([set1, set2, set3], ('Set1', 'Set2', 'Set3')) for text in out.set_labels: text.set_fontsize(25) for text in out.subset_labels: text.set_fontsize(12) plt.show()
Output

Change xticks font size
To change the font size of xticks in a matplotlib plot we can use the fontsize parameter.
Example
from matplotlib import pyplot as plt import numpy as np # Set the figure size plt.rcParams["figure.figsize"] = [7.50, 3.50] plt.rcParams["figure.autolayout"] = True # x and y data points x = np.linspace(-5, 5, 100) y = np.sin(x) plt.plot(x, y) # Set the font size of xticks plt.xticks(fontsize=25) # Display the plot plt.show()
Output

To Continue Learning Please Login