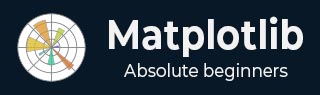
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Image Masking
In Matplotlib library image masking involves selectively displaying parts of an image based on a specified mask which is essentially a binary image defining regions to be shown or hidden. It allows us to apply a filter or condition to reveal or hide specific portions of an image.
Process of Image Masking
The below are the process steps for performing Image Masking.
Create a Mask
Define the criteria or pattern to create the mask. It can be based on colors, shapes, gradients or specific pixel values.
Apply the Mask
Use the mask to modify the transparency or visibility of the corresponding pixels in the original image. Pixels that correspond to areas defined in the mask as "masked" are usually hidden or made transparent while others remain visible.
Overlay or Blend
Overlay or blend the masked image with another image or a background revealing only the unmasked portions. The masked image can be superimposed on another image to combine and display the visible areas.
Types of Image Masks
The below are the types of Image masks.
Binary Masks
Consist of black and white pixels where white represents visible areas and black represents masked areas.
Grayscale Masks
Use various shades of gray to define levels of transparency or partial visibility.
Tools and Techniques for Image Masking
We can use the different tools and techniques for Image masking.
Manual Masking
Tools like Photoshop or GIMP allow users to manually create and edit masks using selection tools, brushes or layer masks.
Programmatic Masking
In programming languages like Python with libraries such as OpenCV or PIL (Pillow) we can create masks using algorithms based on color thresholds, contours or specific image features.
Key Points
Image masking in Matplotlib involves creating a mask array with the same dimensions as the image where specific regions are marked to hide (masked) or reveal (unmasked) portions of the image.
Masking arrays consist of boolean or numerical values where True or non-zero values indicate the regions to be displayed, and False or zero values indicate the regions to be hidden.
Masking allows for selective visualization or manipulation of specific parts of an image based on specified conditions or criteria.
Masking the particular region of the Image
Let's say we have an image and want to mask a particular region displaying only a portion of the image based on certain criteria.
Example
import matplotlib.pyplot as plt import numpy as np # Create a sample image (random pixels) image = np.random.rand(100, 100) # Create a mask to hide certain parts of the image mask = np.zeros_like(image) mask[30:70, 30:70] = 1 # Masking a square region # Apply the mask to the image masked_image = np.ma.masked_array(image, mask=mask) # Display the original and masked images plt.figure(figsize=(8, 4)) plt.subplot(1, 2, 1) plt.imshow(image, cmap='gray') plt.title('Original Image') plt.axis('off') plt.subplot(1, 2, 2) plt.imshow(masked_image, cmap='gray') plt.title('Masked Image') plt.axis('off') plt.show()
Output

Applying mask to an Image
Here this is another of masking an image using the matplotlib library.
Example
import matplotlib.pyplot as plt import numpy as np # Create a sample image image_size = 100 img = np.zeros((image_size, image_size, 3), dtype=np.uint8) img[:, :image_size // 2] = [255, 0, 0] # Set the left half to blue # Create a binary mask mask = np.zeros((image_size, image_size), dtype=np.uint8) mask[:, image_size // 4:] = 1 # Set the right quarter to 1 # Apply the mask to the image masked_img = img * mask[:, :, np.newaxis] # Display the original image, mask, and the masked image plt.figure(figsize=(12, 4)) plt.subplot(131) plt.imshow(img) plt.title('Original Image') plt.axis('off') plt.subplot(132) plt.imshow(mask, cmap='gray') plt.title('Mask') plt.axis('off') plt.subplot(133) plt.imshow(masked_img) plt.title('Masked Image') plt.axis('off') plt.show()
Output

To Continue Learning Please Login