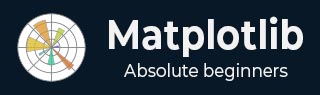
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Saving Figures
Saving figures in Matplotlib library allows us to export our plots to various file formats such as PNG, PDF, SVG and so on to use those saved plots in various reports, presentations or publications. Matplotlib library provides the savefig() function for to save the plot that we have created.
Common File Formats for Saving
PNG (.png) − Good for general-purpose images which supports transparency.
JPEG (.jpg) − Suitable for images with smooth gradients but may lose some quality due to compression.
PDF (.pdf) − Ideal for vector-based images scalable without loss of quality.
SVG (.svg) − Scalable Vector Graphics which suitable for web-based or vector-based graphics.
Saving figures in Matplotlib library is useful for preserving visualizations in various formats by ensuring they can be shared, used or embedded in different contexts as needed. Adjusting the file format and resolution allows us to balance image quality and file size based on your requirements.
Syntax
The following is the syntax and parameters for using the savefig() method.
plt.savefig(fname, dpi=None, bbox_inches='tight', pad_inches=0.1, format=None, kwargs)
Where,
fname − The file name or path of the file to save the figure. The file extension determines the file format such as ".png", ".pdf".
dpi − Dots per inch i.e. resolution for the saved figure. Default is "None" which uses the Matplotlib default.
bbox_inches − Specifies which part of the figure to save. Options include 'tight', 'standard' or a specified bounding box in inches.
pad_inches − Padding around the figure when bbox_inches='tight'.
format − Explicitly specify the file format. If 'None' the format is inferred from the file extension in fname.
kwargs − Additional keyword arguments specific to the chosen file format.
Saving the plot in specified location
In this example we are creating a simple line plot by using the plot() function and then we are trying to save the plotted image in the specified location with the specified filename.
Example
import matplotlib.pyplot as plt # Data x = [22,1,7,2,21,11,14,5] y = [24,2,12,5,5,5,9,12] plt.plot(x,y) # Customize the plot (optional) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Simple Line Plot') # Display the plot plt.savefig('matplotlib/Savefig/lineplot.png') plt.show()
Output
On executing the above code we will get the following output −

Saving plot in .svg format
Here, this is another example of saving the plotted plot by using the savefig() by specifying the file format as svg and dpi as 300 to set the resolution.
Example
import matplotlib.pyplot as plt # Data x = [22,1,7,2,21,11,14,5] y = [24,2,12,5,5,5,9,12] plt.plot(x,y) # Customize the plot (optional) plt.xlabel('X-axis') plt.ylabel('Y-axis') plt.title('Simple Line Plot') # Display the plot plt.savefig('matplotlib/Savefig/lineplot2.svg',dpi = 500) plt.show()
Output
On executing the above code we will get the following output −

Note
We should call savefig() before calling show() if we want to save the figure with the exact appearance shown on the screen otherwise empty file will be saved.
The file extension in the fname parameter determines the format of the saved file. Matplotlib automatically infers the format if format is None.
To Continue Learning Please Login