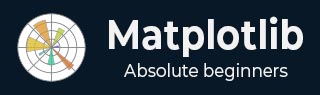
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Font Indexing
In Matplotlib library font indexing refers to accessing and utilizing different fonts or typefaces available for rendering text elements within plots. Matplotlib library provides access to various fonts and understanding font indexing involves knowing how to use these fonts by their names or indices. This is particularly relevant when we want to use fonts that are not in the default set or if we need to use system-specific fonts.
The font.family parameter in Matplotlib accepts either a string representing a known font family or an integer representing a specific font index. The numeric indices are used to reference fonts that are registered with Matplotlib library.
Font Indexing in Matplotlib
The following are the font indexing ways in matplotlib library.
Font Families
Matplotlib library offers several font families such as 'serif', 'sans-serif', 'monospace' and more.
These font families have their unique characteristics and are used to define the general design of the typeface.
Font Names
Matplotlib library allows the use of specific font names to access particular fonts available on our system.
Font names enable the selection of custom or installed fonts for rendering text in plots.
Accessing Fonts by Name
To access fonts in Matplotlib we can use both the font names and their indices if available.
Font Names
We can access the fonts available in matplotlib by using the font names. Here is the example of accessing the font 'Times New Roman' by using the plt.rcParams['font.family'] method.
Example
import matplotlib.pyplot as plt plt.rcParams['font.family'] = 'Times New Roman' # Creating a data x = [i for i in range(10,40)] y = [i for i in range(30,60)] # Creating a plot plt.scatter(x,y,color = 'blue') plt.xlabel('X-axis cube values') plt.ylabel('Y-axis cube values') plt.title('Title with Times New Roman Font') plt.show()
Output

Available Fonts on the System
Matplotlib library can also uses the available fonts on our system. The fonts available might vary based on the operating system such as Windows, macOS, Linux and also the installed font libraries.
Indexing Fonts with findSystemFonts()
Matplotlib provide the function namely matplotlib.font_manager.findSystemFonts() which returns a list of paths to available fonts on the system.
Example
In this example we are using the matplotlib.font_manager.findSystemFonts() function to get the required indices list of font names.
import matplotlib.font_manager as fm # Get a list of available fonts font_list = fm.findSystemFonts() # Display the first five fonts path print("The first five fonts path:",font_list[:5])
Output
The first five fonts path: ['C:\\Windows\\Fonts\\gadugi.ttf', 'C:\\WINDOWS\\Fonts\\COPRGTB.TTF', 'C:\\WINDOWS\\Fonts\\KUNSTLER.TTF', 'C:\\Windows\\Fonts\\OLDENGL.TTF', 'C:\\Windows\\Fonts\\taileb.ttf']
Accessing Fonts by Indexing
Font indexing involves knowing the names or paths of available fonts on our system. We can refer these fonts by their names, aliases or file paths to set the font family in Matplotlib plots by ensuring that the desired font is used for text elements.
Example
Here in this example we are accessing the fonts from the system by using the font path.
import matplotlib as mpl import matplotlib.font_manager as fm plt.rcParams['font.family'] = 'C:\Windows\Fonts\Comicz.ttf' # Creating a data x = [i for i in range(10,40)] y = [i for i in range(30,60)] # Creating a plot plt.plot(x,y,color = 'violet') plt.xlabel('X-axis cube values') plt.ylabel('Y-axis cube values') plt.title("The plot fonts with font indexing") plt.show()
Output

Note
Font indexing and availability may vary depending on the backend being used in Matplotlib library.
Customizing fonts with indices might be backend-specific or require special configurations such as LaTeX rendering.
To Continue Learning Please Login