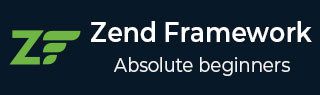
- Zend Framework Tutorial
- Zend Framework - Home
- Zend Framework - Introduction
- Zend Framework - Installation
- Skeleton Application
- Zend Framework - MVC Architecture
- Zend Framework - Concepts
- Zend Framework - Service Manager
- Zend Framework - Event Manager
- Zend Framework - Module System
- Application Structure
- Zend Framework - Creating Module
- Zend Framework - Controllers
- Zend Framework - Routing
- Zend Framework - View Layer
- Zend Framework - Layout
- Models & Database
- Different Databases
- Forms & Validation
- Zend Framework - File Uploading
- Zend Framework - Ajax
- Cookie Management
- Session Management
- Zend Framework - Authentication
- Email Management
- Zend Framework - Unit Testing
- Zend Framework - Error Handling
- Zend Framework - Working Example
- Zend Framework Useful Resources
- Zend Framework - Quick Guide
- Zend Framework - Useful Resources
- Zend Framework - Discussion
Zend Framework - Unit Testing
In general, we can debug a PHP application by using the advanced debugger tool or by using simple commands like echo and die. In a web scenario, we need to test the business logics as well as the presentation layer. Forms in a web application can be tested by entering relevant test data to ensure that the forms are working as expected.
The design of a website can be tested manually by using a browser. These type of test processes can be automated using unit testing. A unit test is essential in large projects. These unit tests will help to automate the testing process and alert the developer when something goes wrong.
Setting up the PHPUnit
Zend framework integrates with the PHPUnit unit testing framework. To write a unit test for the Zend framework, we need to setup the PHPUnit, which can be easily done by using the following Composer command.
$ composer require --dev phpunit/phpunit
After executing the above command, you will get a response as shown in the following code block.
Using version ^5.7 for phpunit/phpunit ./composer.json has been updated Loading composer repositories with package information Updating dependencies (including require-dev) Nothing to install or update Writing lock file Generating autoload files
Now, when you open the “composer.json” file, you will see the following changes −
"require-dev": { "phpunit/phpunit": "^5.7" }
TestCase and Assertions
The Zend framework provides helper classes to unit test the controller. The TestCase is the main component in a PHPUnit framework to write the test cases and the Zend Framework provides an abstract implementation of the TestCase that is called as the AbstractHttpControllerTestCase.
This AbstractHttpControllerTestCase provides various Assert methods and can grouped by functionality. They are as follows −
Request Assertions − Used to assert the http request. For example, assertControllerName.
CSS Select Assertions − Used to check the response HTML using the HTML DOM model.
XPath Assertions − An alternative to the CSS select assertions based on the XPath.
Redirect Assertions − Used to check the page redirection.
Response Header Assertions − Used to check the response header like status code (assertResponseStatusCode)
Create Tests Directory
A unit test can be written separately for each module. All test related coding need to be created inside the test folder under the module's root directory.
For example, to write a test for the TutorialController available under the Tutorial module, the test class needs to be placed under myapp/module/Tutorial/test/Controller/ directory.
Example
Let us write a test class to unit test the TutorialController.
To begin with, we should write a class called TutorialControllerTest and extend it to the AbstractHttpControllerTestCase.
The next step is to write a Setup method to setup the test environment. This can be done by calling the setApplicationConfig method and passing our main application config file myapp/config/application.config.php
public function setUp() { $configOverrides = []; $this->setApplicationConfig(ArrayUtils::merge( include __DIR__ . '/../../../../config/application.config.php', $configOverrides )); parent::setUp(); }
Write one or more methods and call various assert methods depending on the requirement.
$this->assertMatchedRouteName('tutorial');
We have written the test class and the complete listing is as follows −
<?php namespace TutorialTest\Controller; use Tutorial\Controller\TutorialController; use Zend\Stdlib\ArrayUtils; use Zend\Test\PHPUnit\Controller\AbstractHttpControllerTestCase; class TutorialControllerTest extends AbstractHttpControllerTestCase { public function setUp() { $configOverrides = []; $this->setApplicationConfig(ArrayUtils::merge( include __DIR__ . '/../../../../config/application.config.php', $configOverrides )); parent::setUp(); } public function testIndexActionCanBeAccessed() { $this->dispatch('/tutorial', 'GET'); $this->assertResponseStatusCode(200); $this->assertModuleName('tutorial'); $this->assertControllerName(TutorialController::class); $this->assertControllerClass('TutorialController'); $this->assertMatchedRouteName('tutorial'); } }
Now, open a command prompt, move on to application root directory and execute the phpunit executable available inside the vendor folder.
cd /path/to/app ./vendor/bin/phpunit ./vendor/bin/phpunit module/ Tutorial/test/Controller/TutorialControllerTest.php
The result will be as shown in the following code block −
PHPUnit 5.7.5 by Sebastian Bergmann and contributors. .1 / 1 (100%) Time: 96 ms, Memory: 8.00MB OK (1 test, 5 assertions)