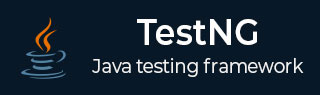
- TestNG Tutorial
- TestNG - Home
- TestNG - Overview
- TestNG - Environment
- TestNG - Writing Tests
- TestNG - Basic Annotations
- TestNG - Execution Procedure
- TestNG - Executing Tests
- TestNG - Suite Test
- TestNG - Ignore a Test
- TestNG - Group Test
- TestNG - Exception Test
- TestNG - Dependency Test
- TestNG - Parameterized Test
- TestNG - Run JUnit Tests
- TestNG - Test Results
- TestNG - Annotation Transformers
- TestNG - Asserts
- TestNG - Parallel Execution
- TestNG - Plug with ANT
- TestNG - Plug with Eclipse
- TestNG - TestNG - vs JUnit
- TestNG Useful Resources
- TestNG - Quick Guide
- TestNG - Useful Resources
- TestNG - Discussion
TestNG - Run JUnit Tests
Now that you have understood TestNG and its various tests, you must be worried by now as to how to refactor your existing JUnit code. There's no need to worry, as TestNG provides a way to shift from JUnit to TestNG at your own pace. You can execute your existing JUnit test cases using TestNG.
TestNG can automatically recognize and run JUnit tests, so that you can use TestNG as a runner for all your existing tests and write new tests using TestNG. All you have to do is to put JUnit library on the TestNG classpath, so it can find and use JUnit classes, change your test runner from JUnit to TestNG in Ant, and then run TestNG in "mixed" mode. This way, you can have all your tests in the same project, even in the same package, and start using TestNG. This approach also allows you to convert your existing JUnit tests to TestNG incrementally.
Let us have an example to demonstrate this amazing ability of TestNG.
Create JUnit Test Case Class
Create a java class, which is a JUnit test class, TestJunit.java in /work/testng/src.
import org.junit.Test; import static org.testng.AssertJUnit.*; public class TestJunit { @Test public void testAdd() { String str = "Junit testing using TestNG"; assertEquals("Junit testing using TestNG",str); } }
Now, let's write the testng.xml in /work/testng/src, which would contain the <suite> tag as follows −
<?xml version = "1.0" encoding = "UTF-8"?> <!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd"> <suite name = "Converted JUnit suite" > <test name = "JUnitTests" junit="true"> <classes> <class name = "TestJunit" /> </classes> </test> </suite>
To execute the JUnit test cases, define the property junit="true" as in the xml above. The JUnit test case class TestJunit is defined in class name.
For JUnit 4, TestNG will use the org.junit.runner.JUnitCore runner to run your tests.
Compile all java classes using javac.
/work/testng/src$ javac TestJunit.java
Now, run testng.xml, which will run the JUnit test case as TestNG.
/work/testng/src$java -cp "/work/testng/src/junit-4.13.2.jar:/work/testng/src/hamcrest-core-1.3.jar" org.testng.TestNG testng.xml
Here, we have placed the dependent JAR files junit-4.13.2.jar and hamcrest-core-1.3.jar under /work/testng/src/.
Verify the output.
=============================================== Converted JUnit suite Total tests run: 1, Passes: 1, Failures: 0, Skips: 0 ===============================================