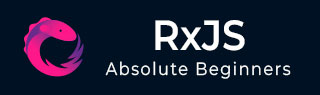
- RxJS Tutorial
- RxJS - Home
- RxJS - Overview
- RxJS - Environment Setup
- RxJS - Latest Updates
- RxJS - Observables
- RxJS - Operators
- RxJS - Working with Subscription
- RxJS - Working with Subjects
- RxJS - Working with Scheduler
- RxJS - Working with RxJS & Angular
- RxJS - Working with RxJS & ReactJS
- RxJS Useful Resources
- RxJS - Quick Guide
- RxJS - Useful Resources
- RxJS - Discussion
Working with RxJS & ReactJS
In this chapter, we will see how to use RxJs with ReactJS. We will not get into the installation process for Reactjs here, to know about ReactJS Installation refer this link: /reactjs/reactjs_environment_setup.htm
Example
We will directly work on an example below, where will use Ajax from RxJS to load data.
index.js
import React, { Component } from "react"; import ReactDOM from "react-dom"; import { ajax } from 'rxjs/ajax'; import { map } from 'rxjs/operators'; class App extends Component { constructor() { super(); this.state = { data: [] }; } componentDidMount() { const response = ajax('https://jsonplaceholder.typicode.com/users').pipe(map(e => e.response)); response.subscribe(res => { this.setState({ data: res }); }); } render() { return ( <div> <h3>Using RxJS with ReactJS</h3> <ul> {this.state.data.map(el => ( <li> {el.id}: {el.name} </li> ))} </ul> </div> ); } } ReactDOM.render(<App />, document.getElementById("root"));
index.html
<!DOCTYPE html> <html> <head> <meta charset = "UTF-8" /> <title>ReactJS Demo</title> <head> <body> <div id = "root"></div> </body> </html>
We have used ajax from RxJS that will load data from this Url − https://jsonplaceholder.typicode.com/users.
When you compile, the display is as shown below −

Advertisements