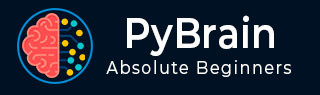
- PyBrain Tutorial
- PyBrain - Home
- PyBrain - Overview
- PyBrain - Environment Setup
- PyBrain - Introduction to PyBrain Networks
- PyBrain - Working with Networks
- PyBrain - Working with Datasets
- PyBrain - Datasets Types
- PyBrain - Importing Data For Datasets
- PyBrain - Training Datasets on Networks
- PyBrain - Testing Network
- Working with Feed-Forward Networks
- PyBrain - Working with Recurrent Networks
- Training Network Using Optimization Algorithms
- PyBrain - Layers
- PyBrain - Connections
- PyBrain - Reinforcement Learning Module
- PyBrain - API & Tools
- PyBrain - Examples
- PyBrain Useful Resources
- PyBrain - Quick Guide
- PyBrain - Useful Resources
- PyBrain - Discussion
PyBrain - Connections
A connection works similar to a layer; an only difference is that it shifts the data from one node to the other in a network.
In this chapter, we are going to learn about −
- Understanding Connections
- Creating Connections
Understanding Connections
Here is a working example of connections used while creating a network.
Example
ffy.py
from pybrain.structure import FeedForwardNetwork from pybrain.structure import LinearLayer, SigmoidLayer from pybrain.structure import FullConnection network = FeedForwardNetwork() #creating layer for input => 2 , hidden=> 3 and output=>1 inputLayer = LinearLayer(2) hiddenLayer = SigmoidLayer(3) outputLayer = LinearLayer(1) #adding the layer to feedforward network network.addInputModule(inputLayer) network.addModule(hiddenLayer) network.addOutputModule(outputLayer) #Create connection between input ,hidden and output input_to_hidden = FullConnection(inputLayer, hiddenLayer) hidden_to_output = FullConnection(hiddenLayer, outputLayer) #add connection to the network network.addConnection(input_to_hidden) network.addConnection(hidden_to_output) network.sortModules() print(network)
Output
C:\pybrain\pybrain\src>python ffn.py FeedForwardNetwork-6 Modules: [<LinearLayer 'LinearLayer-3'>, <SigmoidLayer 'SigmoidLayer-7'>, <LinearLayer 'LinearLayer-8'>] Connections: [<FullConnection 'FullConnection-4': 'SigmoidLayer-7' -> 'LinearLayer-8'>, <FullConnection 'FullConnection-5': 'LinearLayer-3' -> 'SigmoidLayer-7'>]
Creating Connections
In Pybrain, we can create connections by using the connection module as shown below −
Example
connect.py
from pybrain.structure.connections.connection import Connection class YourConnection(Connection): def __init__(self, *args, **kwargs): Connection.__init__(self, *args, **kwargs) def _forwardImplementation(self, inbuf, outbuf): outbuf += inbuf def _backwardImplementation(self, outerr, inerr, inbuf): inerr += outer
To create a connection, there are 2 methods — _forwardImplementation() and _backwardImplementation().
The _forwardImplementation() is called with the output buffer of the incoming module which is inbuf, and the input buffer of the outgoing module called outbuf. The inbuf is added to the outgoing module outbuf.
The _backwardImplementation() is called with outerr, inerr, and inbuf. The outgoing module error is added to the incoming module error in _backwardImplementation().
Let us now use the YourConnection in a network.
testconnection.py
from pybrain.structure import FeedForwardNetwork from pybrain.structure import LinearLayer, SigmoidLayer from connect import YourConnection network = FeedForwardNetwork() #creating layer for input => 2 , hidden=> 3 and output=>1 inputLayer = LinearLayer(2) hiddenLayer = SigmoidLayer(3) outputLayer = LinearLayer(1) #adding the layer to feedforward network network.addInputModule(inputLayer) network.addModule(hiddenLayer) network.addOutputModule(outputLayer) #Create connection between input ,hidden and output input_to_hidden = YourConnection(inputLayer, hiddenLayer) hidden_to_output = YourConnection(hiddenLayer, outputLayer) #add connection to the network network.addConnection(input_to_hidden) network.addConnection(hidden_to_output) network.sortModules() print(network)
Output
C:\pybrain\pybrain\src>python testconnection.py FeedForwardNetwork-6 Modules: [<LinearLayer 'LinearLayer-3'>, <SigmoidLayer 'SigmoidLayer-7'>, <LinearLayer 'LinearLayer-8'>] Connections: [<YourConnection 'YourConnection-4': 'LinearLayer-3' -> 'SigmoidLayer-7'>, <YourConnection 'YourConnection-5': 'SigmoidLayer-7' -> 'LinearLayer-8'>]