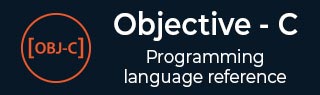
- Basic Objective-C
- Objective-C - Home
- Objective-C - Overview
- Objective-C - Environment Setup
- Objective-C - Program Structure
- Objective-C - Basic Syntax
- Objective-C - Data Types
- Objective-C - Variables
- Objective-C - Constants
- Objective-C - Operators
- Objective-C - Loops
- Objective-C - Decision Making
- Objective-C - Functions
- Objective-C - Blocks
- Objective-C - Numbers
- Objective-C - Arrays
- Objective-C - Pointers
- Objective-C - Strings
- Objective-C - Structures
- Objective-C - Preprocessors
- Objective-C - Typedef
- Objective-C - Type Casting
- Objective-C - Log Handling
- Objective-C - Error Handling
- Command-Line Arguments
- Advanced Objective-C
- Objective-C - Classes & Objects
- Objective-C - Inheritance
- Objective-C - Polymorphism
- Objective-C - Data Encapsulation
- Objective-C - Categories
- Objective-C - Posing
- Objective-C - Extensions
- Objective-C - Protocols
- Objective-C - Dynamic Binding
- Objective-C - Composite Objects
- Obj-C - Foundation Framework
- Objective-C - Fast Enumeration
- Obj-C - Memory Management
- Objective-C Useful Resources
- Objective-C - Quick Guide
- Objective-C - Useful Resources
- Objective-C - Discussion
Objective-C Program Structure
Before we study basic building blocks of the Objective-C programming language, let us look a bare minimum Objective-C program structure so that we can take it as a reference in upcoming chapters.
Objective-C Hello World Example
A Objective-C program basically consists of the following parts −
- Preprocessor Commands
- Interface
- Implementation
- Method
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" −
#import <Foundation/Foundation.h> @interface SampleClass:NSObject - (void)sampleMethod; @end @implementation SampleClass - (void)sampleMethod { NSLog(@"Hello, World! \n"); } @end int main() { /* my first program in Objective-C */ SampleClass *sampleClass = [[SampleClass alloc]init]; [sampleClass sampleMethod]; return 0; }
Let us look various parts of the above program −
The first line of the program #import <Foundation/Foundation.h> is a preprocessor command, which tells a Objective-C compiler to include Foundation.h file before going to actual compilation.
The next line @interface SampleClass:NSObject shows how to create an interface. It inherits NSObject, which is the base class of all objects.
The next line - (void)sampleMethod; shows how to declare a method.
The next line @end marks the end of an interface.
The next line @implementation SampleClass shows how to implement the interface SampleClass.
The next line - (void)sampleMethod{} shows the implementation of the sampleMethod.
The next line @end marks the end of an implementation.
The next line int main() is the main function where program execution begins.
The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program.
The next line NSLog(...) is another function available in Objective-C which causes the message "Hello, World!" to be displayed on the screen.
The next line return 0; terminates main()function and returns the value 0.
Compile & Execute Objective-C Program
Now when we compile and run the program, we will get the following result.
2017-10-06 07:48:32.020 demo[65832] Hello, World!