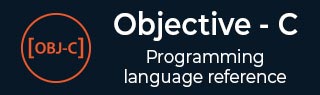
- Basic Objective-C
- Objective-C - Home
- Objective-C - Overview
- Objective-C - Environment Setup
- Objective-C - Program Structure
- Objective-C - Basic Syntax
- Objective-C - Data Types
- Objective-C - Variables
- Objective-C - Constants
- Objective-C - Operators
- Objective-C - Loops
- Objective-C - Decision Making
- Objective-C - Functions
- Objective-C - Blocks
- Objective-C - Numbers
- Objective-C - Arrays
- Objective-C - Pointers
- Objective-C - Strings
- Objective-C - Structures
- Objective-C - Preprocessors
- Objective-C - Typedef
- Objective-C - Type Casting
- Objective-C - Log Handling
- Objective-C - Error Handling
- Command-Line Arguments
- Advanced Objective-C
- Objective-C - Classes & Objects
- Objective-C - Inheritance
- Objective-C - Polymorphism
- Objective-C - Data Encapsulation
- Objective-C - Categories
- Objective-C - Posing
- Objective-C - Extensions
- Objective-C - Protocols
- Objective-C - Dynamic Binding
- Objective-C - Composite Objects
- Obj-C - Foundation Framework
- Objective-C - Fast Enumeration
- Obj-C - Memory Management
- Objective-C Useful Resources
- Objective-C - Quick Guide
- Objective-C - Useful Resources
- Objective-C - Discussion
Objective-C Fast Enumeration
Fast enumeration is an Objective-C's feature that helps in enumerating through a collection. So in order to know about fast enumeration, we need know about collection first which will be explained in the following section.
Collections in Objective-C
Collections are fundamental constructs. It is used to hold and manage other objects. The whole purpose of a collection is that it provides a common way to store and retrieve objects efficiently.
There are several different types of collections. While they all fulfill the same purpose of being able to hold other objects, they differ mostly in the way objects are retrieved. The most common collections used in Objective-C are −
- NSSet
- NSArray
- NSDictionary
- NSMutableSet
- NSMutableArray
- NSMutableDictionary
If you want to know more about these structures, please refer data storage in Foundation Framework.
Fast enumeration Syntax
for (classType variable in collectionObject ) { statements }
Here is an example for fast enumeration.
#import <Foundation/Foundation.h> int main() { NSAutoreleasePool * pool = [[NSAutoreleasePool alloc] init]; NSArray *array = [[NSArray alloc] initWithObjects:@"string1", @"string2",@"string3",nil]; for(NSString *aString in array) { NSLog(@"Value: %@",aString); } [pool drain]; return 0; }
Now when we compile and run the program, we will get the following result.
2013-09-28 06:26:22.835 demo[7426] Value: string1 2013-09-28 06:26:22.836 demo[7426] Value: string2 2013-09-28 06:26:22.836 demo[7426] Value: string3
As you can see in the output, each of the objects in the array is printed in an order.
Fast Enumeration Backwards
for (classType variable in [collectionObject reverseObjectEnumerator] ) { statements }
Here is an example for reverseObjectEnumerator in fast enumeration.
#import <Foundation/Foundation.h> int main() { NSAutoreleasePool * pool = [[NSAutoreleasePool alloc] init]; NSArray *array = [[NSArray alloc] initWithObjects:@"string1", @"string2",@"string3",nil]; for(NSString *aString in [array reverseObjectEnumerator]) { NSLog(@"Value: %@",aString); } [pool drain]; return 0; }
Now when we compile and run the program, we will get the following result.
2013-09-28 06:27:51.025 demo[12742] Value: string3 2013-09-28 06:27:51.025 demo[12742] Value: string2 2013-09-28 06:27:51.025 demo[12742] Value: string1
As you can see in the output, each of the objects in the array is printed but in the reverse order as compared to normal fast enumeration.