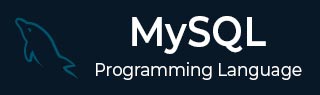
- MySQL Basics
- MySQL - Home
- MySQL - Introduction
- MySQL - Features
- MySQL - Versions
- MySQL - Variables
- MySQL - Installation
- MySQL - Administration
- MySQL - PHP Syntax
- MySQL - Node.js Syntax
- MySQL - Java Syntax
- MySQL - Python Syntax
- MySQL - Connection
- MySQL - Workbench
- MySQL Databases
- MySQL - Create Database
- MySQL - Drop Database
- MySQL - Select Database
- MySQL - Show Database
- MySQL - Copy Database
- MySQL - Database Export
- MySQL - Database Import
- MySQL - Database Info
- MySQL Users
- MySQL - Create Users
- MySQL - Drop Users
- MySQL - Show Users
- MySQL - Change Password
- MySQL - Grant Privileges
- MySQL - Show Privileges
- MySQL - Revoke Privileges
- MySQL - Lock User Account
- MySQL - Unlock User Account
- MySQL Tables
- MySQL - Create Tables
- MySQL - Show Tables
- MySQL - Alter Tables
- MySQL - Rename Tables
- MySQL - Clone Tables
- MySQL - Truncate Tables
- MySQL - Temporary Tables
- MySQL - Repair Tables
- MySQL - Describe Tables
- MySQL - Add/Delete Columns
- MySQL - Show Columns
- MySQL - Rename Columns
- MySQL - Table Locking
- MySQL - Drop Tables
- MySQL - Derived Tables
- MySQL Queries
- MySQL - Queries
- MySQL - Constraints
- MySQL - Insert Query
- MySQL - Select Query
- MySQL - Update Query
- MySQL - Delete Query
- MySQL - Replace Query
- MySQL - Insert Ignore
- MySQL - Insert on Duplicate Key Update
- MySQL - Insert Into Select
- MySQL Indexes
- MySQL - Indexes
- MySQL - Create Index
- MySQL - Drop Index
- MySQL - Show Indexes
- MySQL - Unique Index
- MySQL - Clustered Index
- MySQL - Non-Clustered Index
- MySQL Operators and Clauses
- MySQL - Where Clause
- MySQL - Limit Clause
- MySQL - Distinct Clause
- MySQL - Order By Clause
- MySQL - Group By Clause
- MySQL - Having Clause
- MySQL - AND Operator
- MySQL - OR Operator
- MySQL - Like Operator
- MySQL - IN Operator
- MySQL - ANY Operator
- MySQL - EXISTS Operator
- MySQL - NOT Operator
- MySQL - NOT EQUAL Operator
- MySQL - IS NULL Operator
- MySQL - IS NOT NULL Operator
- MySQL - Between Operator
- MySQL - UNION Operator
- MySQL - UNION vs UNION ALL
- MySQL - MINUS Operator
- MySQL - INTERSECT Operator
- MySQL - INTERVAL Operator
- MySQL Joins
- MySQL - Using Joins
- MySQL - Inner Join
- MySQL - Left Join
- MySQL - Right Join
- MySQL - Cross Join
- MySQL - Full Join
- MySQL - Self Join
- MySQL - Delete Join
- MySQL - Update Join
- MySQL - Union vs Join
- MySQL Keys
- MySQL - Unique Key
- MySQL - Primary Key
- MySQL - Foreign Key
- MySQL - Composite Key
- MySQL - Alternate Key
- MySQL Triggers
- MySQL - Triggers
- MySQL - Create Trigger
- MySQL - Show Trigger
- MySQL - Drop Trigger
- MySQL - Before Insert Trigger
- MySQL - After Insert Trigger
- MySQL - Before Update Trigger
- MySQL - After Update Trigger
- MySQL - Before Delete Trigger
- MySQL - After Delete Trigger
- MySQL Data Types
- MySQL - Data Types
- MySQL - VARCHAR
- MySQL - BOOLEAN
- MySQL - ENUM
- MySQL - DECIMAL
- MySQL - INT
- MySQL - FLOAT
- MySQL - BIT
- MySQL - TINYINT
- MySQL - BLOB
- MySQL - SET
- MySQL Regular Expressions
- MySQL - Regular Expressions
- MySQL - RLIKE Operator
- MySQL - NOT LIKE Operator
- MySQL - NOT REGEXP Operator
- MySQL - regexp_instr() Function
- MySQL - regexp_like() Function
- MySQL - regexp_replace() Function
- MySQL - regexp_substr() Function
- MySQL Fulltext Search
- MySQL - Fulltext Search
- MySQL - Natural Language Fulltext Search
- MySQL - Boolean Fulltext Search
- MySQL - Query Expansion Fulltext Search
- MySQL - ngram Fulltext Parser
- MySQL Functions & Operators
- MySQL - Date and Time Functions
- MySQL - Arithmetic Operators
- MySQL - Numeric Functions
- MySQL - String Functions
- MySQL - Aggregate Functions
- MySQL Misc Concepts
- MySQL - NULL Values
- MySQL - Transactions
- MySQL - Using Sequences
- MySQL - Handling Duplicates
- MySQL - SQL Injection
- MySQL - SubQuery
- MySQL - Comments
- MySQL - Check Constraints
- MySQL - Storage Engines
- MySQL - Export Table into CSV File
- MySQL - Import CSV File into Database
- MySQL - UUID
- MySQL - Common Table Expressions
- MySQL - On Delete Cascade
- MySQL - Upsert
- MySQL - Horizontal Partitioning
- MySQL - Vertical Partitioning
- MySQL - Cursor
- MySQL - Stored Functions
- MySQL - Signal
- MySQL - Resignal
- MySQL - Character Set
- MySQL - Collation
- MySQL - Wildcards
- MySQL - Alias
- MySQL - ROLLUP
- MySQL - Today Date
- MySQL - Literals
- MySQL - Stored Procedure
- MySQL - Explain
- MySQL - JSON
- MySQL - Standard Deviation
- MySQL - Find Duplicate Records
- MySQL - Delete Duplicate Records
- MySQL - Select Random Records
- MySQL - Show Processlist
- MySQL - Change Column Type
- MySQL - Reset Auto-Increment
- MySQL - Coalesce() Function
MySQL - TINYINT
The MySQL TINYINT Data Type
The MySQL TINYINT data type is used to store integer values within a very small range. It occupies just 1 byte (8 bits) of storage and can hold values from -128 to 127 for signed TINYINT or 0 to 255 for unsigned TINYINT.
When you define a TINYINT column in MySQL, by default it is considered as SIGNED. This means it can hold both positive and negative numbers within a specific range. Additionally, you can use either "TINYINT" or "INT1" to define such a column because they work the same way.
Syntax
Following is the syntax of the MySQL TINYINT data type −
TINYINT(M) [SIGNED | UNSIGNED | ZEROFILL]
Example
First, let us create a table with the name tinyint_table using the below query −
CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL );
Following is the output obtained −
Query OK, 0 rows affected, 1 warning (0.03 sec)
Now, let us try to insert some values (128, 128, 128) into these columns as shown below −
INSERT INTO tinyint_table VALUES (128, 128, 128);
An error is generated for the value in col1 because the value we inserted is out of range −
ERROR 1264 (22003): Out of range value for column 'col1' at row 1
Next, if we try to insert a negative value into the TINYINT UNSIGNED column ("col2"), it will result in an error because UNSIGNED values cannot be negative −
INSERT INTO tinyint_table VALUES (127, -120, 128);
The error message displayed is as follows −
ERROR 1264 (22003): Out of range value for column 'col2' at row 1
Similarly, if we insert -128 into the TINYINT ZEROFILL column ("col3"), an error will be generated −
INSERT INTO tinyint_table VALUES (127, 128, -128);
The output is as shown below −
ERROR 1264 (22003): Out of range value for column 'col3' at row 1
However, if we insert values within the valid range, the insertion will succeed as shown below −
INSERT INTO tinyint_table VALUES (127, 128, 128);
Following is the output of the above code −
Query OK, 1 row affected (0.01 sec)
Finally, we can retrieve all the records present in the table using the following SELECT query −
SELECT * FROM tinyint_table;
This query will display the following result −
col1 | col2 | col3 |
---|---|---|
127 | 128 | 128 |
TINYINT Datatype Using a Client Program
We can also create column of the TINYINT datatype using the client program.
Syntax
To create a column of TINYINT datatype through a PHP program, we need to execute the "CREATE TABLE" statement using the mysqli function query() as follows −
$sql = 'CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )'; $mysqli->query($sql);
To create a column of TINYINT datatype through a JavaScript program, we need to execute the "CREATE TABLE" statement using the query() function of mysql2 library as follows −
sql = "CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )"; con.query(sql);
To create a column of TINYINT datatype through a Java program, we need to execute the "CREATE TABLE" statement using the JDBC function execute() as follows −
String sql = "CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )"; statement.execute(sql);
To create a column of TINYINT datatype through a python program, we need to execute the "CREATE TABLE" statement using the execute() function of the MySQL Connector/Python as follows −
sql = 'CREATE TABLE tinyint_table (col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL)' cursorObj.execute(sql)
Example
Following are the programs −
$dbhost = 'localhost'; $dbuser = 'root'; $dbpass = 'password'; $dbname = 'TUTORIALS'; $mysqli = new mysqli($dbhost, $dbuser, $dbpass, $dbname); if ($mysqli->connect_errno) { printf("Connect failed: %s
", $mysqli->connect_error); exit(); } //printf('Connected successfully.
'); $sql = 'CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )'; $result = $mysqli->query($sql); if ($result) { printf("Table created successfully...!\n"); } // insert data into created table $q = " INSERT INTO tinyint_table (col1, col2, col3) VALUES (100, 105, 110)"; if ($res = $mysqli->query($q)) { printf("Data inserted successfully...!\n"); } //now display the table records $s = "SELECT * FROM tinyint_table"; if ($r = $mysqli->query($s)) { printf("Table Records: \n"); while ($row = $r->fetch_assoc()) { printf(" Col_1: %s, Col_2: %s, Col_3: %s", $row["col1"], $row["col2"], $row["col3"]); printf("\n"); } } else { printf('Failed'); } $mysqli->close();
Output
The output obtained is as follows −
Table created successfully...! Data inserted successfully...! Table Records: Col_1: 100, Col_2: 105, Col_3: 110
var mysql = require("mysql2"); var con = mysql.createConnection({ host: "localhost", user: "root", password: "password", }); //Connecting to MySQL con.connect(function (err) { if (err) throw err; // console.log("Connected successfully...!"); // console.log("--------------------------"); sql = "USE TUTORIALS"; con.query(sql); //create a tinyint_table table, that accepts one column of tinyint type. sql = "CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )"; con.query(sql); //insert data into created table sql = "INSERT INTO tinyint_table (col1, col2, col3) VALUES (100, 105, 110)"; con.query(sql); //select datatypes of salary sql = `SELECT DATA_TYPE FROM INFORMATION_SCHEMA.COLUMNS WHERE table_name = 'tinyint_table' AND COLUMN_NAME = 'col2'`; con.query(sql, function (err, result) { if (err) throw err; console.log(result); }); });
Output
The output produced is as follows −
[ { DATA_TYPE: 'tinyint' } ]
import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.Statement; public class TinyInt { public static void main(String[] args) { String url = "jdbc:mysql://localhost:3306/TUTORIALS"; String username = "root"; String password = "password"; try { Class.forName("com.mysql.cj.jdbc.Driver"); Connection connection = DriverManager.getConnection(url, username, password); Statement statement = connection.createStatement(); System.out.println("Connected successfully...!"); //TinyInt data types...!; String sql = "CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )"; statement.execute(sql); System.out.println("column of a TINYINT type created successfully...!"); ResultSet resultSet = statement.executeQuery("DESCRIBE tinyint_table"); while (resultSet.next()){ System.out.println(resultSet.getString(1)+" "+resultSet.getString(2)); } connection.close(); } catch (Exception e) { System.out.println(e); } } }
Output
The output obtained is as shown below −
Connected successfully...! column of a TINYINT type created successfully...! col1 tinyint col2 tinyint unsigned col3 tinyint(3) unsigned zerofill
import mysql.connector # Establishing the connection connection = mysql.connector.connect( host='localhost', user='root', password='password', database='tut' ) # Creating a cursor object cursorObj = connection.cursor() # Create table with Tinyint column sql = ''' CREATE TABLE tinyint_table ( col1 TINYINT, col2 TINYINT UNSIGNED, col3 TINYINT ZEROFILL )''' cursorObj.execute(sql) print("The table is created successfully!") # Insert data into the created table insert_query = "INSERT INTO tinyint_table (col1, col2, col3) VALUES (127, 128, 128);" cursorObj.execute(insert_query) # Commit the changes after the insert operation connection.commit() print("Rows inserted successfully.") # Now display the table records select_query = "SELECT * FROM tinyint_table" cursorObj.execute(select_query) result = cursorObj.fetchall() print("Table Data:") for row in result: print(row) cursorObj.close() connection.close()
Output
Following is the output of the above code −
The table is created successfully! Rows inserted successfully. Table Data: (127, 128, 128)